Stripe Payment Element
Accept payment methods from around the globe with a secure, embeddable UI component.
Interested in using Stripe Tax, discounts, shipping, or currency conversion?
We’re developing a Payment Element integration that manages tax, discounts, shipping, and currency conversion. Read the Build a checkout page guide to learn more.
The Payment Element is a UI component for the web that accepts 40+ payment methods, validates input, and handles errors. Use it alone or with other elements in your web app’s frontend.
To try the Payment Element for yourself, start with one of these examples:
Create a Payment Element
This code creates a Payment Element and mounts it to the DOM:
const stripe = Stripe(
); const appearance = { /* appearance */ }; const options = { /* options */ }; const elements = stripe.elements({'pk_test_TYooMQauvdEDq54NiTphI7jx', appearance }); const paymentElement = elements.create('payment', options); paymentElement.mount('#payment-element');clientSecret
Accepting payments with the Payment Element requires additional backend code. See the quickstart or sample app to learn how this works.
Combine elements
The Payment Element interoperates with other elements. For instance, this form uses one additional element to autofill checkout details, and another to collect the shipping address.
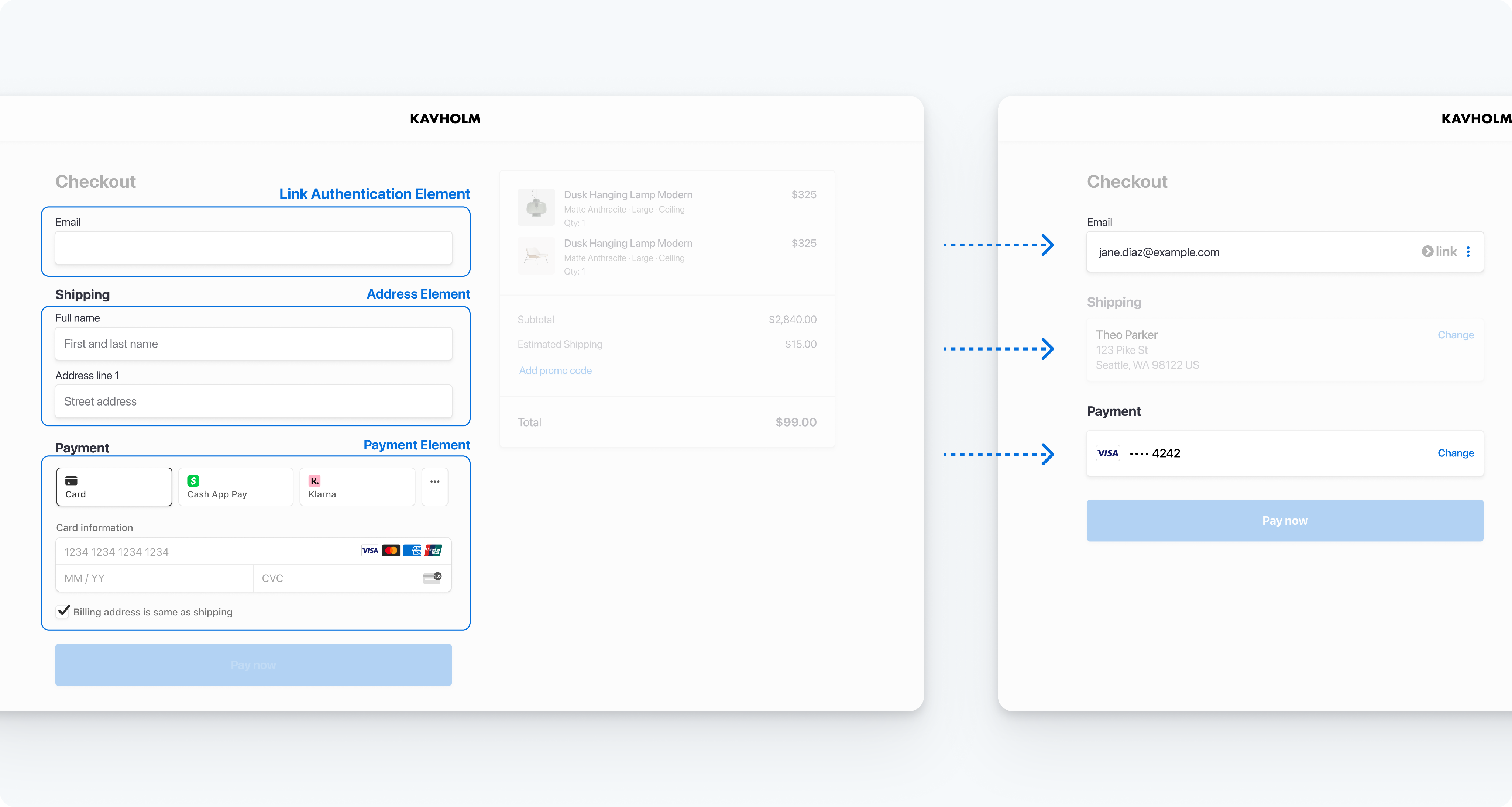
For the complete code for this example, see Add Link to an Elements integration.
You can also combine the Payment Element with the Express Checkout Element. In this case, wallet payment methods such as Apple Pay and Google Pay are only displayed in the Express Checkout Element to avoid duplication.
Payment methods
Stripe enables certain payment methods for you by default. We might also enable additional payment methods after notifying you. Use the Dashboard to enable or disable payment methods at any time. With the Payment Element, you can use Dynamic payment methods to:
- Manage payment methods in the Dashboard without coding
- Dynamically display the most relevant payment options based on factors such as location, currency, and transaction amount
For instance, if a customer in Germany is paying in EUR, they see all the active payment methods that accept EUR, starting with ones that are widely used in Germany.
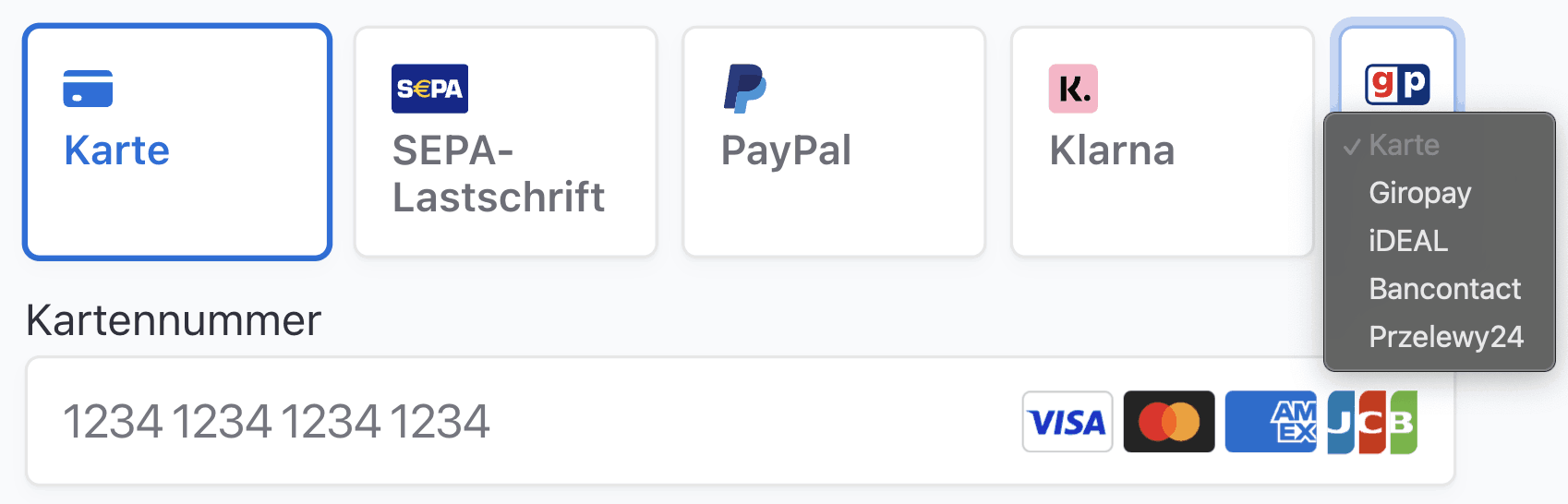
Show payment methods in order of relevance to your customer
To further customize how payment methods render, such as by filtering card brands that you don’t want to support, see Customize payment methods. To add payment methods integrated outside of Stripe, see External payment methods.
If your integration requires you to list payment methods manually, see Manually list payment methods.
Layout
You can customize the Payment Element’s layout to fit your checkout flow. The following image is the same Payment Element rendered using different layout configurations.
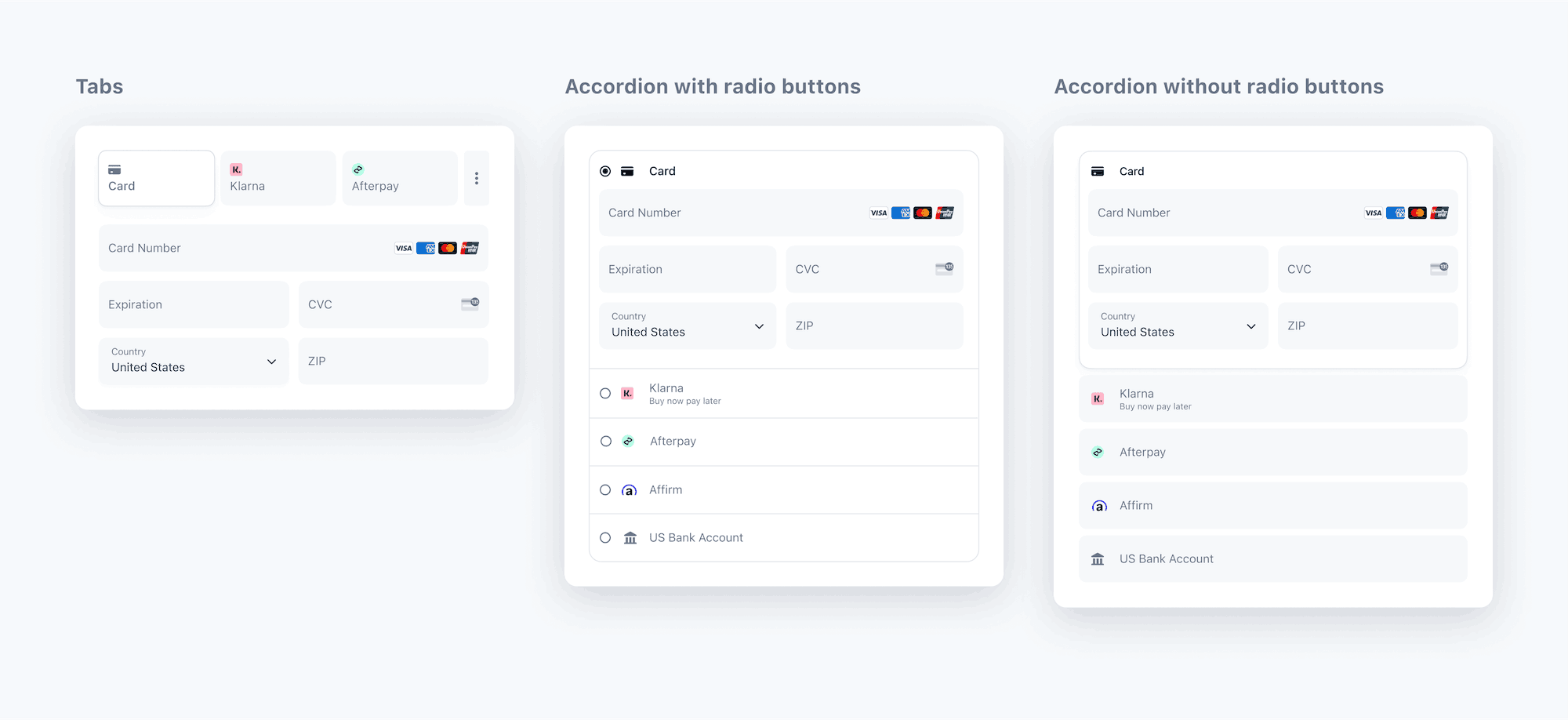
Payment Element with different layouts.
Appearance
Use the Appearance API to control the style of all elements. Choose a theme or update specific details.
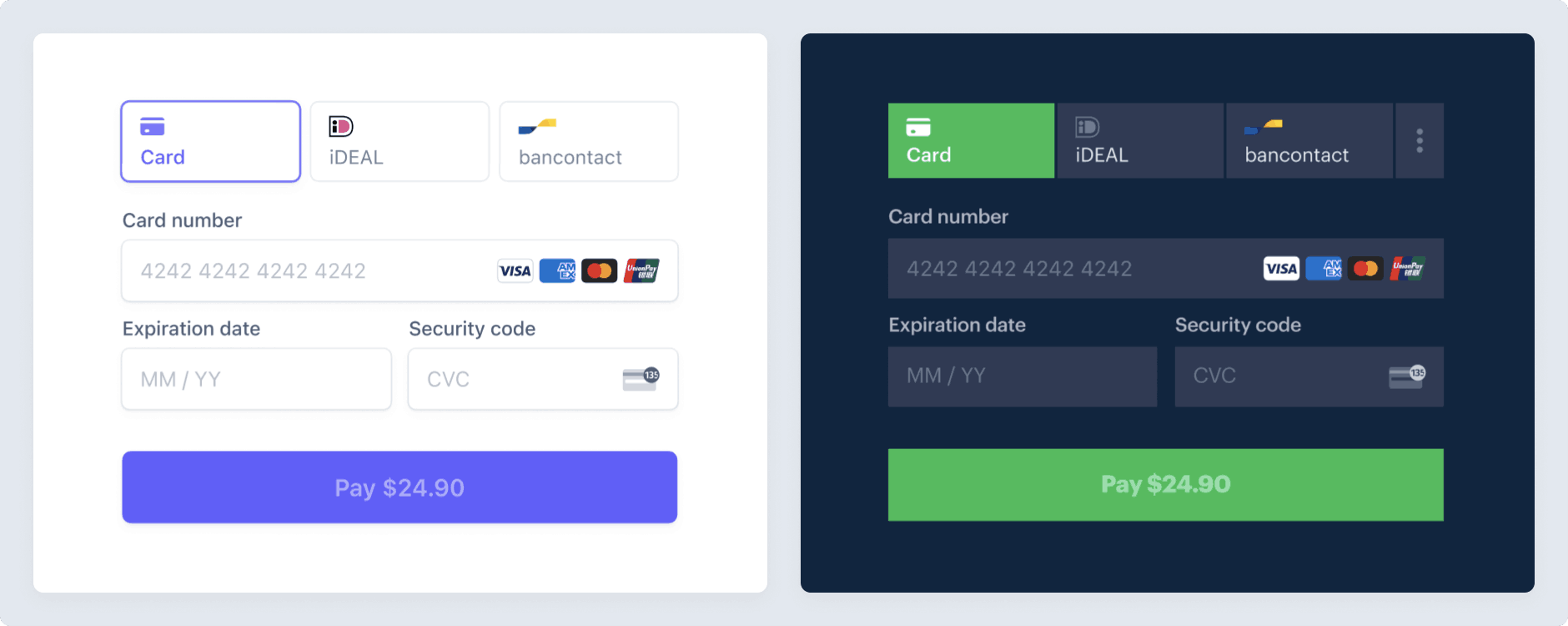
For instance, choose the “flat” theme and override the primary text color.
const stripe = Stripe(
); const appearance = { theme: 'flat', variables: { colorPrimaryText: '#262626' } };'pk_test_TYooMQauvdEDq54NiTphI7jx'
See the Appearance API documentation for a full list of themes and variables.
Options
Stripe elements support more options than these. For instance, display your business name using the business option.
const stripe = Stripe(
); const appearance = { /* appearance */}; const options = { business: "RocketRides" };'pk_test_TYooMQauvdEDq54NiTphI7jx'
The Payment Element supports the following options. See each options’s reference entry for more information.
layout | Layout for the Payment Element. |
defaultValues | Initial customer information to display in the Payment Element. |
business | Information about your business to display in the Payment Element. |
paymentMethodOrder | Order to list payment methods in. |
fields | Whether to display certain fields. |
readOnly | Whether payment details can be changed. |
terms | Whether mandates or other legal agreements are displayed in the Payment Element. The default behavior is to show them only when necessary. |
wallets | Whether to show wallets like Apple Pay or Google Pay. The default is to show them when possible. |