Collecter les adresses physiques et les numéros de téléphone
Découvrez comment collecter des adresses et des numéros de téléphone dans votre application mobile.
Pour collecter des adresses complètes à des fins de facturation ou de livraison, utilisez l’Address Element.
Vous pouvez également utiliser l’Address Element pour :
- Collecter les numéros de téléphone des clients
- Utiliser l’autocomplétion (activée par défaut sous iOS)
- Préremplir les informations de facturation dans le Payment Element en transmettant une adresse de livraison
Stripe combine les informations recueillies sur l’adresse et le moyen de paiement pour créer un PaymentIntent.
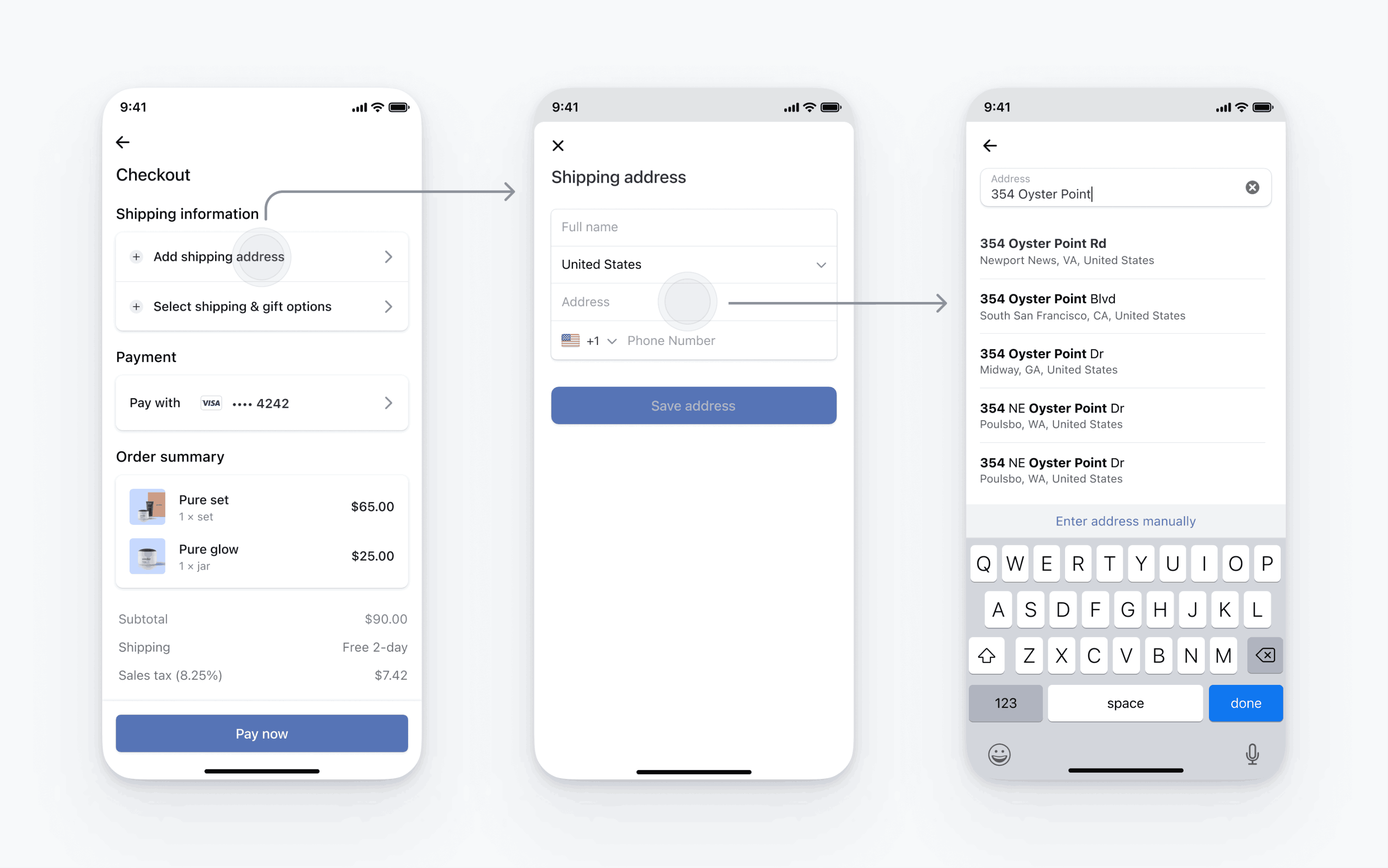
Configurer StripeCôté serveurCôté client
Pour commencer, vous devez créer un compte Stripe. Inscrivez-vous maintenant.
Le SDK iOS de Stripe est disponible en open source et fait l’objet d’une documentation complète. Il est également compatible avec les applications prenant en charge iOS 13 et les versions ultérieures.
Remarque
Pour obtenir de plus amples informations sur la version la plus récente du SDK et ses versions antérieures, consultez la page des versions sur GitHub. Pour recevoir une notification lors de la publication d’une nouvelle version, surveillez les versions à partir du référentiel.
Configurez le SDK avec votre clé publiable Stripe au démarrage de votre application. Cela lui permet d’envoyer des requêtes à l’API Stripe.
Remarque
Utilisez vos clés de test lors de vos activités de test et de développement et vos clés du mode production pour la publication de votre application.
Configurer des suggestions de remplissage automatique de l'adresse
L’autocomplétion est activée par défaut sous iOS.
Configurer l'Address Element
Vous pouvez configurer l’Address Element en ajoutant des détails tels que l’affichage des valeurs par défaut, la définition des pays autorisés, la personnalisation de l’apparence, etc. Reportez-vous à AddressViewController.Configuration pour consulter la liste complète des options de configuration.
let addressConfiguration = AddressViewController.Configuration( additionalFields: .init(phone: .required), allowedCountries: ["US", "CA", "GB"], title: "Shipping Address" )
Récupérer les informations de l'adresse
Récupérez les détails de l’adresse en vous conformant à AddressViewControllerDelegate
, puis en utilisant addressViewControllerDidFinish pour fermer le contrôleur d’affichage. La valeur de l’adresse est une adresse valide ou nil.
extension MyViewController: AddressViewControllerDelegate { func addressViewControllerDidFinish(_ addressViewController: AddressViewController, with address: AddressViewController.AddressDetails?) { addressViewController.dismiss(animated: true) self.addressDetails = address } }
Présenter l'Address Element
Créez un AddressViewController à l’aide de la configuration d’adresse et déléguez depuis les étapes précédentes. Vous pouvez le présenter dans un contrôleur de navigation ou le pousser sur un contrôleur de navigation.
self.addressViewController = AddressViewController(configuration: addressConfiguration, delegate: self) let navigationController = UINavigationController(rootViewController: addressViewController) present(navigationController, animated: true)