Add custom payment methods
Add custom payment methods to the Payment Element.
The Stripe Payment Element lets your users pay with many payment methods through a single integration. Use custom payment methods if you need to display additional payment methods that aren’t processed through Stripe. If you use custom payment methods, you can optionally record purchases processed outside of Stripe to your Stripe account for reporting purposes.
To configure a custom payment method, create it in your Stripe Dashboard, and provide a display name and icon that the Payment Element also displays. The Stripe Dashboard also provides access to over 50 preset custom payment methods. After you create the payment method, follow the guide below to configure the Payment Element. Setting up the Payment Element requires some additional configuration work because custom payment method transactions process and finalize outside of Stripe.
Note
When integrating with a third party payment processor, you’re responsible for complying with applicable legal requirements, including your agreement with your PSP, applicable laws, and so on.
This guide adds a custom payment method using the HTML or JS example from the Collect payment details before creating an Intent guide.
Before you begin 
- Create a Stripe account or sign in.
- Follow the Collect payment details before creating an Intent guide to complete a payments integration.
- Then, for each custom payment method you want to provide, follow the steps below.
Create your custom payment method in the DashboardDashboard
Go to Settings > Payments > Custom Payment Methods to get to the custom payment methods page. Create a new custom payment method and provide the display name and logo that the Payment Element displays.
Choose the right logo 
- If you provide a logo with a transparent background, consider the background color of the Payment Element on your page and make sure that it stands out.
- If you provide a logo with a background fill, we won’t provide rounded corners—include these in your file.
- Choose a logo variant that can scale down to 16 pixels x 16 pixels. This is often the standalone logo mark for a brand.
After creating the custom payment method, the Dashboard displays the custom payment method ID (beginning with cpmt_
) needed in step 2.
Add the custom payment method type to your Stripe elements configClient-side
In your checkout.
file where you initialize Stripe Elements, specify the customPaymentMethods to add to the Payment Element. In addition to providing the ID from step 1 (it begins with cpmt_
), provide the options.
and optional subtitle
.
const elements = stripe.elements({ // ... customPaymentMethods: [ { id:
, options: { type: 'static', subtitle: 'Optional subtitle', } } ] });'{{CUSTOM_PAYMENT_METHOD_TYPE_ID}}'
When the Payment Element loads, it now shows your custom payment method.
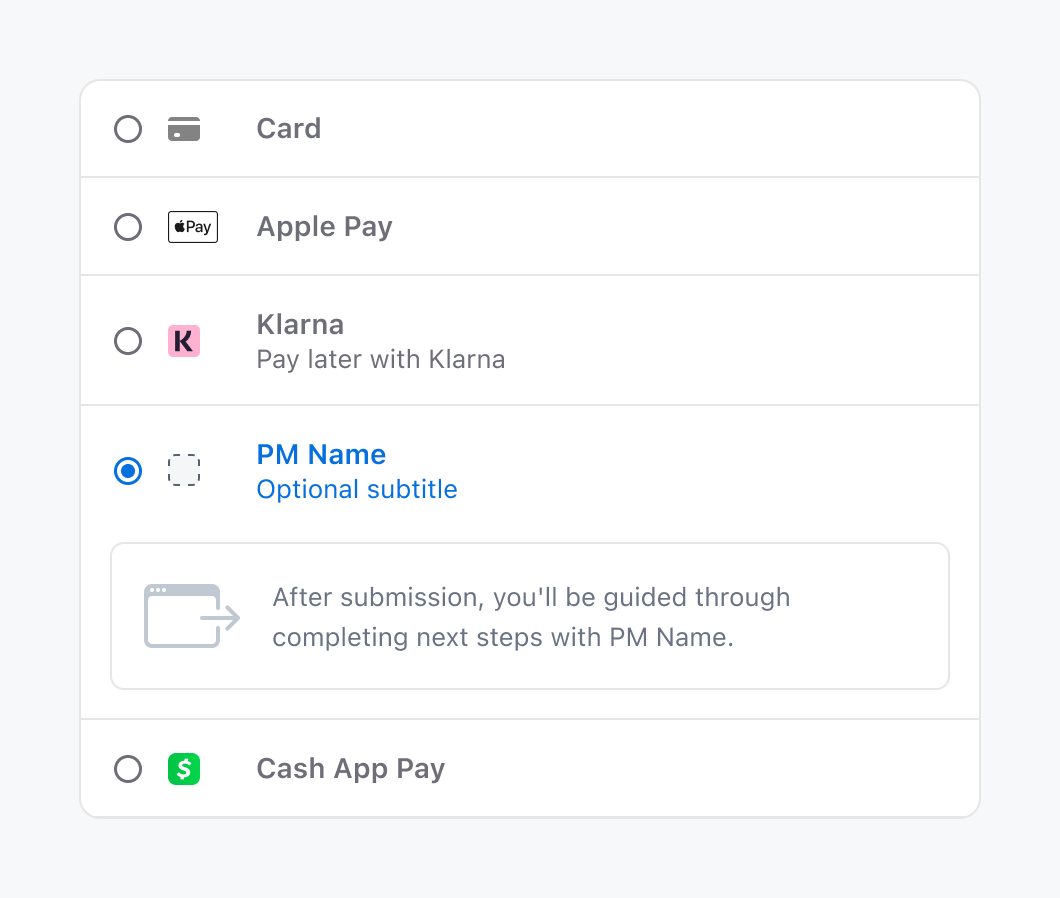
Handle payment method submissionClient-side
Update the handleSubmit
function that’s called when users click the pay button on your website so that custom payment method transactions are processed outside of Stripe.
The elements.submit() function retrieves the currently selected payment method type. If the selected payment method is your custom payment method, process the transaction accordingly. For example, you might show a modal and then process the payment on your own server, or redirect your customer to an external payment page.
async function handleSubmit(e) { const { submitError, selectedPaymentMethod } = await elements.submit(); if (selectedPaymentMethod ===
) { // Process CPM payment on merchant server and handle redirect const res = await fetch("/process-cpm-payment", { method: 'post' }); ... } else { // Process Stripe payment methods ... } }'{{CUSTOM_PAYMENT_METHOD_TYPE_ID}}'