SettingsView
アカウントでのアプリの機能に関して、顧客が詳細を変更できるようにします。
専用の設定ビューを定義すると、ユーザーが各自のアカウントでアプリがどのように機能するかの詳細を変更することができます。たとえば、Zendesk などのサードパーティー API を使用するアプリでは、SettingsView
を使用してユーザーを Zendesk アカウントで認証できます。詳細については、アプリの設定ページを追加する方法をご覧ください。
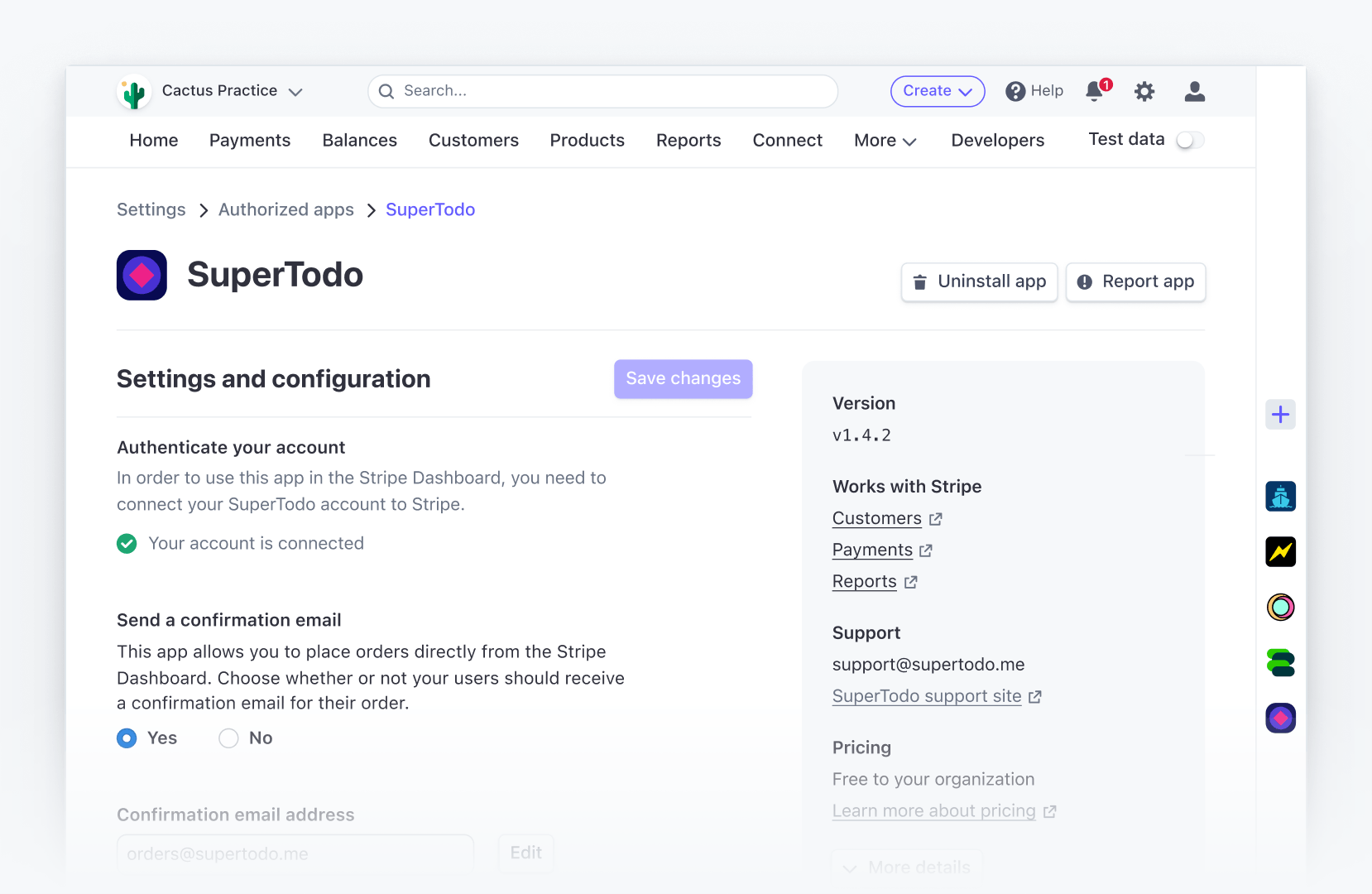
SettingsView のデザイン
SettingsView
は、ContextView
と同様に、その他すべての UI エレメントを含むビューのルートコンポーネントです。特定のオブジェクトに関連付けられていない唯一のビューですが、settings
ビューポートに関連付けられています。settings
ビューポートは、アプリドロワー以外の、ダッシュボードの事前設定された場所にマッピングされます。
アプリをアップロードすると、SettingsView
がダッシュボードのアプリの設定ページに表示されます。アプリをローカルでプレビューしてる間は、ダッシュボードの https://dashboard.stripe.com/test/apps/settings-preview で SettingsView
をプレビューできます。
SettingsView
を使用するには、settings
ビューポートでアプリマニフェストにビューを追加する必要があります。SettingsView があるアプリケーションには、以下のような ui_extension
フィールドを含むアプリマニフェストがあります。
{ ..., "ui_extension": { "views": [ ..., { "viewport": "settings", "component": "AppSettings" } ], } }
Props
Prop | Type | Description |
---|---|---|
children Required |
| The contents of the SettingsView, usually a Form or some
other content surrounding a form. |
onSave |
| If provided, a "Save" button will be rendered with the SettingsView.
This callback will be called when the button is clicked. |
statusMessage |
| A string to display a status such as "Saved" or "Error"
in the footer of the view. |
例
この例は、外部 API から設定を取得して表示し、変更を保存する方法を示しています。
import {useState, useEffect, useCallback} from 'react'; import { Box, TextField, SettingsView, } from '@stripe/ui-extension-sdk/ui'; type FormStatus = 'initial' | 'saving' | 'saved' | 'error'; const AppSettings = ({ userContext }: any) => { const [storedValue, setStoredValue] = useState<string>(''); const [status, setStatus] = useState<FormStatus>('initial'); // use the current user id to retrieve the stored value from an external api const key = userContext.id; useEffect(() => { const fetchSetting = async (key: number) => { try { const response = await fetch(`https://www.my-api.com/${key}`) const storedSettingValue = await response.text() if (storedSettingValue) { setStoredValue(storedSettingValue) } } catch (error) { console.log('Error fetching setting: ', error) } }; fetchSetting(key); }, [key]); const saveSettings = useCallback(async (values) => { setStatus('saving'); try { const { greeting } = values; const result = await fetch( 'https://www.my-api.com/', { method: 'POST', body: JSON.stringify(values) } ); await result.text(); setStatus('saved'); setStoredValue(greeting); } catch (error) { console.error(error); setStatus('error'); } }, []); const getStatusLabel = useCallback(() => { switch(status) { case 'saving': return 'Saving...'; case 'saved': return 'Saved!'; case 'error': return 'Error: There was an error saving your settings.'; case 'initial': default: return ''; } }, [status]) const statusLabel = getStatusLabel(); return ( <SettingsView onSave={saveSettings} statusMessage={statusLabel} > <Box css={{ padding:'medium', backgroundColor: 'container', }} > <Box css={{ font: 'lead' }} > Please enter a greeting </Box> <Box css={{ marginBottom: 'medium', font: 'caption' }} > Saved value: {storedValue || 'None'} </Box> <TextField id="greeting" name="greeting" type="text" label="Greeting:" size="medium" /> </Box> </SettingsView> ); }; export default AppSettings;