Accept in-app payments
Build a customized payments integration in your iOS, Android, or React Native app using the Embedded Payment Element.
The Embedded Payment Element is a customizable component that renders a list of payment methods that you can add into any screen in your app. When customers interact with payment methods in the list, the component opens individual bottom sheets to collect payment details.
A PaymentIntent flow allows you to create a charge in your app. In this integration, you render the Embedded Payment Element, create a PaymentIntent, and confirm a charge in your app.
Set up StripeServer-sideClient-side
Server-side 
This integration requires endpoints on your server that talk to the Stripe API. Use our official libraries for access to the Stripe API from your server:
Client-side 
The Stripe iOS SDK is open source, fully documented, and compatible with apps supporting iOS 13 or above.
Note
For details on the latest SDK release and past versions, see the Releases page on GitHub. To receive notifications when a new release is published, watch releases for the repository.
You also need to set your publishable key so that the SDK can make API calls to Stripe. To get started, you can hardcode the publishable key on the client while you’re integrating, but fetch the publishable key from your server in production.
// Set your publishable key: remember to change this to your live publishable key in production // See your keys here: https://dashboard.stripe.com/apikeys STPAPIClient.shared.publishableKey =
"pk_test_TYooMQauvdEDq54NiTphI7jx"
Enable payment methods
View your payment methods settings and enable the payment methods you want to support. You need at least one payment method enabled to create a PaymentIntent.
By default, Stripe enables cards and other prevalent payment methods that can help you reach more customers, but we recommend turning on additional payment methods that are relevant for your business and customers. See Payment method support for product and payment method support, and our pricing page for fees.
Collect payment detailsClient-side
The Embedded Mobile Payment Element is designed to be placed on the checkout page of your native mobile app. The element displays a list of payment methods and you can customize it to match your app’s look and feel.
When the customer taps the Card row, it opens a sheet where they can enter their payment method details. The button in the sheet says Continue by default and dismisses the sheet when tapped, which lets your customer finish payment in your checkout.
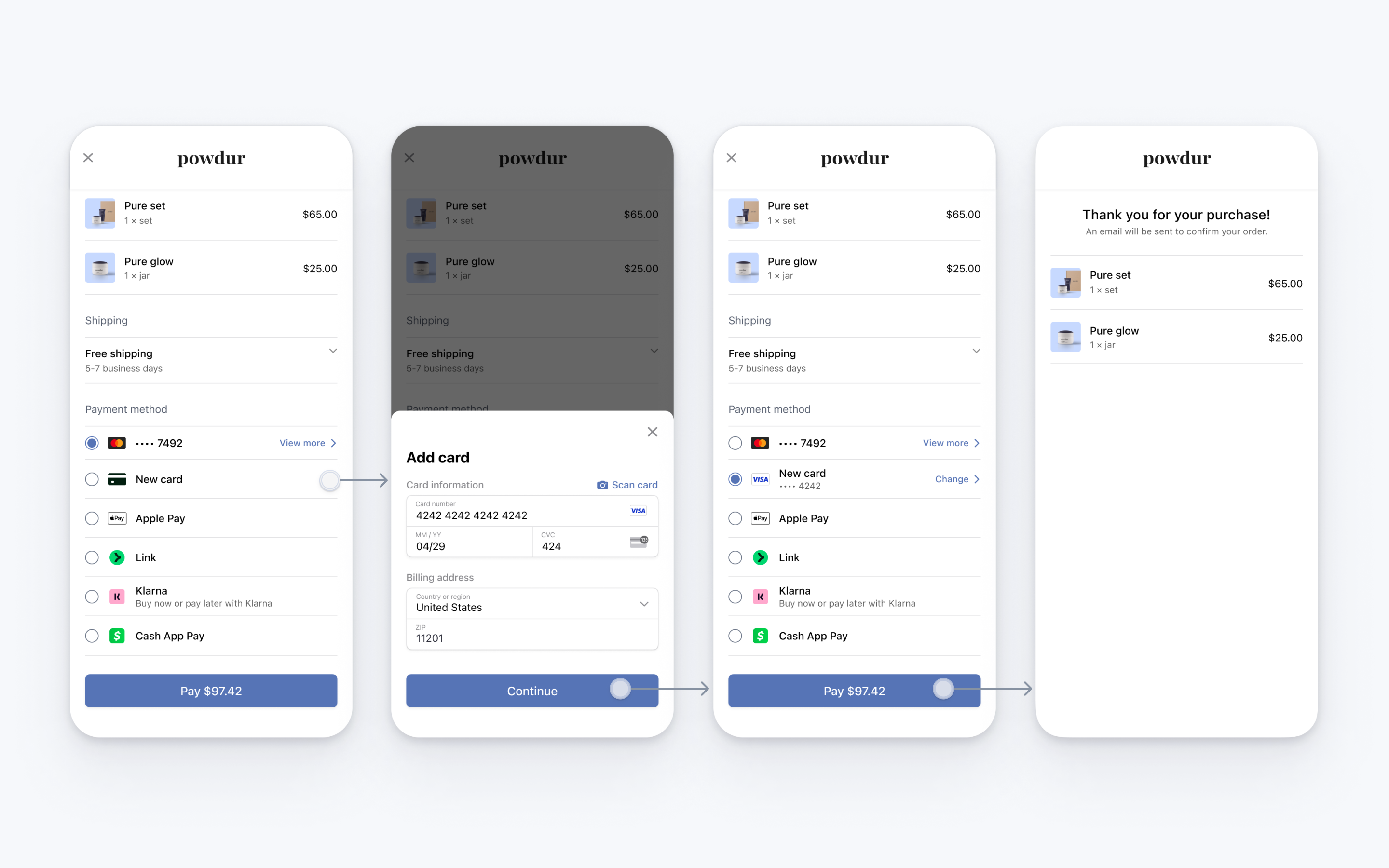
You can also configure the button to immediately complete payment instead of continuing. To do so, complete this step after following the guide.
Create a PaymentIntentServer-side
On your server, create a PaymentIntent with an amount and currency. You can manage payment methods from the Dashboard. Stripe handles the return of eligible payment methods based on factors such as the transaction’s amount, currency, and payment flow. To prevent malicious customers from choosing their own prices, always decide how much to charge on the server-side (a trusted environment) and not the client.
If the call succeeds, return the PaymentIntent client secret. If the call fails, handle the error and return an error message with a brief explanation for your customer.
Note
Verify that all IntentConfiguration properties match your PaymentIntent (for example, setup_
, amount
, and currency
).
Set up a return URLClient-side
The customer might navigate away from your app to authenticate (for example, in Safari or their banking app). To allow them to automatically return to your app after authenticating, configure a custom URL scheme and set up your app delegate to forward the URL to the SDK. Stripe doesn’t support universal links.
Additionally, set the returnURL on your EmbeddedPaymentElement.Configuration object to the URL for your app.
var configuration = EmbeddedPaymentElement.Configuration() configuration.returnURL = "your-app://stripe-redirect"
Handle post-payment eventsServer-side
Stripe sends a payment_intent.succeeded event when the payment completes. Use the Dashboard webhook tool or follow the webhook guide to receive these events and run actions, such as sending an order confirmation email to your customer, logging the sale in a database, or starting a shipping workflow.
Listen for these events rather than waiting on a callback from the client. On the client, the customer could close the browser window or quit the app before the callback executes, and malicious clients could manipulate the response. Setting up your integration to listen for asynchronous events is what enables you to accept different types of payment methods with a single integration.
In addition to handling the payment_
event, we recommend handling these other events when collecting payments with the Payment Element:
Event | Description | Action |
---|---|---|
payment_intent.succeeded | Sent when a customer successfully completes a payment. | Send the customer an order confirmation and fulfill their order. |
payment_intent.processing | Sent when a customer successfully initiates a payment, but the payment has yet to complete. This event is most commonly sent when the customer initiates a bank debit. It’s followed by either a payment_ or payment_ event in the future. | Send the customer an order confirmation that indicates their payment is pending. For digital goods, you might want to fulfill the order before waiting for payment to complete. |
payment_intent.payment_failed | Sent when a customer attempts a payment, but the payment fails. | If a payment transitions from processing to payment_ , offer the customer another attempt to pay. |
Test the integration
See Testing for additional information to test your integration.