FocusView
FocusView を使用して、エンドユーザーが特定のタスクを実行する専用スペースを開きます。
FocusView
コンポーネントは他の View
コンポーネントから開くことができ、開発者はエンドユーザーが特定のタスクを実行する専用スペースを開くことができます。以下はその例です。
- データベースに新しいエントリーを作成するための詳細を入力する
- ウィザードを経て次のステップを決定する
- 指示されたアクションをユーザーが実行することを確認する
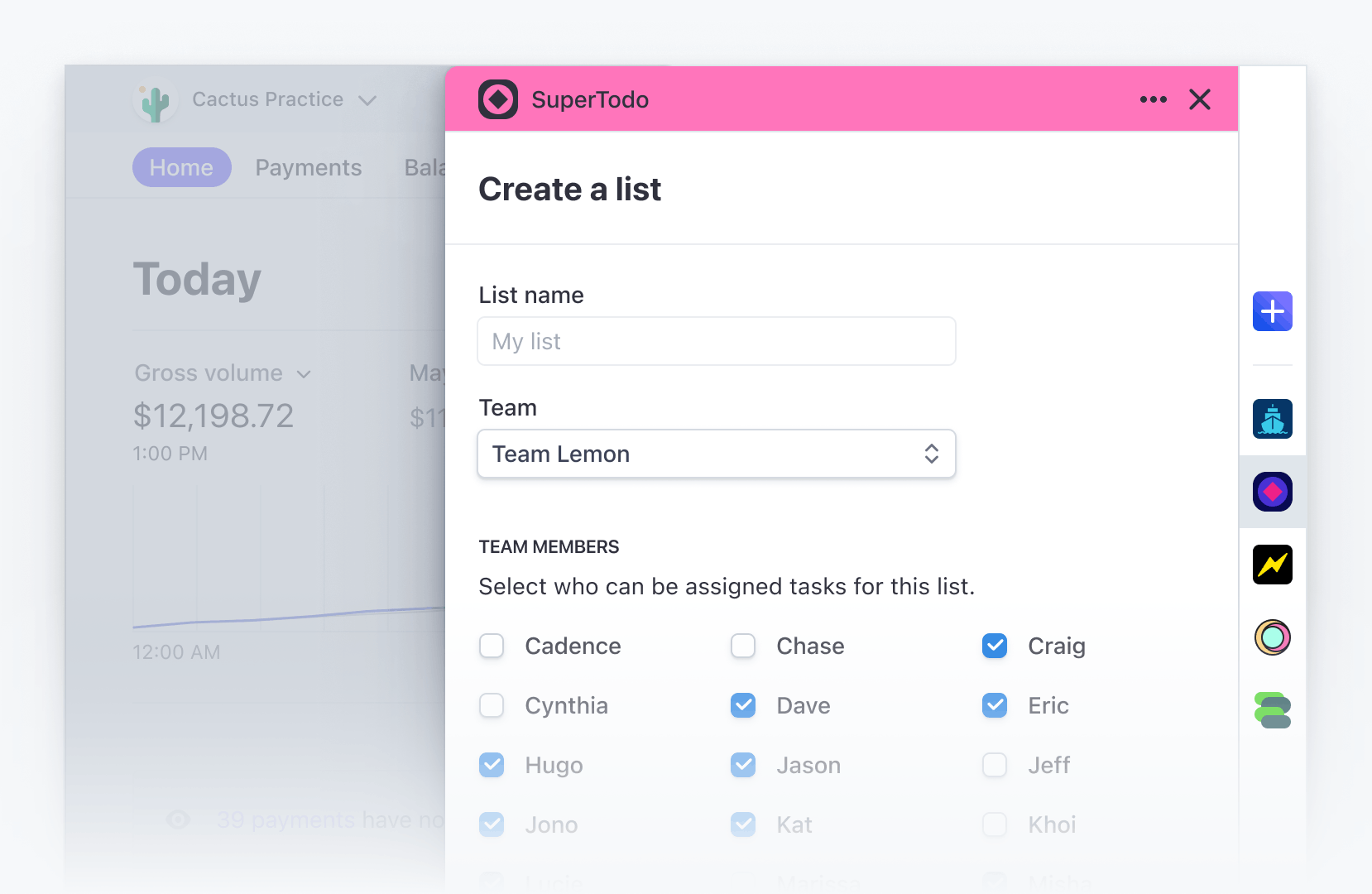
FocusView のデザイン
FocusView
は、ContextView
の子にする必要があります。FocusView
を条件でラップするのではなく、shown
プロパティーを使用して表示状態を管理します。詳細については、ContextView をご覧ください。
FocusView
コンポーネントをアプリに追加するには、以下のようにします。
import {FocusView} from '@stripe/ui-extension-sdk/ui';
Props
Prop | Type | Description |
---|---|---|
children Required |
| The contents of the FocusView. |
title Required |
| The title of the FocusView. This will be displayed at the top
of the drawer under your app's name. |
confirmCloseMessages |
| If provided, confirmCloseMessages will be displayed when the user closes the FocusView. |
footerContent |
| React node adjacent to any actions in the footer. |
primaryAction |
| A primary call to action ("Save" or "Continue") button placed in the footer. |
secondaryAction |
| A secondary call to action ("Cancel") button placed in the footer. |
setShown |
| Allows the FocusView to manage shown state if a user requests to close the window, or if
it needs to stay open because of the close confirmation dialog. |
shown |
| Whether the FocusView should be shown or not. This property is maintained by a parent view. |
onClose Deprecated |
| (Deprecated, use `setShown` instead) If the user clicks out of the FocusView or presses
the escape button, this informs the extension that the user has closed the view. |
確認ダイアログを閉じる
confirmCloseMessages
を渡す際に、終了の確認ダイアログがすべての終了シナリオで正しく機能するように、setShown
プロパティを渡して、FocusView
が shown
のステータスを管理できるようにします。確認ダイアログを表示するタイミングを制御するには、次の例のように、ステータスを使用して条件付きで confirmCloseMessages
を FocusView
に渡します。
例
import { useState } from "react"; import { Box, Button, ContextView, FocusView, Select, } from "@stripe/ui-extension-sdk/ui"; type Mood = "Happy" | "Sad"; const confirmCloseMessages = { title: "Your mood will not be saved", description: "Are you sure you want to exit?", cancelAction: "Cancel", exitAction: "Exit", }; const MoodView = () => { const [mood, setMood] = useState<Mood>("Happy"); const [shown, setShown] = useState<boolean>(false); const [confirmClose, setConfirmClose] = useState<boolean>(false); const open = () => { setConfirmClose(true); setShown(true); }; const closeWithoutConfirm = () => { setConfirmClose(false); setShown(false); }; const closeWithConfirm = () => { setShown(false); }; const updateMood = (newMood: Mood) => { setMood(newMood); closeWithoutConfirm(); }; return ( <ContextView title="Mood picker" description="This section communicates my extension's feelings" > <FocusView title="Pick your mood" shown={shown} setShown={setShown} confirmCloseMessages={confirmClose ? confirmCloseMessages : undefined} secondaryAction={<Button onPress={closeWithConfirm}>Cancel</Button>} > <Select onChange={(e) => updateMood(e.target.value as Mood)}> <option label="">Select mood</option> <option label="Happy">Happy</option> <option label="Sad">Sad</option> </Select> </FocusView> <Box css={{ stack: "x", gap: "medium" }}> <Box css={{ font: "subheading", color: mood === "Happy" ? "success" : "info", }} > {mood} </Box> <Button onPress={open}>Change mood</Button> </Box> </ContextView> ); }; export default MoodView;