Connect to a reader
Connect your application to a Stripe Terminal reader.
Note
If you haven’t chosen a reader yet, compare the available Terminal readers and choose one that best suits your needs.
Tap to Pay lets users accept in-person contactless payments with compatible NFC-equipped Android devices or compatible iPhones. Tap to Pay includes support for Visa, Mastercard, American Express contactless cards, and NFC-based mobile wallets (Apple Pay, Google Pay, and Samsung Pay). Tap to Pay on iPhone and Android support is included in the native Terminal SDKs and enables payments directly in your mobile app.
Follow these steps to connect your app to the Tap to Pay reader on a supported device:
- Discover readers using the SDK to confirm device compatibility.
- Connect to a reader using the SDK to accept payments.
- Handle unexpected disconnects to make sure your user can continue to accept payments if the reader disconnects unexpectedly.
Create a locationServer-sideDashboard
Create a Location for each physical location where your readers operate. Set the display_
to represent the name of the business. Your customer sees the display_
on the device’s tap screen unless you explicitly provide the name of a business when you connect to a reader. You can edit existing locations as necessary to adjust this text.
If your business requires you to move your readers frequently, your locations can use an address that represents your primary place of business.
To create a location using the API, use the create location request.
Locations for the US require:
line1
city
state
postal_
code country
To create a location in the Dashboard, click the +New button on the Locations page.
Discover readers
Use the discoverReaders
method to determine hardware support for Tap to Pay on your device.
If your application runs on a device that doesn’t meet the requirements above, the discoverReaders
method returns an error.
export default function DiscoverReadersScreen() { const { discoverReaders, discoveredReaders } = useStripeTerminal({ onUpdateDiscoveredReaders: (readers) => { // The `readers` variable will contain an array of all the discovered readers. }, }); useEffect(() => { const { error } = await discoverReaders({ discoveryMethod: 'localMobile', }); }, [discoverReaders]); return <View />; }
Connect to a reader
To accept Tap to Pay payments, provide the discovered reader from the previous step to the connectLocalMobileReader
method.
On iPhones if you use destination charges with on_
, you must also pass the connected account ID to connectLocalMobileReader
.
const { reader, error } = await connectLocalMobileReader({ reader: selectedReader, locationId:
}); if (error) { console.log('connectLocalMobileReader error:', error); return; } console.log('Reader connected successfully', reader);'{{LOCATION_ID}}'
Account linking and Apple terms and conditions
Users are presented with Apple’s Tap to Pay on iPhone Terms and Conditions the first time they connect to the reader on iPhones. To register with Apple, you must specify a valid Apple ID representing your business before accepting the terms presented by Apple. You only need to perform this once per Stripe account. This flow isn’t presented on subsequent connections using the same Stripe account, including on other mobile devices.
Each connected account must accept the Terms and Conditions when:
- A Connect user creates direct charges
- A Connect user creates a destination charge and specifies an
on_
accountbehalf_ of
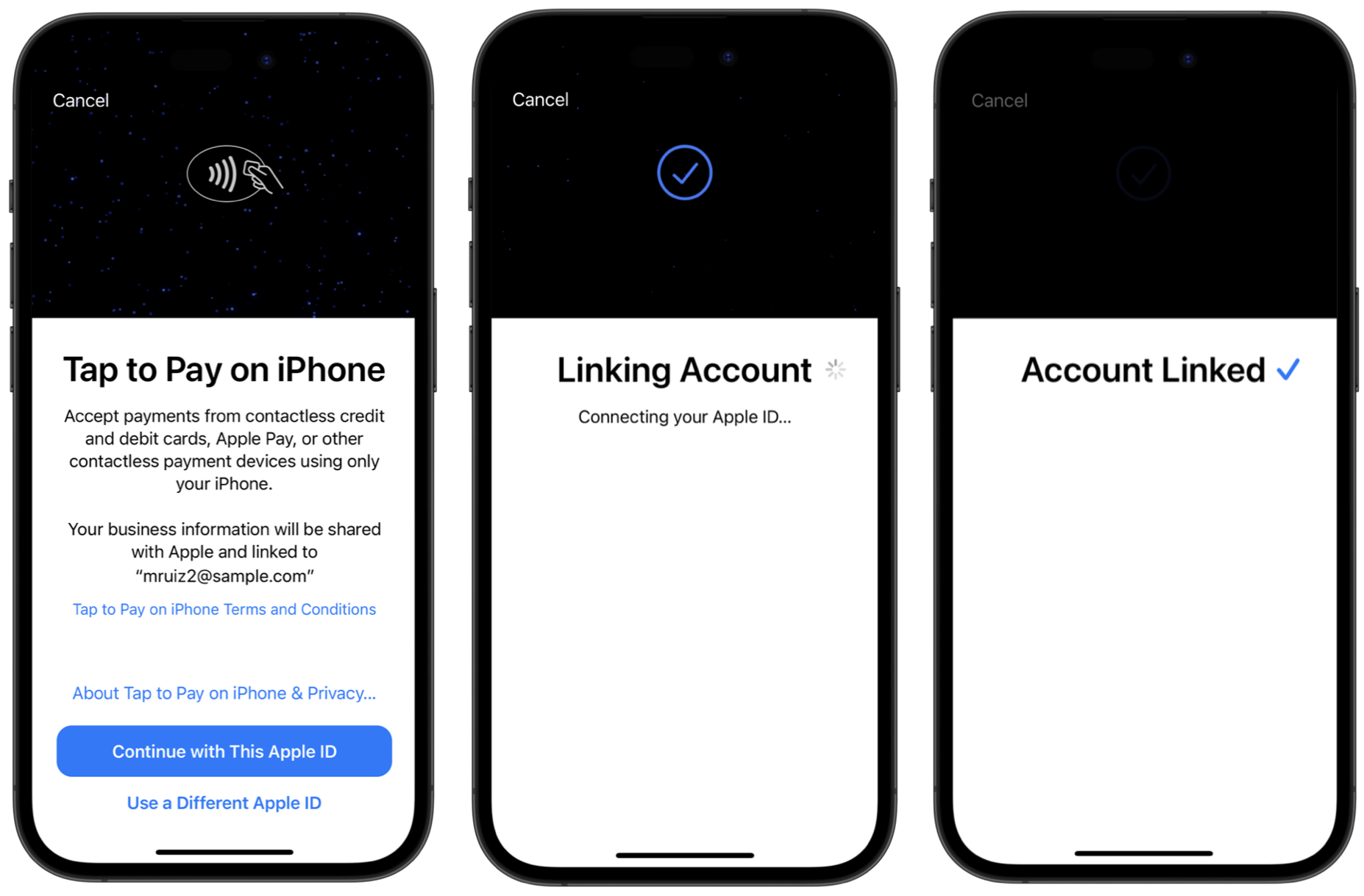
Link your Apple ID account to accept Tap to Pay payments
Learn more about account linking in the Tap to Pay on iPhone Business Information section of the Apple Tap to Pay on iPhone FAQ.
Handle unexpected disconnects 
Unexpected disconnects might occur between your app and the reader. For example, the Tap to Pay reader might unexpectedly disconnect because the device loses internet connectivity.
During testing, you can simulate an unexpected disconnect by disabling internet access to your device.
To handle the disconnect, you must implement the onDidReportUnexpectedReaderDisconnect
callback. This allows your app to reconnect to the Tap to Pay reader and, when appropriate, notify the user of what went wrong and how they can enable access to Tap to Pay. End users can resolve certain errors, such as internet connectivity issues.
const { discoverReaders, connectedReader, discoveredReaders } = useStripeTerminal({ onDidReportUnexpectedReaderDisconnect: (readers) => { // Consider displaying a UI to notify the user and start rediscovering readers }, });
Next steps
You’ve connected your application to the reader. Next, collect your first Stripe Terminal payment.
The BBPOS and Chipper™ name and logo are trademarks or registered trademarks of BBPOS Limited in the United States and/or other countries. The Verifone® name and logo are either trademarks or registered trademarks of Verifone in the United States and/or other countries. Use of the trademarks does not imply any endorsement by BBPOS or Verifone.