Apple Pay
允许客户在他们的 iPhone、iPad 或 Apple Watch 上用 Apple Pay 安全地付款。
Apple Pay 与大多数 Stripe 产品和功能兼容。Stripe 用户可以在 iOS 9 及更高版本的 iOS 应用程序以及 iOS 10 或 macOS Sierra 及以上版本的 Safari 网页上接受 Apple Pay。处理 Apple Pay 付款时没有额外费用,定价与其他银行卡交易一样。
Apple Pay 可由在受支持国家的参与银行那里开户的持卡人使用。有关更多信息,请参考 Apple 的参与银行文档。
付款流程
以下是您的结账页面的 Apple Pay 付款流程演示:
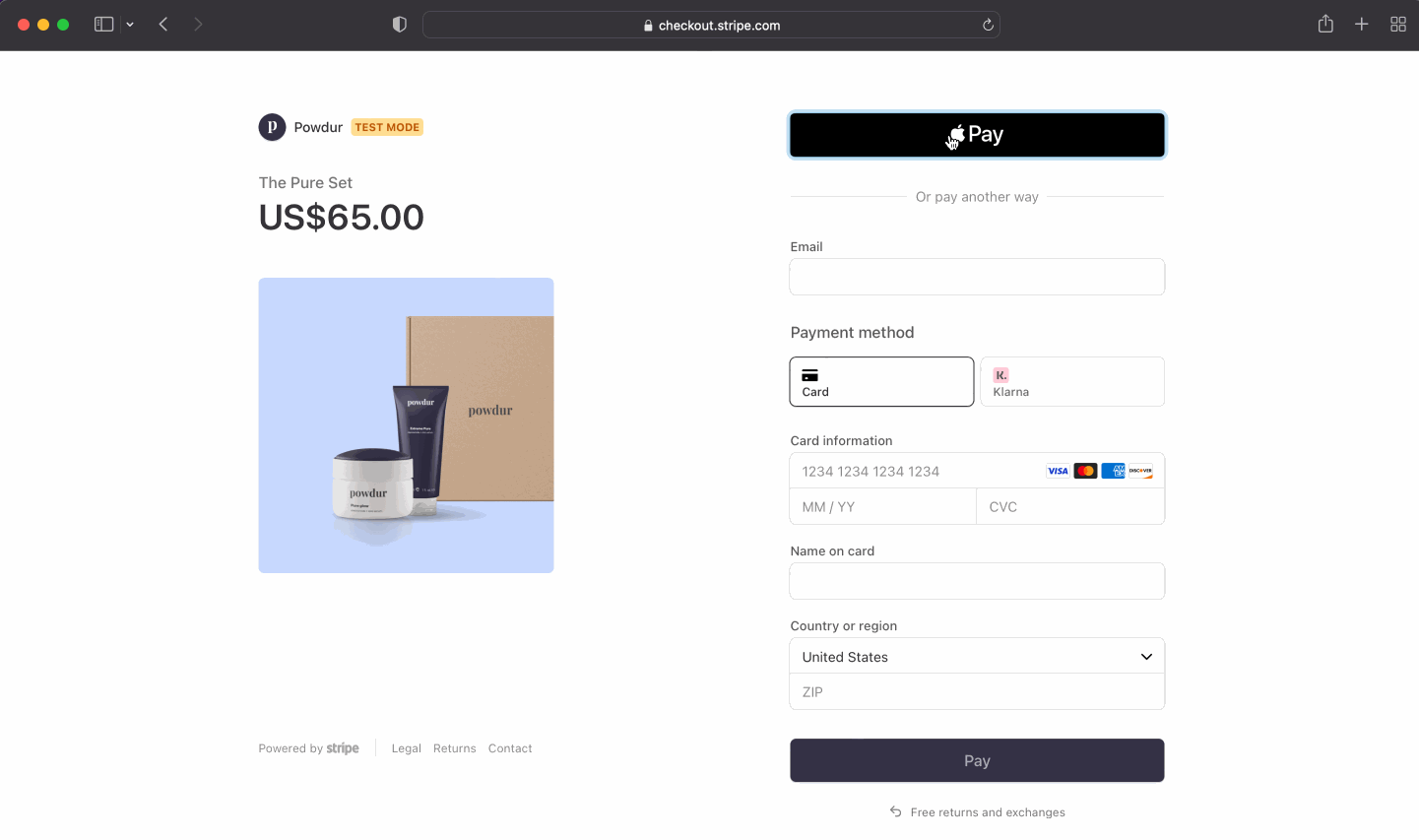
使用 Stripe 和 Apple Pay 与应用内购买
对于实物商品、服务和某些其他东西的销售,您的应用程序可以接受 Apple Pay 或其他任何 Stripe 支持的支付方式。这些付款是通过 Stripe 处理的,只需支付 Stripe 的手续费。但是,数字产品、内容和某些其他东西的销售必须使用 Apple 的应用内购买。这些付款由 Apple 公司处理,会收取交易费。
有关哪些类型的销售必须使用应用内购买的更多信息,请参见 Apple App Store 审查指南。
接受 Apple Pay
Stripe 提供了多种添加 Apple Pay 的方法。有关集成的详细信息,请选择您最喜欢的方法:
您可以使用 Stripe React Native SDK,同时接受 Apple Pay 和传统信用卡付款。开始前,您需要注册 Apple Developer Program 并在您的服务器和应用程序中设置 Stripe。接下来,执行这些步骤:
- 注册 Apple Merchant ID
- 创建新的 Apple Pay 证书
- 用 Xcode 集成
- 在 StripeProvider 中设置您的 Apple Merchant ID
- 检查是否支持 Apple Pay
- 出示支付表单
- 向 Stripe 提交付款
注意
注册 Apple Merchant ID
可通过在 Apple 开发人员网站注册新的标识符来获取 Apple 商家 ID。
在表单中填写描述和标识符。描述内容仅供您自己记录之用,之后可随时更改。Stripe 建议用您的应用的名称作为标识符(例如,merchant.
)。
集成 Xcode
将 Apple Pay 功能添加到您的应用程序。在 Xcode 中,打开您的项目设置,点击签名和功能选项卡,然后添加 Apple Pay 功能。此时,系统可能会提示您登入您的开发人员账户。选择您之前创建的商家 ID,您的应用程序就可以接受 Apple Pay 了。
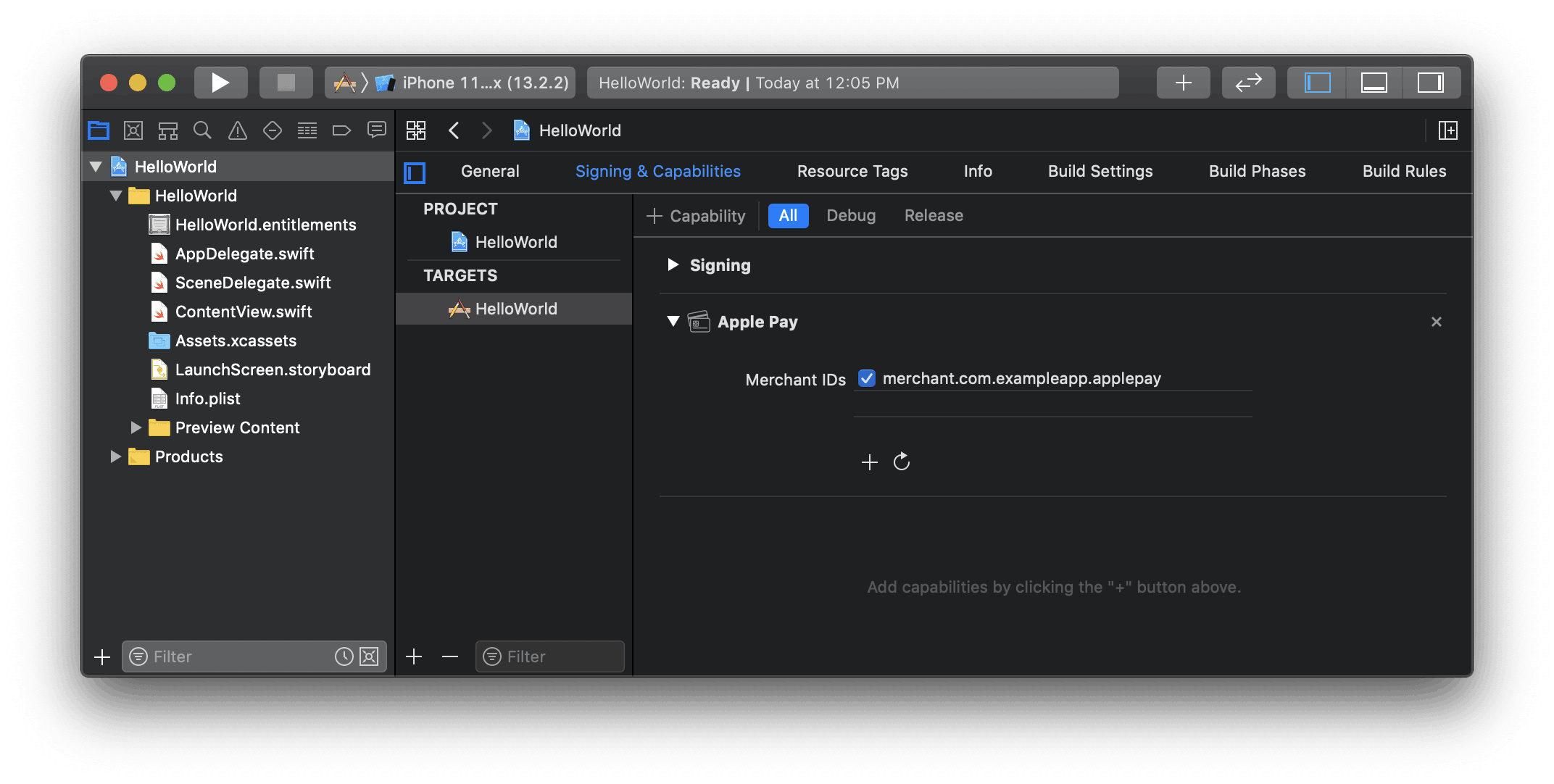
在 Xcode 中启用 Apple Pay 功能
在 StripeProvider 中设置您的 Apple Merchant ID
在 StripeProvider
组件中,指定您成功注册的 Apple Merchant ID:
import { StripeProvider } from '@stripe/stripe-react-native'; function App() { return ( <StripeProvider publishableKey="
" merchantIdentifier="merchant.com.{{YOUR_APP_NAME}}" > // Your app code here </StripeProvider> ); }pk_test_TYooMQauvdEDq54NiTphI7jx
检查是否支持 Apple Pay
在您的应用程序中显示 Apple Pay 此支付选项之前,请确定用户的设备支持 Apple Pay,并且他们的钱包中添加了银行卡:
import { PlatformPayButton, isPlatformPaySupported } from '@stripe/stripe-react-native'; function PaymentScreen() { const [isApplePaySupported, setIsApplePaySupported] = useState(false); useEffect(() => { (async function () { setIsApplePaySupported(await isPlatformPaySupported()); })(); }, [isPlatformPaySupported]); // ... const pay = async () => { // ... }; // ... return ( <View> {isApplePaySupported && ( <PlatformPayButton onPress={pay} type={PlatformPay.ButtonType.Order} appearance={PlatformPay.ButtonStyle.Black} borderRadius={4} style={{ width: '100%', height: 50, }} /> )} </View> ); }
创建 Payment Intent
服务器端
生成一个端点,创建一个具有 amount 和 currency 的 PaymentIntent。始终在服务器端决定扣款金额,这是一个可信的环境,客户端不行。这样可防止客户自己选择价格。
客户端
创建一个从您的服务器请求 PaymentIntent 的方法:
function PaymentScreen() { // ... const fetchPaymentIntentClientSecret = async () => { const response = await fetch(`${API_URL}/create-payment-intent`, { method: 'POST', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify({ some: 'value', }), }); const { clientSecret } = await response.json(); return clientSecret; }; // ... }
故障排除
如果您在尝试创建令牌时看到 Stripe API 出现错误,则很可能是您的 Apple Pay Certificate 有问题。如本页所述,您需要生成一个新证书并上传到 Stripe。请确保您使用的是从您的管理平台获得的 CSR,而不是您自己生成的那个。Xcode 经常错误地缓存旧的证书,所以除了生成新证书外,Stripe 建议创建一个新的 Apple Merchant ID。
如果您收到错误:
您尚未将您的 Apple 商家账户添加到 Stripe
您的应用程序好像正在发送用以前的(非 Stripe) CSR/Certificate 加密的数据。务必撤销您的 Apple Merchant ID 下任何不是由 Stripe 生成的证书。如果这样不能解决问题,请删除您的 Apple 账户中的商家 ID,然后重新创建它。然后,根据之前使用的相同(Stripe 提供的) CSR 创建一个新证书。您不需要将此新的证书上传到 Stripe。完成后,在您的应用程序中打开并关闭 Apple Pay Credentials,以确保正确刷新。
出示支付表单
在您的 PlatformPayButton
的 onPress
属性中,调用 confirmPlatformPayPayment
,打开一个 Apple Pay 表单。要在支付表单上显示客户购物车中的项目,将项目作为参数传递。
注意
在您的那些处理客户操作的代码中,不要在显示支付表单前包含任何复杂或异步操作。如果用户操作不直接调用支付表单,Apple Pay 会返回一个错误。
import { confirmPlatformPayPayment } from '@stripe/stripe-react-native'; function PaymentScreen() { // ... see above const pay = async () => { const clientSecret = await fetchPaymentIntentClientSecret() const { error } = await confirmPlatformPayPayment( clientSecret, { applePay: { cartItems: [ { label: 'Example item name', amount: '14.00', paymentType: PlatformPay.PaymentType.Immediate, }, { label: 'Total', amount: '12.75', paymentType: PlatformPay.PaymentType.Immediate, }, ], merchantCountryCode: 'US', currencyCode: 'USD', requiredShippingAddressFields: [ PlatformPay.ContactField.PostalAddress, ], requiredBillingContactFields: [PlatformPay.ContactField.PhoneNumber], }, } ); if (error) { // handle error } else { Alert.alert('Success', 'Check the logs for payment intent details.'); console.log(JSON.stringify(paymentIntent, null, 2)); } }; // ... see above }
测试 Apple Pay
要测试 Apple Pay,必须使用真实的信用卡卡号和测试 API 密钥。Stripe 会识别到您处于测试模式,并返回一个成功的测试卡令牌供您使用,因此您可以在不收款的情况下使用真实卡来测试付款。
您无法将Stripe测试卡或 Apple Pay 测试卡保存到 Apple Pay 钱包来测试 Apple Pay。