Query transactional data
Create custom reports for charges, refunds, transfers, and more.
Use the data in the tables within the schema for reporting on your account’s balance activity. The tables in the Payment Tables sections represent funds that flow between your customers and your Stripe account, such as charges or refunds. The Transfer Tables section has information about transfers of your Stripe account balance to your bank account (payouts).
Use the balance_
table as a starting point for accounting purposes. Unlike using separate tables (such as charges
or refunds
), it provides a ledger-style record of every type of transaction that comes into or flows out of your Stripe account balance. Use balance transactions to generate frequently used reports and to simplify how you report on financial activity. Some common types of balance transactions include:
charges
refunds
transfers
payouts
adjustments
application_
fees
Each balance transaction row represents an individual balance_transaction object that doesn’t change after it’s created. For example, creating a charge also creates a corresponding balance transaction of type charge
. Refunding this charge creates a separate balance transaction of type refund
—but it doesn’t modify the original balance transaction. Similarly, receiving a payout in your bank account (represented as a transfer) creates a balance transaction.
The following example query uses this table to retrieve some information about the five most recent balance transactions.
select date_format(created, '%m-%d-%Y') as day, id, amount, currency, source_id, type from balance_transactions order by day desc limit 5
day | id | amount | currency | source_id | type |
---|---|---|---|---|---|
txn_Nh7Ihbrh2FP8osN | -1,000 | usd | re_aD4v8IDQr0KcNDT | refund | |
txn_Qhf9GOwJWSQPSqD | 1,000 | usd | ch_UADoj4RAC83QlFo | charge | |
txn_0uyzyQUuAY9AYDk | 1,000 | usd | ch_VGciJAe2yoDAWgv | charge | |
txn_hqAlJv2FcDxuM0A | 1,000 | eur | ch_BsbZi1TLKtEBAH4 | charge | |
txn_BlBap9vubp6OXZJ | -1,000 | usd | re_XTSWRVtaqAJwQVE | refund |
You can calculate the most common financial summaries by joining the balance_
table with other tables containing the appropriate information. Some of our query templates (such as the daily balance and monthly summary and balance) work by joining this table to others.
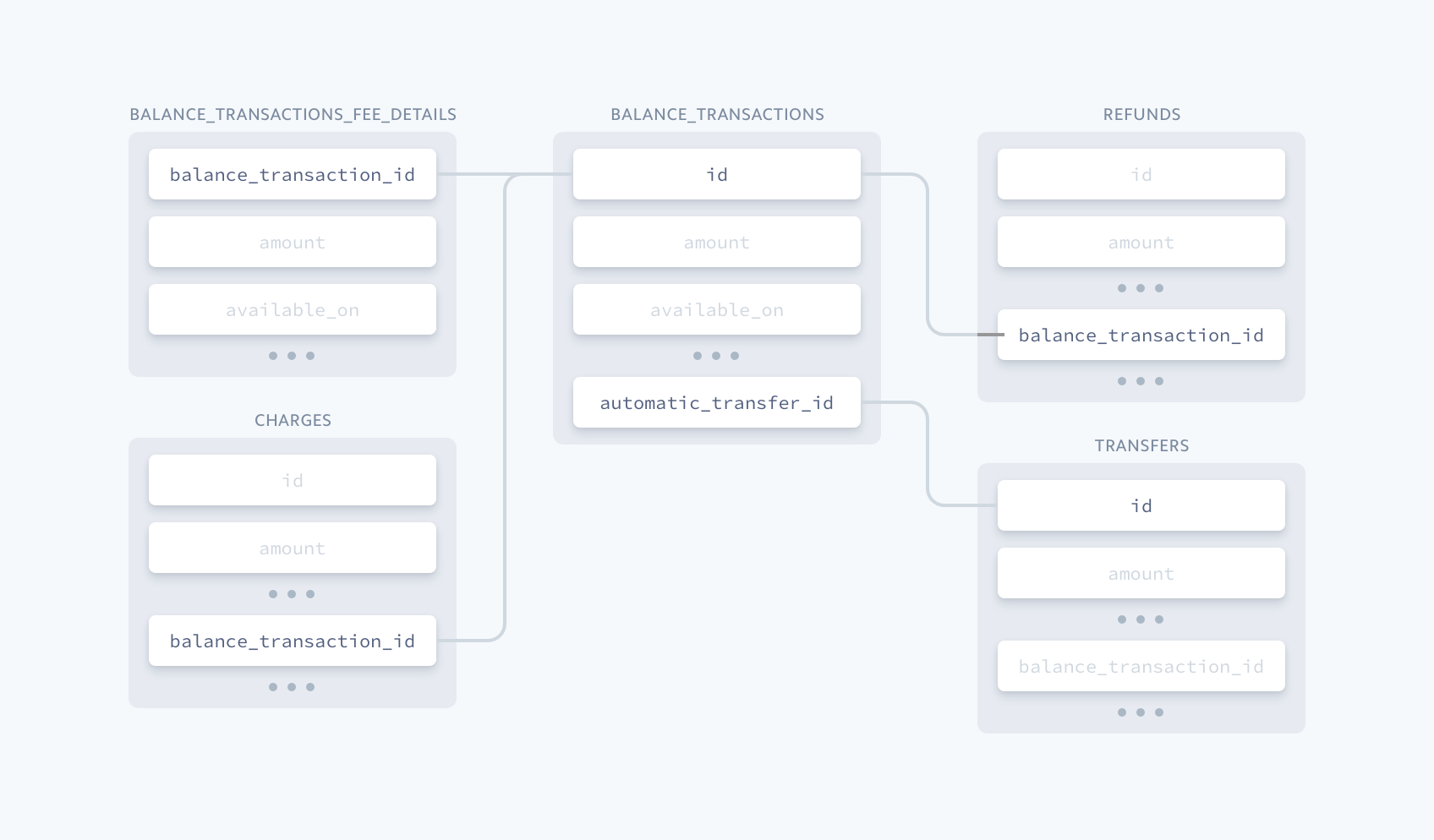
Balance transaction fee details
The balance_
table provides fee information about each individual balance transaction. Joining this table to balance_
in the manner below allows you to return fee information for each balance transaction.
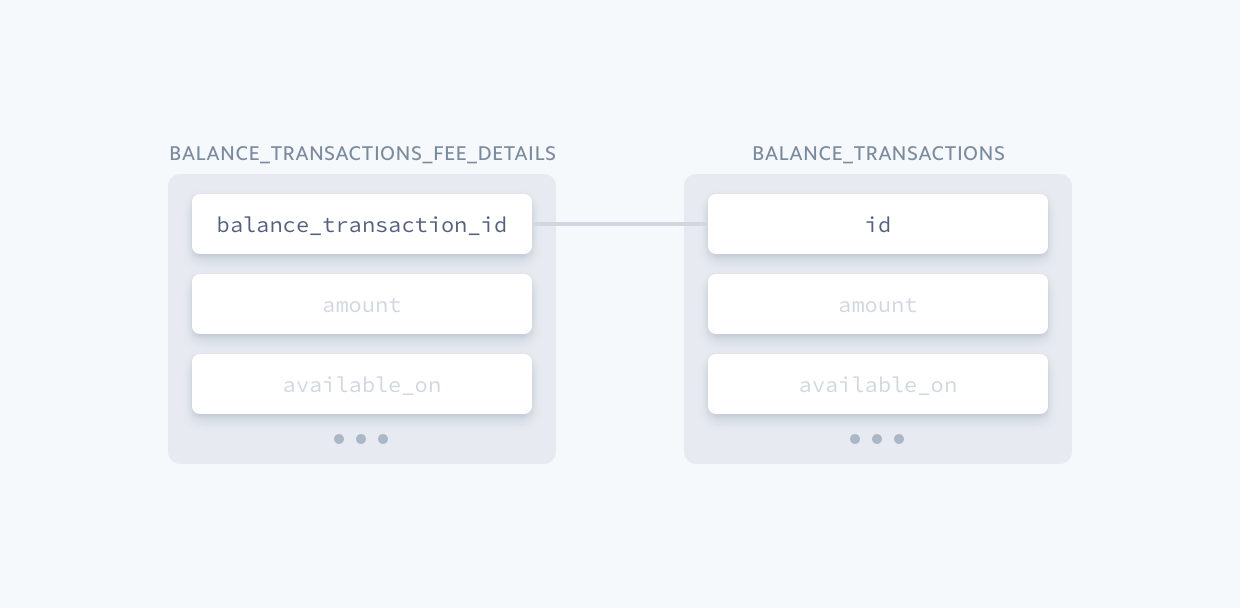
The following query joins the balance_
and balance_
tables together. Each balance transaction item returned includes the amount, fee, type of fee applied, and a description of the fee.
select date_format(date_trunc('day', balance_transactions.created), '%m-%d-%Y') as day, balance_transactions.id, balance_transactions.amount, balance_transactions.fee, balance_transaction_fee_details.type from balance_transactions inner join balance_transaction_fee_details on balance_transaction_fee_details.balance_transaction_id=balance_transactions.id order by day desc limit 5
day | id | amount | fee | type |
---|---|---|---|---|
txn_pCIJMH1ahmByG6o | 1,000 | 59 | stripe_fee | |
txn_qm6o6S5RpAHyNn6 | 1,000 | 59 | stripe_fee | |
txn_Eh7ouW8sPYI8qJG | 1,000 | 59 | stripe_fee | |
txn_fY4ikFUYr0lwgJG | 1,000 | 59 | stripe_fee | |
txn_psdKGV9Ad88jxvR | 1,000 | 59 | stripe_fee |
Charges
The charges
table contains data about Charge objects. Use this table for queries that focus on charge-specific information rather than for accounting or reconciliatory purposes. It also supplements accounting reports with additional customer data. For example, the payment card breakdown template query uses the charges
table to report on the different types of cards your customers have used.
You can join the charges
table to a number of others to retrieve more information with your queries.
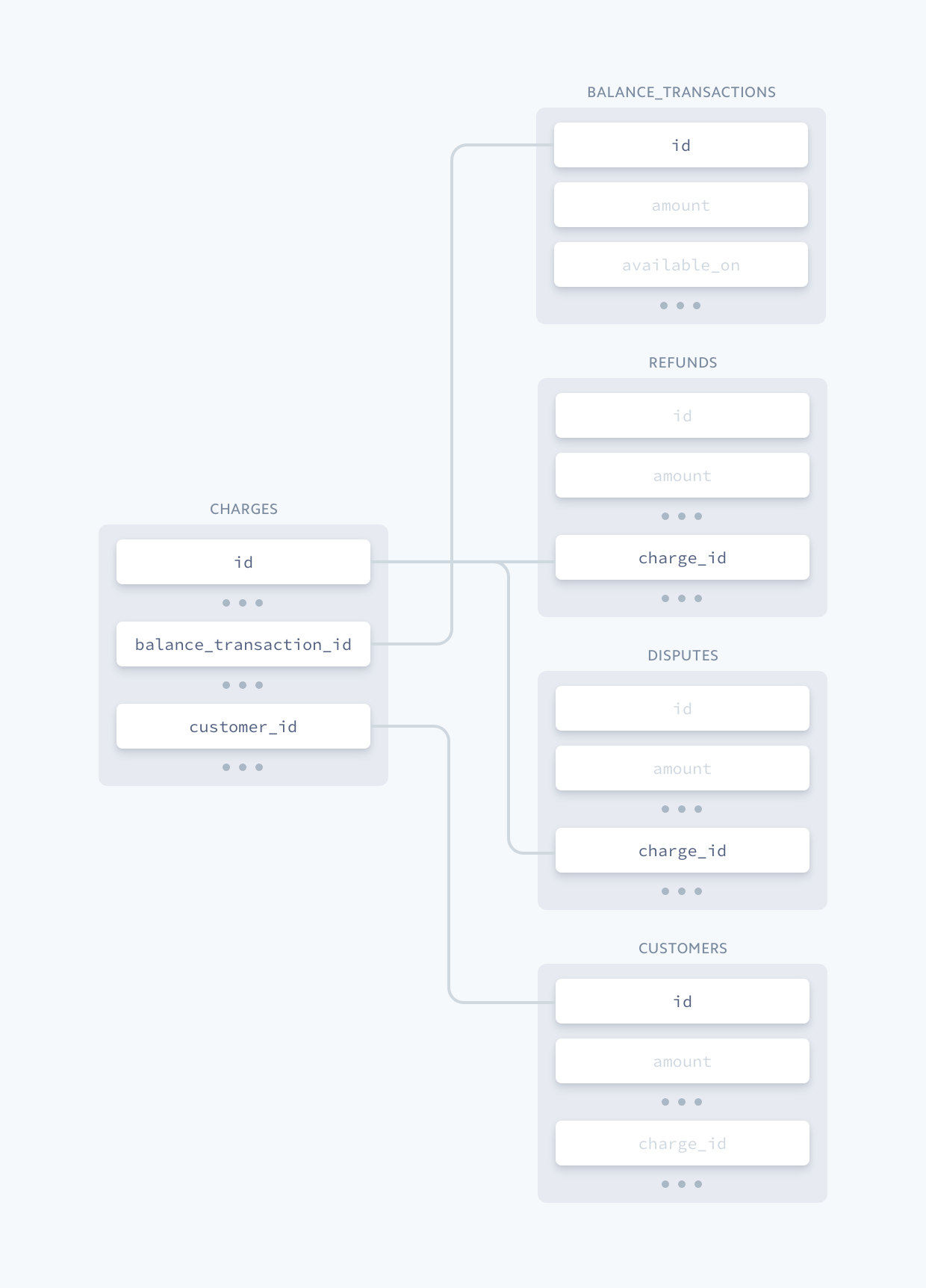
The following example uses the charges
table to report on failed charges, returning the card brand and a failure code and message.
select date_format(date_trunc('day', created), '%m-%d-%Y') as day, id, card_brand, failure_code, failure_message from charges where status = 'failed' order by day desc limit 5
day | id | card_brand | failure_code | failure_message |
---|---|---|---|---|
ch_suKYpbAWnpx6Xpk | Visa | card_declined | Your card was declined. | |
ch_e6yGhLXyAwuq8AH | MasterCard | card_declined | Your card doesn’t support this type of purchase. | |
ch_6szp0Ddw2vWXqqw | Visa | card_declined | Your card has insufficient funds. | |
ch_eCxpjiMsA07Nz0L | Visa | card_declined | Your card was declined. | |
ch_xUJhvSGBfszPDrZ | MasterCard | card_declined | Your card was declined. |
Customers
The customers
table contains data about Customer objects (this table isn’t part of the Payment Tables group). Use it if you’re creating charges using customers (for example, with saved payment information). It’s also useful if you’re using subscriptions.
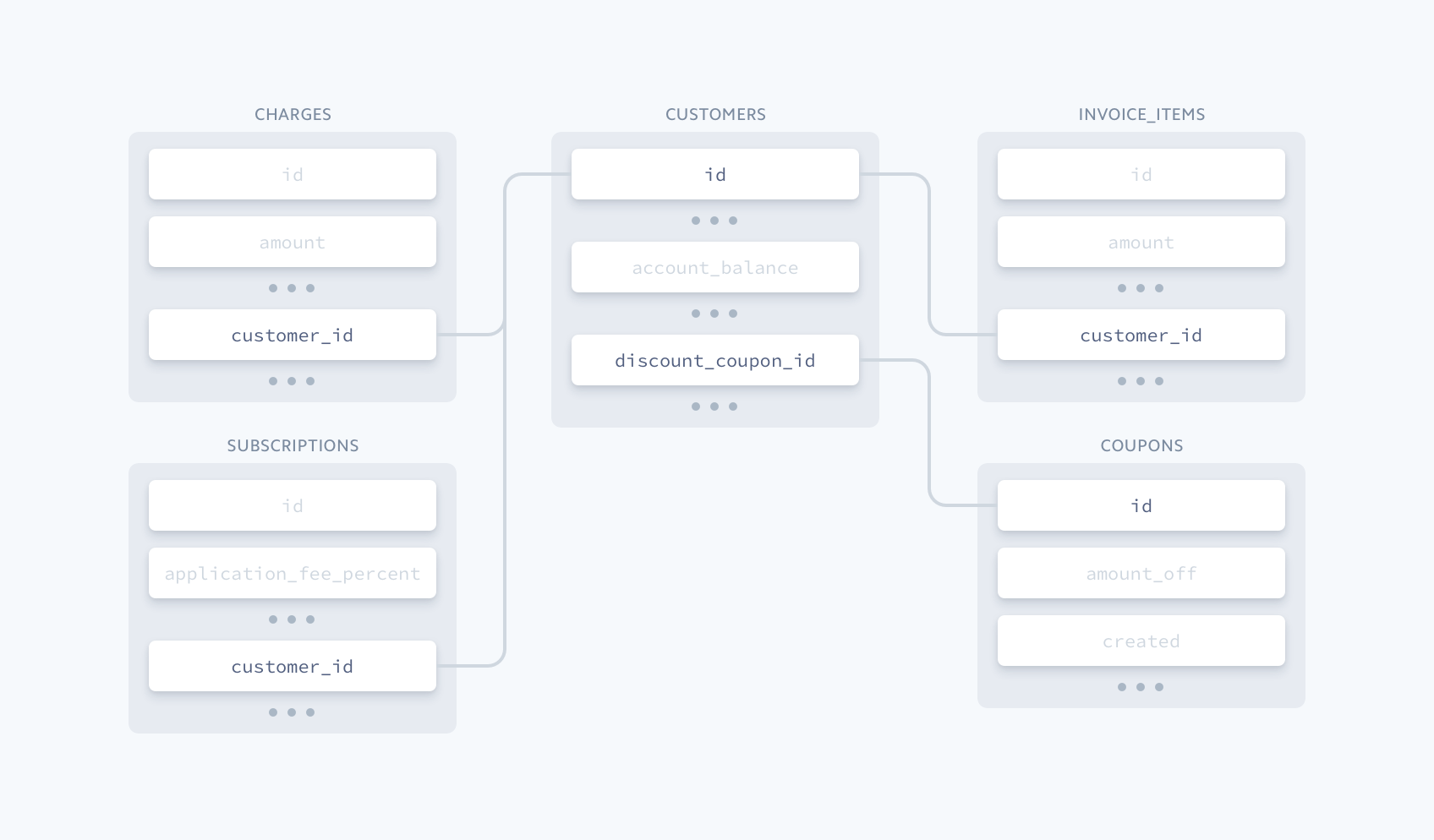
The following example retrieves a list of failed charges, with the ID and email address for each customer.
select date_format(date_trunc('day', charges.created), '%m-%d-%Y') as day, customers.id, customers.email, charges.id from charges inner join customers on customers.id=charges.customer_id where charges.status = 'failed' order by day desc limit 5
Refunds
Charges and refunds are separate objects within the API. Refunding a charge creates a Refund. This data is available in the refunds
table and provides in-depth information about completed refunds. Similar to reporting on charges, a best practice is to start with information about balance transactions. If necessary, you can then gather additional details using the refunds
table.
You can join the refunds
table to the balance_
and charges
tables to further explore refund data.
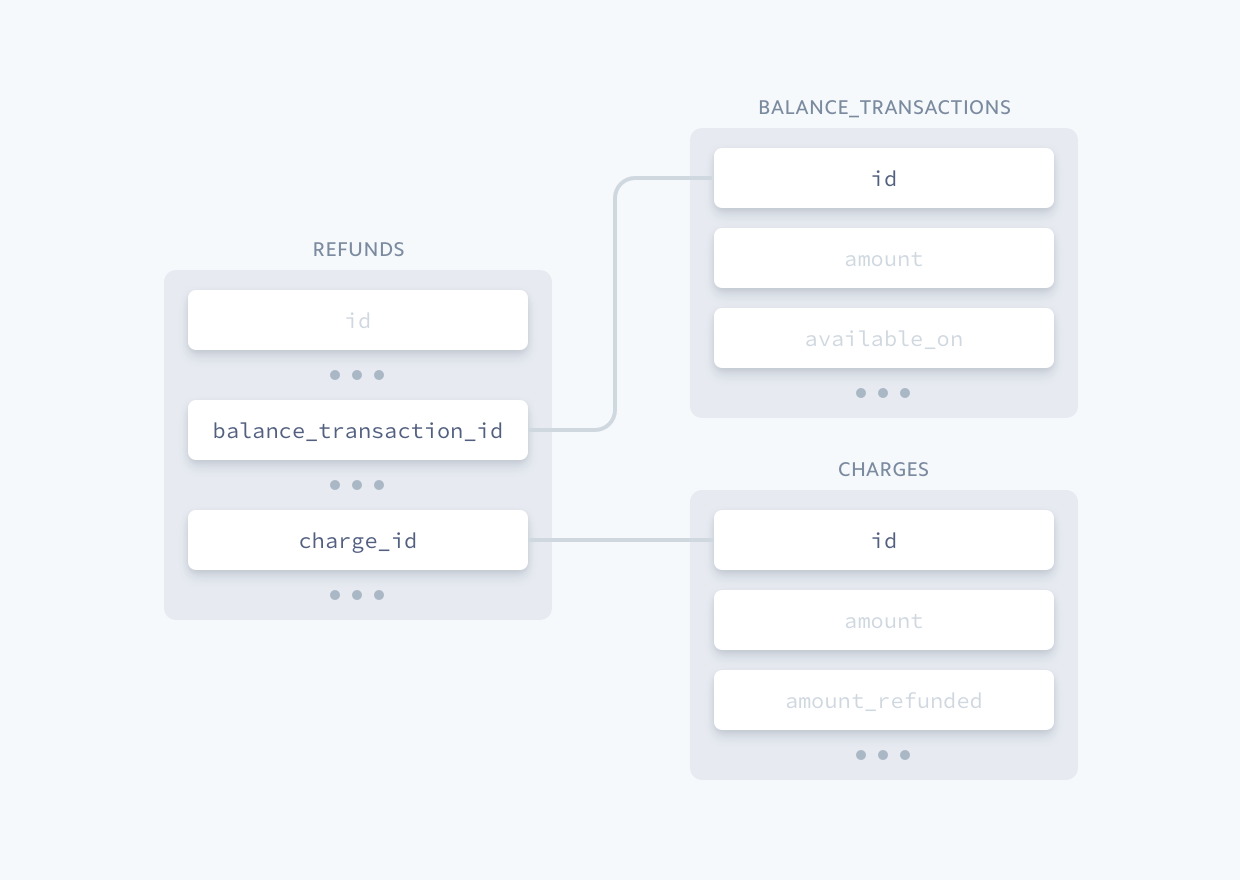
The following example joins the balance_
and refunds
tables together using the refunds.
and balance_
columns. Each balance transaction item returned is a refund, displaying the charge ID and amount. Only balance transactions created after a certain date are returned.
select date_format(date_trunc('day', balance_transactions.created), '%m-%d-%Y') as day, balance_transactions.source_id, refunds.charge_id, balance_transactions.amount from balance_transactions inner join refunds on refunds.balance_transaction_id=balance_transactions.id where balance_transactions.type = 'refund' order by day desc limit 5
day | source_id | charge_id | amount |
---|---|---|---|
re_w3WBmSUO6KvmcAn | ch_CuzIMEWRUygXItY | -1,000 | |
re_yNmiTVDbznw1Kbh | ch_sLA3gScmCqN1cQe | -1,000 | |
re_ZiImWgzsk23NYKR | ch_bs6vj2kvebefs3i | -1,000 | |
re_Hmn6xKKIV94PiLT | ch_vTickUdwFCAzKwo | -1,000 | |
re_S63tZmTeB5E0Kxg | ch_QEpQCHKO9yl3nSv | -1,000 |
Partial capture refunds
Using auth and capture and capturing only some of the authorized amount creates both a charge and a refund. An authorized charge creates a charge
and an associated balance transaction for the full amount. After a partial capture is complete, the uncaptured amount is released and a refund
is created with a reason
field of partial_
along with an associated balance transaction.
For example, authorizing a 10 USD charge but only capturing 7 USD creates a charge
for 10 USD. This also creates a refund
with the reason partial_
for the remaining 3 USD.
Take this into account if your business is performing auth and capture charges as you’re creating reports to review customer refund rates. Without consideration, auth and capture can misrepresent the number of refunds on your account. Use the refund’s reason
field to filter out partial capture refunds when retrieving payment information.
select balance_transactions.id, balance_transactions.amount from balance_transactions inner join refunds on refunds.id=balance_transactions.source_id where reason != 'partial_capture' limit 5
Transfers and payouts
The transfers
table contains data about payouts made from your Stripe balance to your bank account. You can use this table to reconcile each payout with the specific charges, refunds, and adjustments that comprise it, as long as you’re using automatic payouts.
For Connect platforms, this table also includes data about transfers of funds to connected Stripe accounts.
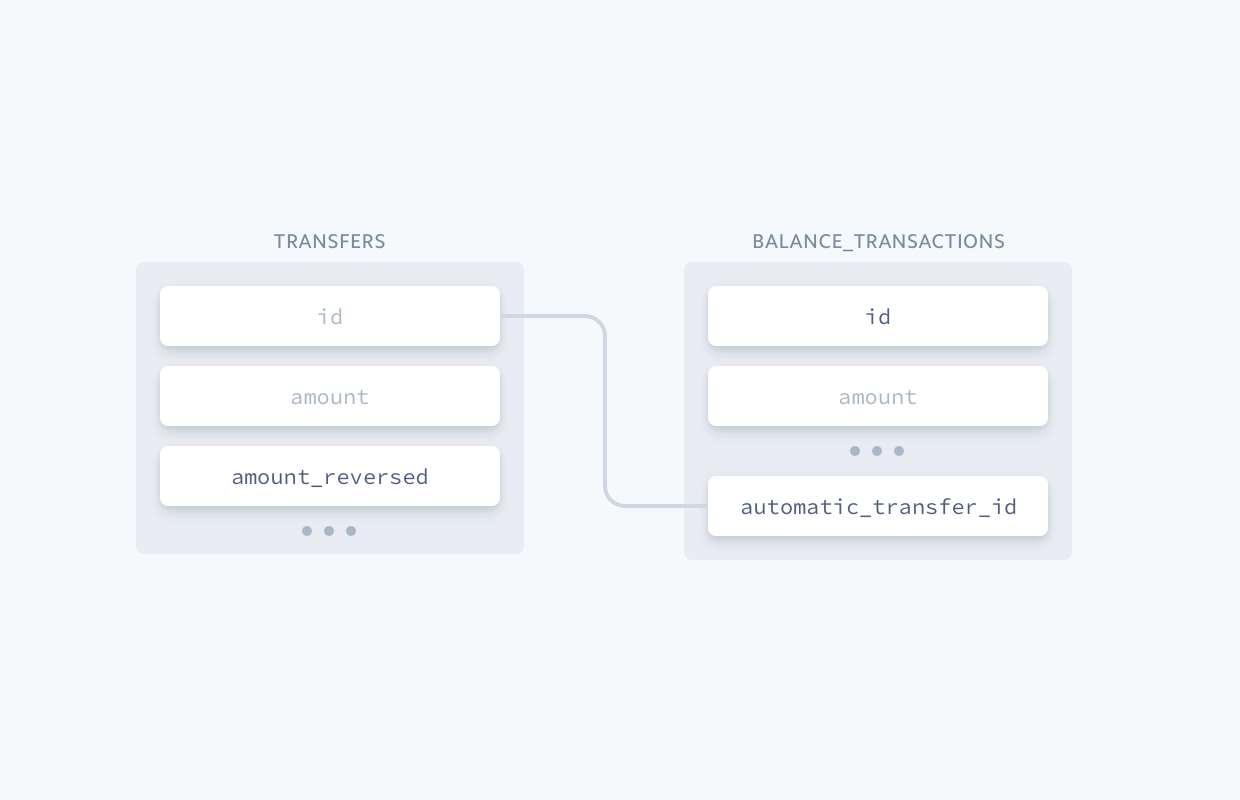
If you’re performing payouts manually, the amount in each payout to your bank account is arbitrary. As such, you can’t reconcile it to specific balance transactions and it only reflects the amount you requested to pay out to your bank account.
The following example joins the balance_
and transfers
tables together. It returns a list of charges and refunds, the payout they relate to, and the date that the payout is scheduled to arrive into your bank account.
select date_format(date_trunc('day', balance_transactions.created), '%m-%d-%Y') as bt_created, balance_transactions.source_id, balance_transactions.type, balance_transactions.net as net_amount, balance_transactions.automatic_transfer_id as transfer_id, date_format(date_trunc('day', transfers.date), '%m-%d-%Y') as transfer_date from balance_transactions inner join transfers on balance_transactions.automatic_transfer_id=transfers.id where balance_transactions.type = 'charge' and balance_transactions.type != 'refund' order by bt_created desc limit 5
day | source_id | type | net_amount | transfer_id | transfer_date |
---|---|---|---|---|---|
05-22-2017 | ch_trNCTecI6eSJOFO | charge | 941 | po_RJ6TMOc33QlLign | 05-24-2017 |
05-22-2017 | ch_2SuH9BdjNhvstXq | charge | 941 | po_TnYi6S7WOxERLO2 | 05-24-2017 |
05-21-2017 | ch_fBirJP89YWW31Gs | charge | 941 | po_HWp3exvDbVZ5M97 | 05-23-2017 |
05-21-2017 | ch_QKvC0P0vdqS78YO | charge | 941 | po_ctW2gpVpWhHdOwX | 05-23-2017 |
05-21-2017 | ch_8DyVgd2LhgVfqnB | charge | 941 | po_4R8Amh175xNrZsi | 05-23-2017 |
Caution
Payouts before 04-06-2017 have a TRANSFER_ID with a tr_
prefix.
Transfer reversals
You can reverse a manually created payout (or transfer to a connected Stripe account) if it hasn’t been paid out yet by using funds returned to the available balance in your account. These are represented as Transfer_reversal objects and reside in the transfer_
table.
Transfer reversals only apply to payouts and transfers that have been created manually—you can’t reverse automatic payouts.