Use digital wallets with Issuing
Learn how to use Issuing to add cards to digital wallets.
Issuing allows users to add cards to digital wallets like Apple Pay and Google Pay. Stripe supports the addition of cards through two methods:
- Manual Provisioning: cardholders enter their card details into a phone’s wallet application to add it to their digital wallets.
- Push Provisioning: mobile applications allow users to add cards to their digital wallets straight from the app.
When a card is added to a digital wallet, a tokenized representation of that card is created. Network tokens are managed separately from cards. For more information about network tokens and how they work, see Token Management.
Manual Provisioning
Cardholders can add Stripe Issuing virtual cards and physical cards to their Apple Pay, Google Pay, and Samsung Pay wallets through manual provisioning.
To do so, cardholders open the wallet app on their phone and enter their card details. Stripe then sends a 6-digit verification code to the phone_
or email
of the cardholder associated with the card.
A card not supported error displays if neither field is set on the cardholder when the card was provisioned.
No code is required to implement manual provisioning, but the process to set it up can vary depending on the digital wallet provider and the country you’re based in:
US
Apple Pay wallets require approval from Apple. Check your digital wallets settings to view the status of Apple Pay in your account. You might need to submit an application before using Apple Pay.
Google Pay and Samsung Pay have no additional required steps.
EU/UK
Digital wallet integrations require additional approval from the Stripe partnership team. Get in touch with your account representative or contact Stripe for more information.
Apple Pay wallets require additional approval. Check your digital wallets settings to view the status of Apple Pay in your account. You might need to submit an application before using Apple Pay.
Push Provisioning
With push provisioning, cardholders can add their Stripe Issuing cards to their digital wallets using your app, by pressing an “add to wallet” button like the ones shown below.
Users must first complete manual provisioning steps to enable push provisioning in the US. In addition to manual provisioning approval, push provisioning requires you to integrate with the Stripe SDK.
This requires both approval processes through Stripe and code integration with the Stripe SDK for each platform you wish to support push provisioning on. Platform approvals cascade down to all of their connected accounts.
Samsung Pay push provisioning isn’t supported with our SDKs.
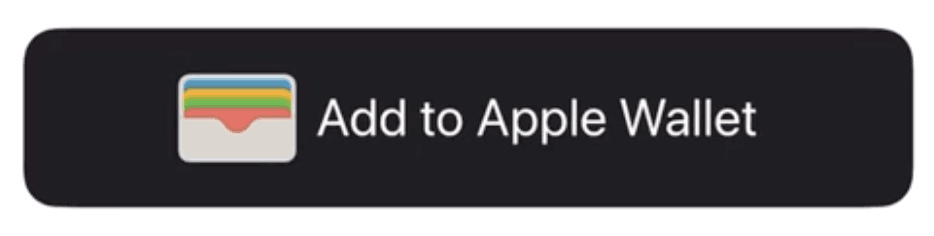
Request Access
Push provisioning requires a special entitlement from Apple called com.
. You can request it by emailing support-issuing@stripe.com. In your email, include your:
- Card network—Visa or MasterCard.
- Card name—This is the name of the card displayed in the wallet.
- App name—Your app’s name.
- Developer team ID—Found in your Apple Developer account settings under membership (for example,
2A23JCNA5E
). - ADAM ID—Your app’s unique numeric ID. Found in App Store Connect, or in the App Store link to your app (for example,
https://apps.
).apple. com/app/id123456789 - Bundle ID—Your app’s bundle identifier, also found in App Store Connect (for example,
com.
).example. yourapp
If you have multiple apps (such as for testing), that have any different fields for the above attributes, you’ll need to request access for each of these.
After we approve and apply your request, your app appears on the details page of a provisioned card in the Wallet app, and the PKSecureElementPass
object is available in your app by calling PKPassLibrary().
. You might need to remove and re-provision the card for the change to take effect.
Check eligibilityClient-side
Make sure you’ve integrated the latest version of the Stripe iOS SDK with your app.
Determine if the device is eligible to use push provisioning.
- Check that the value of
wallets[apple_
in the issued card ispay][eligible] true
. - Call
PKPassLibrary().
with thecanAddSecureElementPass(primaryAccountIdentifier:) wallets[primary_
from your card, and check that the result isaccount_ identifier] true
. If theprimary_
is empty, pass an empty string toaccount_ identifier canAddSecureElementPass()
.
Retrieve these values on your back end, then pass them to your app for the eligibility check.
Warning
You must check the server-side wallets[apple_
flag and the result of canAddSecureElementPass()
before showing the PKAddPassButton
. If you show an Add to Apple Wallet button without checking these values, App Review might reject your app.
For more context, see the code snippets and references to the sample app at each step. For this step, see how the sample app checks eligibility.
Provision a cardClient-side
When the user taps the PKAddPassButton
, create and present a PKAddPaymentPassViewController
, which contains Apple’s UI for the push provisioning flow.
Note
PKAddPaymentPassViewController
can use the primaryAccountIdentifier
from the previous step to determine if a card has already been provisioned on a specific device. For example, if the card has already been added to an iPhone, Apple’s UI offers to add it to a paired Apple Watch.
For more context, see how the sample app uses a PKAddPaymentPassViewController
.
The PKAddPaymentPassViewController
’s initializer takes a delegate that you need to implement – typically this can just be the view controller from which you’re presenting it. We provide a class called STPPushProvisioningContext
to help you implement these methods.
For more context, see how the sample app implements PKAddPaymentPassViewControllerDelegate
.
You can see that the STPPushProvisioningContext
’s initializer expects a keyProvider
. This is an instance of a class that implements the STPIssuingCardEphemeralKeyProvider
protocol.
This protocol defines a single required method, createIssuingCardKeyWithAPIVersion:completion
. To implement this method, make an API call to your backend. Your backend creates an Ephemeral Key object using the Stripe API, and returns it to your app. Your app then calls the provided completion handler with your backend’s API response.
For more context, see how the sample app implements STPIssuingCardEphemeralKeyProvider
.
Update your backendServer-side
The push provisioning implementation exposes methods that expect you to communicate with your own backend to create a Stripe Ephemeral Key and return a JSON of it to your app. This key is a short-lived API credential that you can use to retrieve the encrypted card details for a single instance of a card object.
To make sure that the object returned by the Stripe API is compatible with the version of the iOS or Android SDK you’re using, the Stripe SDK lets you know what API version it prefers. You must explicitly pass this API version to our API when creating the key.
{ "id": "ephkey_1G4V6eEEs6YsaMZ2P1diLWdj", "object": "ephemeral_key", "associated_objects": [ { "id": "ic_1GWQp6EESaYspYZ9uSEZOcq9", "type": "issuing.card" } ], "created": 1586556828, "expires": 1586560428, "livemode": false, "secret": "ek_test_YWNjdF8xRmdlTjZFRHelWWxwWVo5LEtLWFk0amJ2N0JOa0htU1JzEZkd2RpYkpJdnM_00z2ftxCGG" }
For more context, see how the sample backend creates a Stripe Ephemeral Key.
Testing
The com.
entitlement only works with distribution provisioning profiles, meaning even after you obtain it, the only way to test the end-to-end push provisioning flow is by distributing your app with TestFlight or the App Store.
To help with testing, we provide a mock version of PKAddPaymentPassViewController
called STPFakeAddPaymentPassViewController
that can be used interchangeably during testing.
To build the sample app, follow the steps in the readme. You don’t need to build the app to follow the instructions above.