Build a subscriptions integration
Create and manage subscriptions to accept recurring payments.
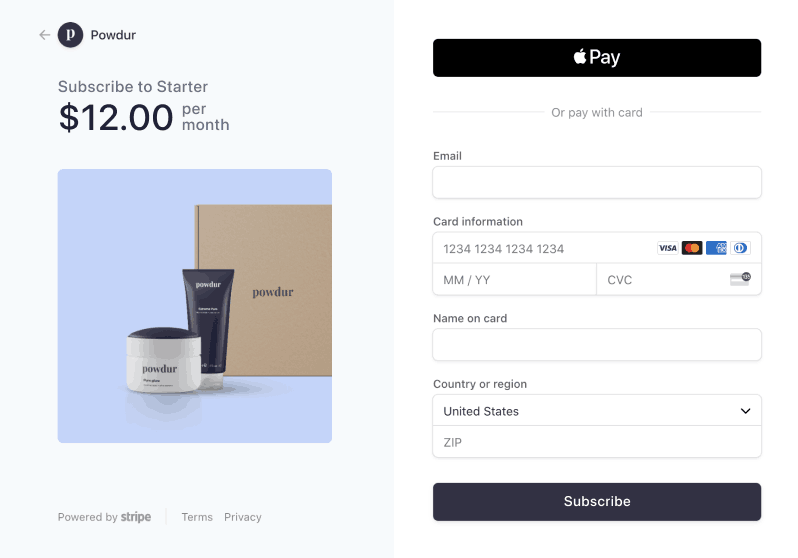
Customize logo, images, and colors.
Use prebuilt hosted forms to collect payments and manage subscriptions.
Clone a sample integration from GitHub.
For an immersive version of this guide, see the Billing integration quickstart.
View the demo to see a hosted example.
What you’ll build 
This guide describes how to sell fixed-price monthly subscriptions using Stripe Checkout.
This guide shows you how to:
- Model your business by building a product catalog
- Add a Checkout session to your site, including a button and success and cancellation pages
- Monitor subscription events and provision access to your service
- Set up the customer portal
- Add a customer portal session to your site, including a button and redirect
- Let customers manage their subscription through the portal
After you complete the integration, you can extend it to:
- Display taxes
- Apply discounts
- Offer customers a free trial period
- Add more payment methods
- Integrate the hosted invoice page
- Use Checkout in setup mode
- Set up metered billing, pricing tiers, and usage-based pricing
- Manage prorations
- Allow customers to subscribe to multiple products
- Integrate entitlements to manage access to your product’s features
Set up Stripe
Install the Stripe client of your choice:
Install the Stripe CLI (optional). The CLI provides webhook testing, and you can run it to create your products and prices.
For additional install options, see Get started with the Stripe CLI.
Create the pricing modelDashboard or Stripe CLI
Create your products and their prices in the Dashboard or with the Stripe CLI.
This example uses a fixed-price service with two different service-level options: Basic and Premium. For each service-level option, you need to create a product and a recurring price. (If you want to add a one-time charge for something like a setup fee, create a third product with a one-time price. To keep things simple, this example doesn’t include a one-time charge.)
In this example, each product bills at monthly intervals. The price for the Basic product is 5 USD. The price for the Premium product is 15 USD.
If you offer multiple billing intervals, use Checkout to upsell customers on longer billing intervals and collect more revenue upfront.
For other pricing models, see Billing examples.
Create a Checkout SessionClient and Server
Add a checkout button to your website that calls a server-side endpoint to create a Checkout Session.
<html> <head> <title>Checkout</title> </head> <body> <form action="/create-checkout-session" method="POST"> <!-- Note: If using PHP set the action to /create-checkout-session.php --> <input type="hidden" name="priceId" value="price_G0FvDp6vZvdwRZ" /> <button type="submit">Checkout</button> </form> </body> </html>
On the backend of your application, define an endpoint that creates the session for your frontend to call. You need these values:
- The price ID of the subscription the customer is signing up for—your frontend passes this value
- Your
success_
, a page on your website that Checkout returns your customer to after they complete the paymenturl
You can optionally provide cancel_
, a page on your website that Checkout returns your customer to if they cancel the payment process. You can also configure a billing cycle anchor to your subscription in this call.
If you created a one-time price in step 2, pass that price ID as well. After creating a Checkout Session, redirect your customer to the URL returned in the response.
Note
You can use lookup_keys to fetch prices rather than Price IDs. See the sample application for an example.
This example customizes the success_
by appending the Session ID. For more information about this approach, see the documentation on how to Customize your success page.
From your Dashboard, enable the payment methods you want to accept from your customers. Checkout supports several payment methods.
Provision and monitor subscriptionsServer
After the subscription signup succeeds, the customer returns to your website at the success_
, which initiates a checkout.
webhooks. When you receive a checkout.
event, you can provision the subscription. Continue to provision each month (if billing monthly) as you receive invoice.
events. If you receive an invoice.
event, notify your customer and send them to the customer portal to update their payment method.
To determine the next step for your system’s logic, check the event type and parse the payload of each event object, such as invoice.
. Store the subscription.
and customer.
event objects in your database for verification.
For testing purposes, you can monitor events in the Dashboard. For production, set up a webhook endpoint and subscribe to appropriate event types. If you don’t know your STRIPE_
key, click the webhook in the Dashboard to view it.
The minimum event types to monitor:
Event name | Description |
---|---|
checkout. | Sent when a customer clicks the Pay or Subscribe button in Checkout, informing you of a new purchase. |
invoice. | Sent each billing interval when a payment succeeds. |
invoice. | Sent each billing interval if there is an issue with your customer’s payment method. |
For even more events to monitor, see Subscription webhooks.
Configure the customer portalDashboard
The customer portal lets your customers directly manage their existing subscriptions and invoices.
Use the Dashboard to configure the portal. At a minimum, make sure to configure it so that customers can update their payment methods. See Integrating the customer portal for information about other settings you can configure.
Create a portal SessionServer
Define an endpoint that creates the customer portal session for your frontend to call. Here CUSTOMER_
refers to the customer ID created by a Checkout Session that you saved while processing the checkout.
webhook. You can also set a default redirect link for the portal in the Dashboard.
Pass an optional return_
value for the page on your site to redirect your customer to after they finish managing their subscription:
Send customers to the customer portalClient
On your frontend, add a button to the page at the success_
that provides a link to the customer portal:
<html> <head> <title>Manage Billing</title> </head> <body> <form action="/customer-portal" method="POST"> <!-- Note: If using PHP set the action to /customer-portal.php --> <button type="submit">Manage Billing</button> </form> </body> </html>
After exiting the customer portal, the Customer returns to your website at the return_
. Continue to monitor webhooks to track the state of the Customer’s subscription.
If you configure the customer portal to allow actions such as canceling a subscription, see Integrating the customer portal for additional events to monitor.
Test your integration
Test payment methods
Use the following table to test different payment methods and scenarios.
Payment method | Scenario | How to test |
---|---|---|
BECS Direct Debit | Your customer successfully pays with BECS Direct Debit. | Fill out the form using the account number 900123456 and BSB 000-000 . The confirmed PaymentIntent initially transitions to processing , then transitions to the succeeded status three minutes later. |
BECS Direct Debit | Your customer’s payment fails with an account_ error code. | Fill out the form using the account number 111111113 and BSB 000-000 . |
Credit card | The card payment succeeds and does not require authentication. | Fill out the credit card form using the credit card number 4242 4242 4242 4242 with any expiration, CVC, and postal code. |
Credit card | The card payment requires authentication. | Fill out the credit card form using the credit card number 4000 0025 0000 3155 with any expiration, CVC, and postal code. |
Credit card | The card is declined with a decline code like insufficient_ . | Fill out the credit card form using the credit card number 4000 0000 0000 9995 with any expiration, CVC, and postal code. |
SEPA Direct Debit | Your customer successfully pays with SEPA Direct Debit. | Fill out the form using the account number AT321904300235473204 . The confirmed PaymentIntent initially transitions to processing, then transitions to the succeeded status three minutes later. |
SEPA Direct Debit | Your customer’s payment intent status transition from processing to requires_ . | Fill out the form using the account number AT861904300235473202 . |
Monitoring events
Set up webhooks to listen to subscription change events like upgrades and cancellations. Read the guide to learn more about subscription webhooks. You can view events in the Dashboard or with the Stripe CLI.
For more details about testing your Billing integration, read the guide.