Accept payments for digital goods on iOS with a prebuilt payment page
Open Stripe Checkout in a browser to sell in-app goods or subscriptions.
In some countries, you can link to an external website to accept payments on iOS. As an example, this guide describes how to sell credits for consumption in your app. You use Stripe Checkout to redirect your customers to a Stripe-hosted payment page. If you have a limited number of products and prices, you can also use low-code Payment Links to accept payments for digital goods on iOS.
Note
Contact our sales team if your business is new to Stripe, processes a high volume of payments, and has advanced integration needs.
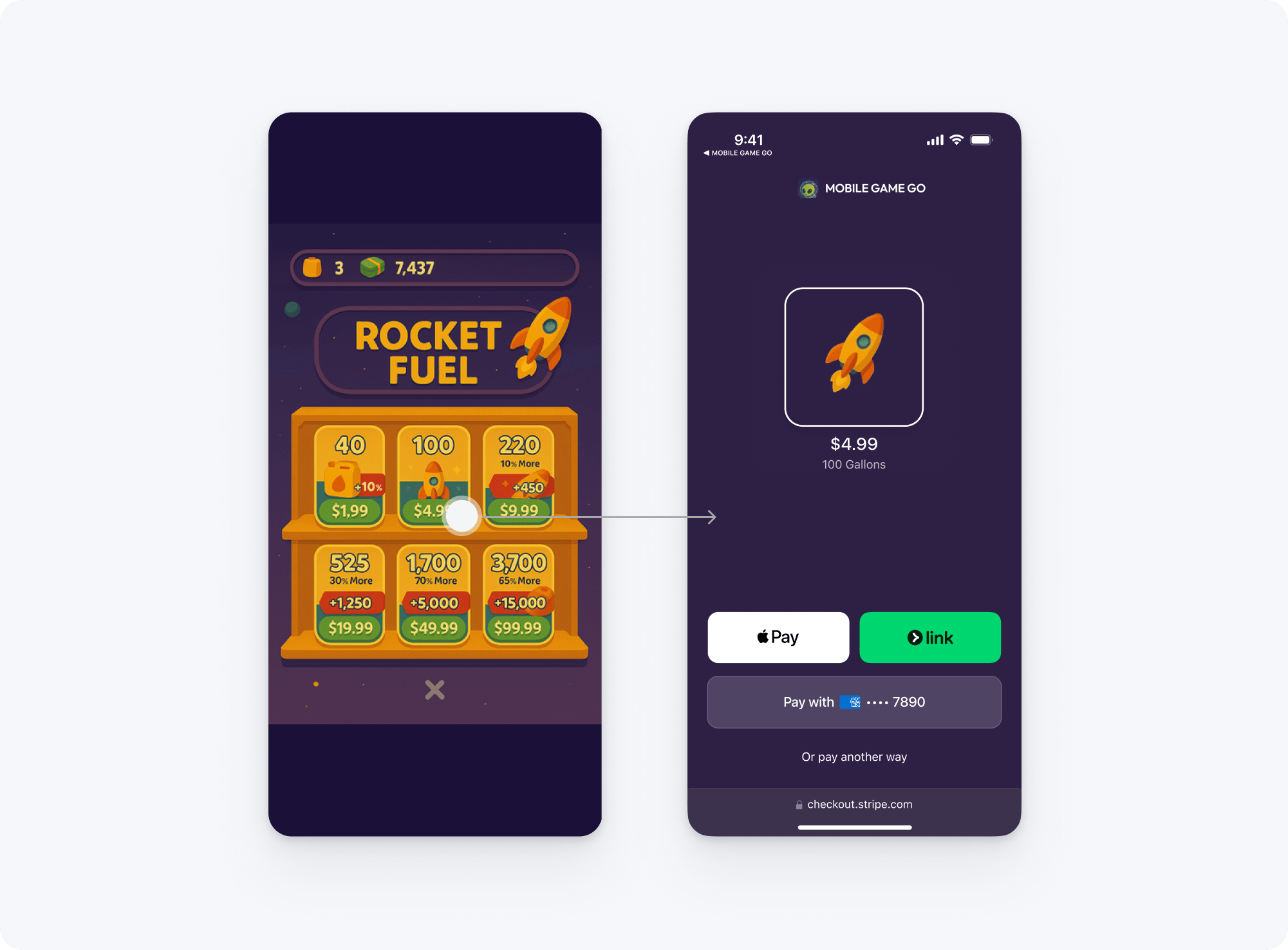
Link out of app for one-time payments
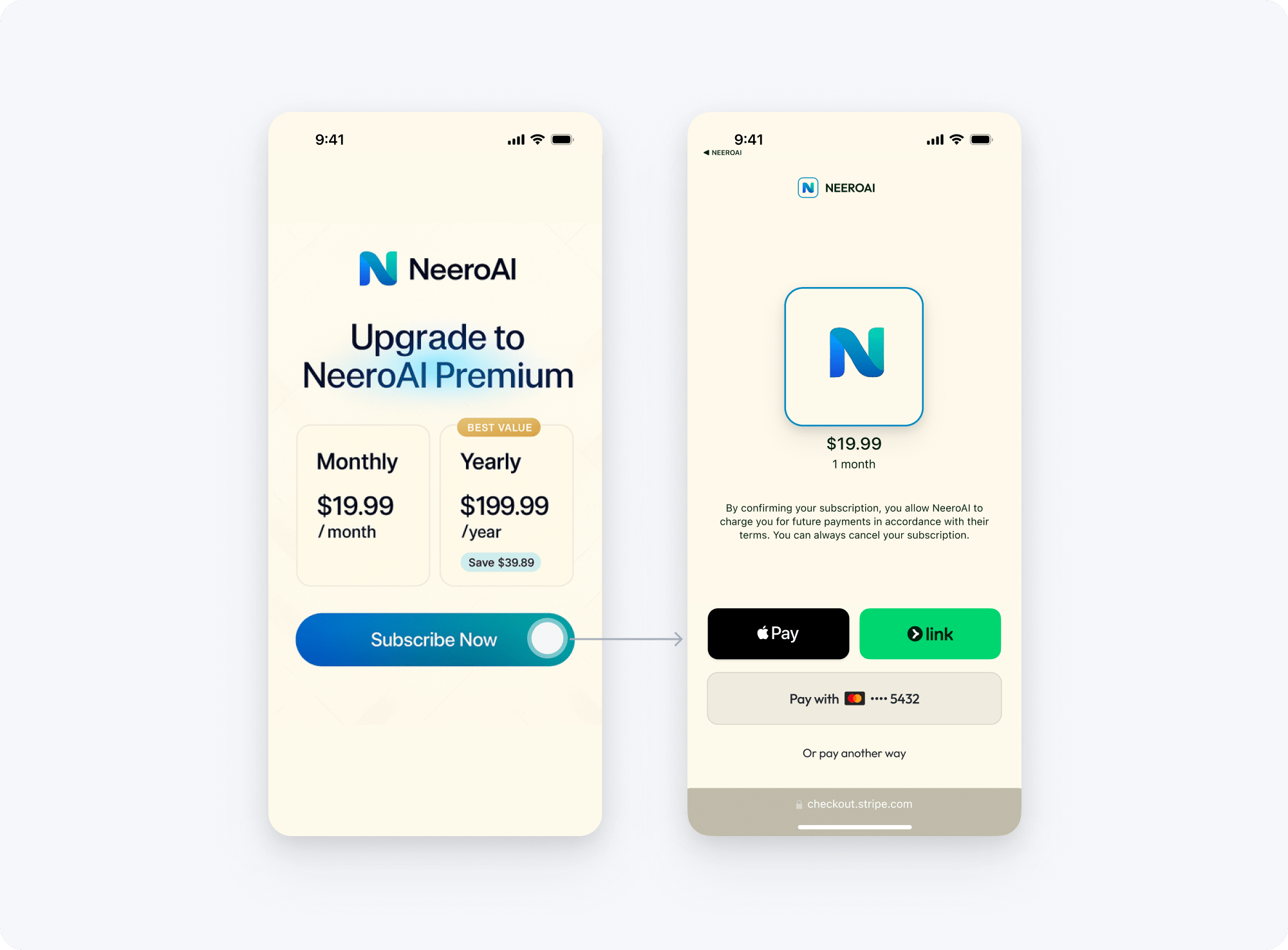
Link out of app for recurring or subscription payments
What you’ll build 
Note
This guide only describes the process for selling in-app digital goods. If you’re selling:
- Physical items
- Goods and services intended for consumption outside your app
- Real-time person-to-person services between two individuals
Use the native iOS payment guide instead.
This guide shows you how to:
- Collect payment information with Checkout.
- Model your credit packages with Products, Prices, and Customers.
- Use Universal Links to redirect directly to your app from Checkout.
- Monitor webhooks to update your customer’s in-app currency balance.
What isn’t covered 
This guide demonstrates how to add Stripe Checkout alongside your existing in-app purchase system. It doesn’t cover:
- User authentication. If you don’t have an existing authentication provider, you can use a third-party provider, such as Sign in with Apple or Firebase Authentication.
- Native in-app purchases. To implement in-app purchases using StoreKit, visit Apple’s in-app purchase guide.
Set up StripeServer-side
First, register for a Stripe account.
Then add the Stripe API library to your backend:
Next, install the Stripe CLI. The CLI provides the webhook testing you’ll need, and you can run it to create your products and prices.
For additional install options, see Get started with the Stripe CLI.
Create products and prices
Create your products and their prices in the Dashboard or with the Stripe CLI. You can model digital goods using one-off prices and subscriptions using recurring prices. You can also let your customer pay what they want (for example, to decide how many credits to buy), by selecting Customers choose what to pay.
This example uses a single product and price to represent a 100 coin bundle.
Create customersServer-side
Each time you create a Checkout session, create a Customer object for your user if one doesn’t already exist.
Warning
Make sure to store an association on your server between the user account and the Stripe customer ID. Without a way to associate a customer with a purchase, your customers can’t recover their purchases.
If your app doesn’t have an existing authentication provider, you can use Sign in with Apple.
Use the customer argument to pass their Customer ID when creating a Checkout Session. This ensures that all objects created during the session associate with the correct Customer object.
Note
If the customer changes their email on the Checkout page, the Customer object updates with the new email.
In payment
mode, we use the customer’s most recent card payment method to prefill the email, name, card details, and billing address on the Checkout page. Checkout requires a valid billing address to prefill the customer’s card details.
You can save payment method details to have Checkout automatically attach the payment method to the Customer for future reuse.
Set up universal linksClient-sideServer-side
Universal links allow Checkout to deep link into your app. To configure a universal link:
- Add an
apple-app-site-association
file to your domain. - Add an Associated Domains entitlement to your app.
- Add a fallback page for your Checkout redirect URLs.
Define the associated domains
Add a file to your domain at .well-known/apple-app-site-association to define the URLs your app handles. Prepend your App ID with your Team ID, which you can find on the Membership page of the Apple Developer Portal.
{ "applinks": { "apps": [], "details": [ { "appIDs": [ "A28BC3DEF9.com.example.MyApp1", "A28BC3DEF9.com.example.MyApp1-Debug" ], "components": [ { "/": "/checkout_redirect*", "comment": "Matches any URL whose path starts with /checkout_redirect" } ] } ] } }
Warning
You must serve the file with MIME type application/json
. Use curl -I
to confirm the content type.
curl -I https://example.com/.well-known/apple-app-site-association
See Apple’s page on supporting associated domains for more details.
Add an Associated Domains entitlement to your app
- Open the Signing & Capabilities pane of your app’s target.
- Click + Capability, then select Associated Domains.
- Add an entry for
applinks:example.
to the Associated Domains list.com
For more information on universal links, see Apple’s Universal Links for Developers page.
Although iOS intercepts links to the URLs defined in your apple-app-site-association
file, you might encounter situations where the redirect fails to open your app.
Make sure to create a fallback page at your success
and cancel
URLs.
Create a Checkout sessionServer-side
A Checkout Session is the programmatic representation of what your customer sees when they’re redirected to the payment form. Checkout Sessions expire 24 hours after creation. Configure it with:
- The customer ID
- The product ID (either a one time payment or subscription)
- An
origin_
set tocontext mobile_
to opt in to a UI that is optimized for app-to-web purchases.app - A
success_
, a universal link to redirect your customer to your app after they complete the payment.url - Optionally, a
cancel_
, a universal link to return your customer to your app if they click on your logo in Checkout.url
Common mistake
Create the Checkout Session with origin_
to opt in to a UI that is optimized for app-to-web purchases.
After creating a Checkout Session, return the URL from the response to your app.
Note
Apple Pay is enabled by default and automatically appears in Checkout when a customer uses a supported device. You can accept additional payment methods by using dynamic payment methods.
Open Checkout in SafariClient-side
Add a checkout button to your app. This button:
- Calls your server-side endpoint to create a Checkout session.
- Returns the Checkout session to the client.
- Opens the session URL in Safari.
import Foundation import SwiftUI import StoreKit struct BuyCoinsView: View { @EnvironmentObject var myBackend: MyServer @State var paymentComplete = false var body: some View { // Check if payments are blocked by Parental Controls on this device. if !SKPaymentQueue.canMakePayments() { Text("Payments are disabled on this device.") } else { if paymentComplete { Text("Payment complete!") } else { Button { myBackend.createCheckoutSession { url in UIApplication.shared.open(url, options: [:], completionHandler: nil) } } label: { Text("Buy 100 coins") }.onOpenURL { url in // Handle the universal link from Checkout. if url.absoluteString.contains("success") { // The payment was completed. Show a success // page and fetch the latest customer entitlements // from your server. paymentComplete = true } } } } } }
Fetch the Checkout URL on the client
Use your server endpoint to fetch the checkout session.
class MyServer: ObservableObject { // The cached login token var token: String? func createCheckoutSession(completion: @escaping (URL) -> Void) { // Send the login token to the `/create_checkout_session` endpoint let request = URLRequest(url: URL(string: "https://example.com/create-checkout-session?token=\(self.token)")!) let task = URLSession.shared.dataTask(with: request, completionHandler: { (data, response, error) in guard let unwrappedData = data, let json = try? JSONSerialization.jsonObject(with: unwrappedData, options: []) as? [String : Any], let urlString = json["url"] as? String, let url = URL(string: urlString) else { // Handle error return } DispatchQueue.main.async { // Call the completion block with the Checkout session URL returned from the backend completion(url) } }) task.resume() } func login() { // Login using the server and set the login token. let request = URLRequest(url: URL(string: "https://example.com/login")!) let task = URLSession.shared.dataTask(with: request, completionHandler: { (data, response, error) in guard let unwrappedData = data, let json = try? JSONSerialization.jsonObject(with: unwrappedData, options: []) as? [String : Any], let token = json["token"] as? String else { // Handle error return } self.token = token }) task.resume() } }
Handle order fulfillmentServer-side
After the purchase succeeds, Stripe sends you a checkout.
webhook. When you receive this event, you can add the coins to the customer on your server.
Checkout redirects your customer to the success_
when you acknowledge you received the event. In scenarios where your endpoint is down or the event isn’t acknowledged properly, Checkout redirects the customer to the success_
10 seconds after a successful payment.
For testing purposes, you can monitor events in the Dashboard or using the Stripe CLI. For production, set up a webhook endpoint and subscribe to appropriate event types. If you don’t know your STRIPE_
key, click the webhook in the Dashboard to view it.
Testing 
You should now have a working checkout button that redirects your customer to Stripe Checkout.
- Click the checkout button, which redirects you to the Stripe Checkout payment form.
- Enter the test number , a three-digit CVC, a future expiration date, and any valid postal code.
- Tap Pay.
- The
checkout.
webhook fires, and Stripe notifies your server about the transaction.session. completed - You’re redirected back to your app.
If your integration isn’t working, see the additional testing resources section below.