Create direct charges
Create charges directly on the connected account and collect fees.
Create direct charges when customers transact directly with a connected account, often unaware of your platform’s existence. With direct charges:
- The payment appears as a charge on the connected account, not your platform’s account.
- The connected account’s balance increases with every charge.
- Your account balance increases with application fees from every charge.
This charge type is best suited for platforms providing software as a service. For example, Shopify provides tools for building online storefronts, and Thinkific enables educators to sell online courses.
Note
We recommend using direct charges for connected accounts that have access to the full Stripe Dashboard.
Redirect to a Stripe-hosted payment page using Stripe Checkout. See how this integration compares to Stripe’s other integration types.
Integration effort
Integration type
Redirect to Stripe-hosted payment page
UI customization
First, register for a Stripe account.
Use our official libraries to access the Stripe API from your application:
Create a Checkout SessionClient-sideServer-side
A Checkout Session controls what your customer sees in the payment form such as line items, the order amount, and currency. Add a checkout button to your website that calls a server-side endpoint to create a Checkout Session.
<html> <head> <title>Checkout</title> </head> <body> <form action="/create-checkout-session" method="POST"> <button type="submit">Checkout</button> </form> </body> </html>
On your server, create a Checkout Session and redirect your customer to the URL returned in the response.
line_
- This attribute represents items that your customer is purchasing and shows up in the Stripe-hosted checkout page.items payment_
- This attribute specifies the amount your platform deducts from the transaction as an application fee. After the payment is processed on the connected account, theintent_ data[application_ fee_ amount] application_
is transferred to the platform. See collect fees for more information.fee_ amount success_
- Stripe redirects the customer to the success URL after they complete a payment and replaces theurl {CHECKOUT_
string with the Checkout Session ID. Use this to retrieve the Checkout Session and inspect the status to decide what to show your customer. You can also append your own query parameters, which persist through the redirect process. See customize redirect behavior with a Stripe-hosted page for more information.SESSION_ ID} Stripe-Account
- This header indicates a direct charge for your connected account. The connected account’s branding is used in Checkout, which allows their customers to feel like they’re interacting directly with the connected account instead of your platform.
Charges that you create directly on the connected account are reported only on that account. These charges aren’t shown in your platform’s Dashboard or exports. Direct charges are included in reports and Sigma for connected accounts that your platform controls. You can always retrieve this information using the Stripe API.
Handle post-payment eventsServer-side
Stripe sends a checkout.session.completed event when the payment completes. Use a webhook to receive these events and run actions, like sending an order confirmation email to your customer, logging the sale in a database, or starting a shipping workflow.
Listen for these events rather than waiting on a callback from the client. On the client, the customer could close the browser window or quit the app before the callback executes. Some payment methods also take 2-14 days for payment confirmation. Setting up your integration to listen for asynchronous events enables you to accept multiple payment methods with a single integration.
Stripe recommends handling all of the following events when collecting payments with Checkout:
Event | Description | Next steps |
---|---|---|
checkout.session.completed | The customer has successfully authorized the payment by submitting the Checkout form. | Wait for the payment to succeed or fail. |
checkout.session.async_payment_succeeded | The customer’s payment succeeded. | Fulfill the purchased goods or services. |
checkout.session.async_payment_failed | The payment was declined, or failed for some other reason. | Contact the customer through email and request that they place a new order. |
These events all include the Checkout Session object. After the payment succeeds, the underlying PaymentIntent status changes from processing
to succeeded
or a failure status.
Test the integration
See Testing for additional information to test your integration.
Collect fees 
When a payment is processed, your platform can take a portion of the transaction in the form of application fees. You can set application fee pricing in two ways:
- Use the Platform Pricing Tool to set and test pricing rules. This no-code feature in the Stripe Dashboard is currently only available for platforms responsible for paying Stripe fees.
- Set your pricing rules in-house, specifying application fees directly in a PaymentIntent. Fees set with this method override the pricing logic specified in the Platform Pricing Tool.
Your platform can take an application fee with the following limitations:
- The value of
application_
must be positive and less than the amount of the charge. The application fee collected is capped at the captured amount of the charge.fee_ amount - There are no additional Stripe fees on the application fee itself.
- In line with Brazilian regulatory and compliance requirements, platforms based outside of Brazil, with Brazilian connected accounts can’t collect application fees through Stripe.
- The currency of
application_
depends upon a few multiple currency factors.fee_ amount
The resulting charge’s balance transaction includes a detailed fee breakdown of both the Stripe and application fees. To provide a better reporting experience, an Application Fee is created after the fee is collected. Use the amount
property on the application fee object for reporting. You can then access these objects with the Application Fees endpoint.
Earned application fees are added to your available account balance on the same schedule as funds from regular Stripe charges. Application fees are viewable in the Collected fees section of the Dashboard.
Caution
Application fees for direct charges are created asynchronously by default. If you expand the application_
object in a charge creation request, the application fee is created synchronously as part of that request. Only expand the application_
object if you must, because it increases the latency of the request.
To access the application fee objects for application fees that are created asynchronously, listen for the application_fee.created webhook event.
Flow of funds with fees 
When you specify an application fee on a charge, the fee amount is transferred to your platform’s Stripe account. When processing a charge directly on the connected account, the charge amount—less the Stripe fees and application fee—is deposited into the connected account.
For example, if you make a charge of 10 USD with a 1.23 USD application fee (like in the previous example), 1.23 USD is transferred to your platform account. 8.18 USD (10 USD - 0.59 USD - 1.23 USD) is netted in the connected account (assuming standard US Stripe fees).
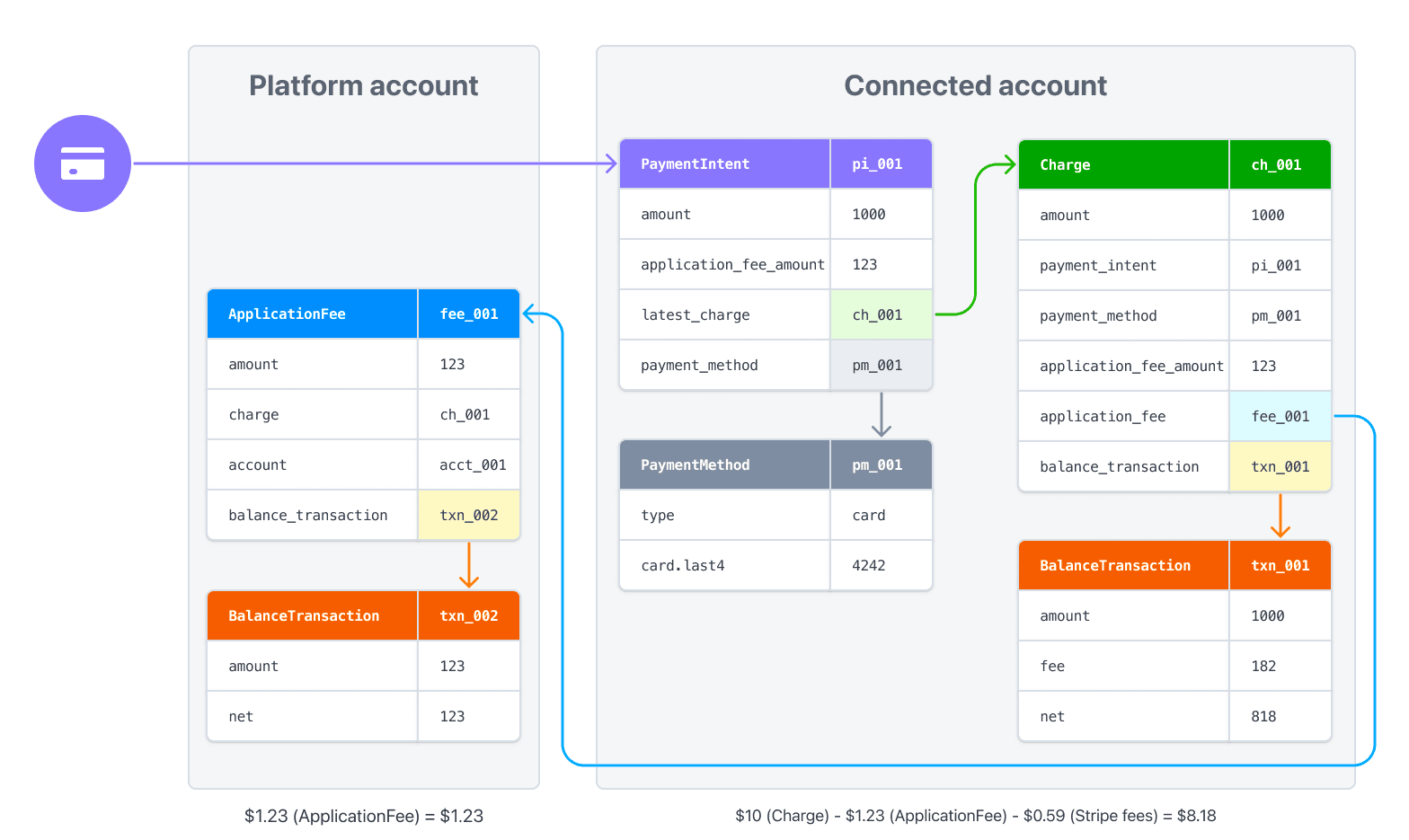
If you process payments in multiple currencies, read how currencies are handled in Connect.
Customize branding 
Your platform and connected accounts can use the Branding settings in the Dashboard to customize branding on the payments page. For direct charges, Checkout uses the brand settings of the connected account.
You can also use the API to update branding settings:
icon
- Displayed next to the business name in the header of the Checkout page.logo
- Used in place of the icon and business name in the header of the Checkout page.primary_
- Used as the background color on the Checkout page.color secondary_
- Used as the button color on the Checkout page.color
Issue refunds 
Just as platforms can create charges on connected accounts, they can also create refunds of charges on connected accounts. Create a refund using your platform’s secret key while authenticated as the connected account.
Application fees are not automatically refunded when issuing a refund. Your platform must explicitly refund the application fee or the connected account—the account on which the charge was created—loses that amount. You can refund an application fee by passing a refund_
value of true in the refund request:
By default, the entire charge is refunded, but you can create a partial refund by setting an amount
value as a positive integer. If the refund results in the entire charge being refunded, the entire application fee is refunded. Otherwise, a proportional amount of the application fee is refunded. Alternatively, you can provide a refund_
value of false and refund the application fee separately.