Apple Pay
Allow customers to securely make payments using Apple Pay on their iPhone, iPad, or Apple Watch.
Apple Pay is compatible with most Stripe products and features. Stripe users can accept Apple Pay in iOS applications in iOS 9 and above, and on the web in Safari starting with iOS 10 or macOS Sierra. There are no additional fees to process Apple Pay payments, and pricing is the same as for other card transactions.
Apple Pay is available to cardholders at participating banks in supported countries. For more information, refer to Apple’s participating banks documentation.
Payment flow
Below is a demonstration of the Apple Pay payment flow from your checkout page:
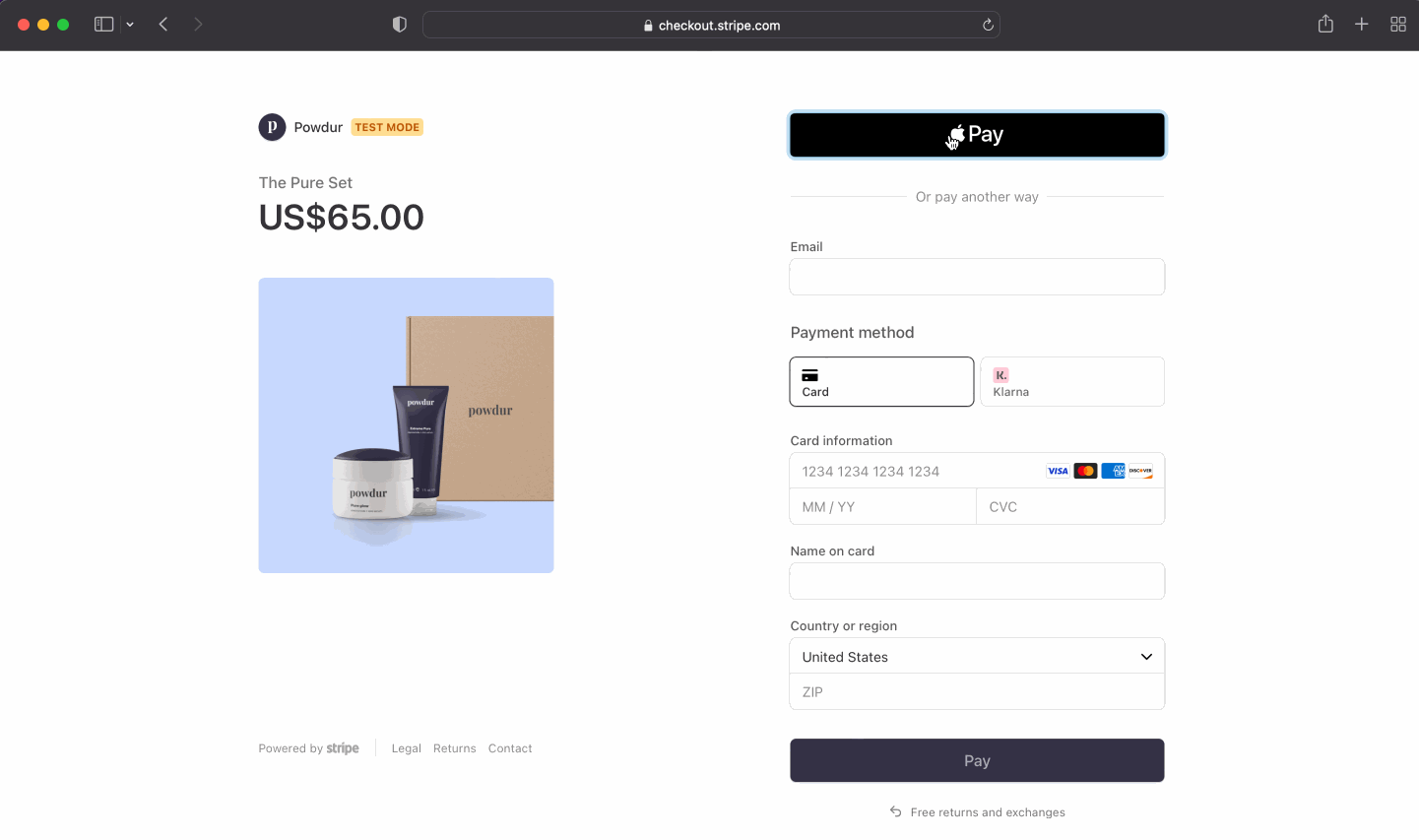
Using Stripe and Apple Pay versus in-app purchases
For sales of physical goods, services, and certain other things, your app can accept Apple Pay or any other Stripe-supported payment method. Those payments are processed through Stripe, and you only need to pay Stripe’s processing fees. However, sales of digital products, content, and certain other things must use Apple’s in-app purchases. Those payments are processed by Apple and are subject to their transaction fees.
For more information about which sales must use in-app purchases, see the Apple App Store Review Guidelines.
Accept Apple Pay
Stripe offers a variety of methods to add Apple Pay as a payment method. For integration details, select the method you prefer:
You can use the Stripe React Native SDK, to accept both Apple Pay and traditional credit card payments. Before starting, you need to enrol in the Apple Developer Program and set up Stripe on your server and in your app. Next, follow these steps:
- Register for an Apple Merchant ID
- Create a new Apple Pay certificate
- Integrate with Xcode
- Set your Apple Merchant ID in StripeProvider
- Check if Apple Pay is supported
- Present the payment sheet
- Submit the payment to Stripe
Register for an Apple Merchant ID
Obtain an Apple Merchant ID by registering for a new identifier on the Apple Developer website.
Fill out the form with a description and identifier. Your description is for your own records and you can modify it in the future. Stripe recommends using the name of your app as the identifier (for example, merchant.
).
Create a new Apple Pay certificate
Create a certificate for your app to encrypt payment data.
Go to the iOS Certificate Settings in the Dashboard, click Add new application, and follow the guide.
Download a Certificate Signing Request (CSR) file to get a secure certificate from Apple that allows you to use Apple Pay.
One CSR file must be used to issue exactly one certificate. If you switch your Apple Merchant ID, you must go to the iOS Certificate Settings in the Dashboard to obtain a new CSR and certificate.
Integrate with Xcode
Add the Apple Pay capability to your app. In Xcode, open your project settings, click the Signing & Capabilities tab, and add the Apple Pay capability. You might be prompted to log in to your developer account at this point. Select the merchant ID you created earlier, and your app is ready to accept Apple Pay.
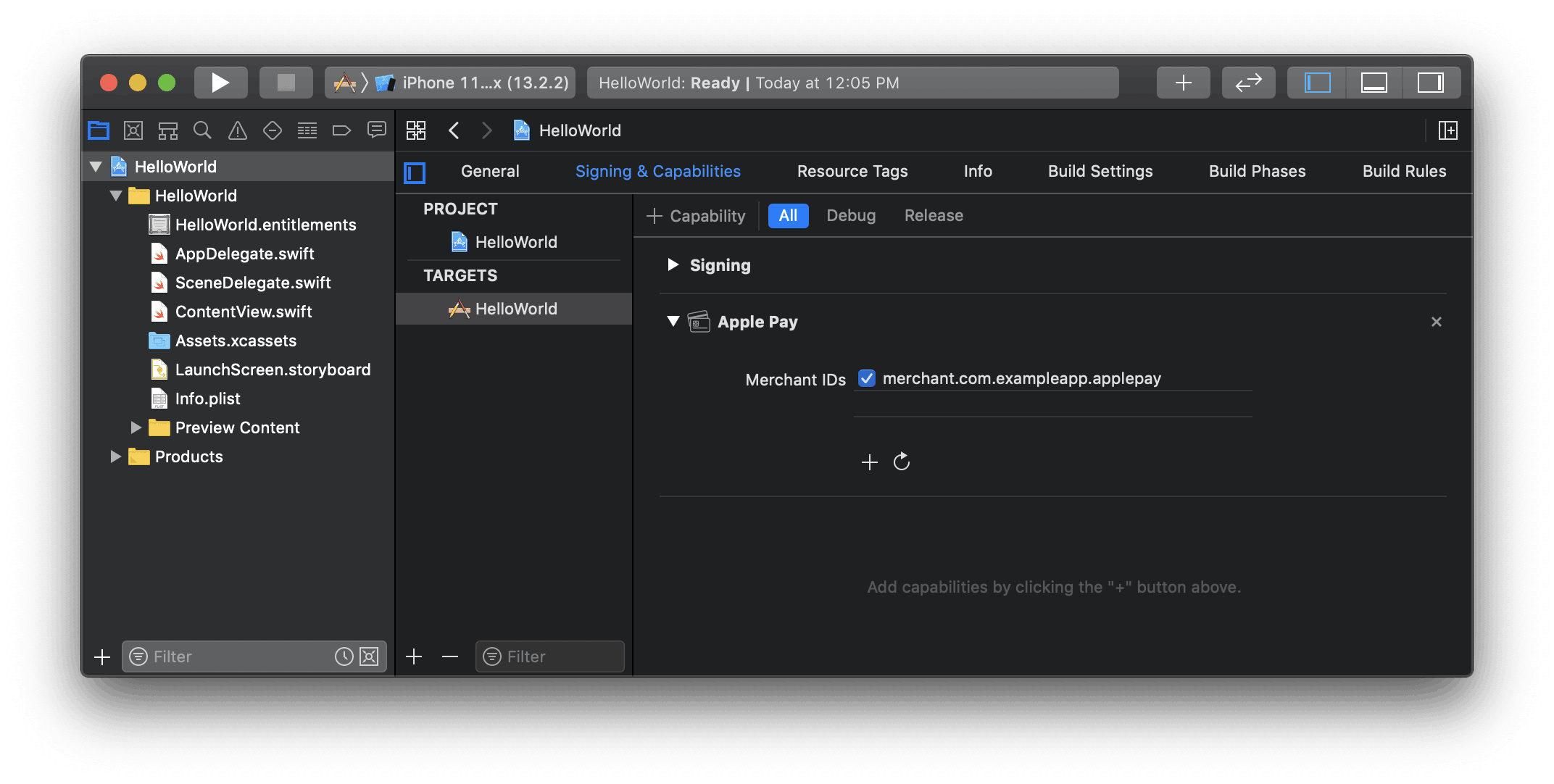
Enable the Apple Pay capability in Xcode
Set your Apple Merchant ID in StripeProvider
In the StripeProvider
component, specify the Apple Merchant ID that you successfully registered for:
import { StripeProvider } from '@stripe/stripe-react-native'; function App() { return ( <StripeProvider publishableKey="
" merchantIdentifier="merchant.com.{{YOUR_APP_NAME}}" > // Your app code here </StripeProvider> ); }pk_test_TYooMQauvdEDq54NiTphI7jx
Check if Apple Pay is supported
Before displaying Apple Pay as a payment option in your app, determine if the user’s device supports Apple Pay and that they have a card added to their wallet:
import { PlatformPayButton, isPlatformPaySupported } from '@stripe/stripe-react-native'; function PaymentScreen() { const [isApplePaySupported, setIsApplePaySupported] = useState(false); useEffect(() => { (async function () { setIsApplePaySupported(await isPlatformPaySupported()); })(); }, [isPlatformPaySupported]); // ... const pay = async () => { // ... }; // ... return ( <View> {isApplePaySupported && ( <PlatformPayButton onPress={pay} type={PlatformPay.ButtonType.Order} appearance={PlatformPay.ButtonStyle.Black} borderRadius={4} style={{ width: '100%', height: 50, }} /> )} </View> ); }
Create the Payment Intent
Server-side
Make an endpoint that creates a PaymentIntent with an amount and currency. Always decide how much to charge on the server side, a trusted environment, as opposed to the client side. This prevents malicious customers from choosing their own prices.
Client-side
Create a method that requests a PaymentIntent from your server:
function PaymentScreen() { // ... const fetchPaymentIntentClientSecret = async () => { const response = await fetch(`${API_URL}/create-payment-intent`, { method: 'POST', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify({ some: 'value', }), }); const { clientSecret } = await response.json(); return clientSecret; }; // ... }
Troubleshooting
If you’re seeing errors from the Stripe API when attempting to create tokens, you most likely have a problem with your Apple Pay Certificate. You’ll need to generate a new certificate and upload it to Stripe, as described on this page. Make sure you use a CSR obtained from your Dashboard and not one you generated yourself. Xcode often incorrectly caches old certificates, so in addition to generating a new certificate, Stripe recommends creating a new Apple Merchant ID as well.
If you receive the error:
You haven’t added your Apple merchant account to Stripe
it’s likely your app is sending data encrypted with a previous (non-Stripe) CSR/Certificate. Make sure any certificates generated by non-Stripe CSRs are revoked under your Apple Merchant ID. If this doesn’t resolve the issue, delete the merchant ID in your Apple account and re-create it. Then, create a new certificate based on the same (Stripe-provided) CSR that was previously used. You don’t need to upload this new certificate to Stripe. When finished, toggle the Apple Pay Credentials off and on in your app to ensure they refresh properly.
Present the payment sheet
In your PlatformPayButton
’s onPress
prop, call confirmPlatformPayPayment
to open an Apple Pay sheet. To display the customer’s cart items on the payment sheet, pass the items as an argument.
Note
In your code that handles the customer action, don’t include any complex or asynchronous actions before displaying the payment sheet. If the user action doesn’t directly invoke the payment sheet, Apple Pay returns an error.
import { confirmPlatformPayPayment } from '@stripe/stripe-react-native'; function PaymentScreen() { // ... see above const pay = async () => { const clientSecret = await fetchPaymentIntentClientSecret() const { error } = await confirmPlatformPayPayment( clientSecret, { applePay: { cartItems: [ { label: 'Example item name', amount: '14.00', paymentType: PlatformPay.PaymentType.Immediate, }, { label: 'Total', amount: '12.75', paymentType: PlatformPay.PaymentType.Immediate, }, ], merchantCountryCode: 'US', currencyCode: 'USD', requiredShippingAddressFields: [ PlatformPay.ContactField.PostalAddress, ], requiredBillingContactFields: [PlatformPay.ContactField.PhoneNumber], }, } ); if (error) { // handle error } else { Alert.alert('Success', 'Check the logs for payment intent details.'); console.log(JSON.stringify(paymentIntent, null, 2)); } }; // ... see above }
Testing Apple Pay
Stripe test card information can’t be saved to Wallet in iOS. Instead, Stripe recognises when you’re using your test API keys and returns a successful test card token for you to use. This allows you to make test payments using a live card without it being charged.