Accept a payment
Securely accept payments online.
Build a payment form or use a prebuilt checkout page to start accepting online payments.
Embed a prebuilt payment form on your site using Stripe Checkout. See how this integration compares to Stripe’s other integration types.
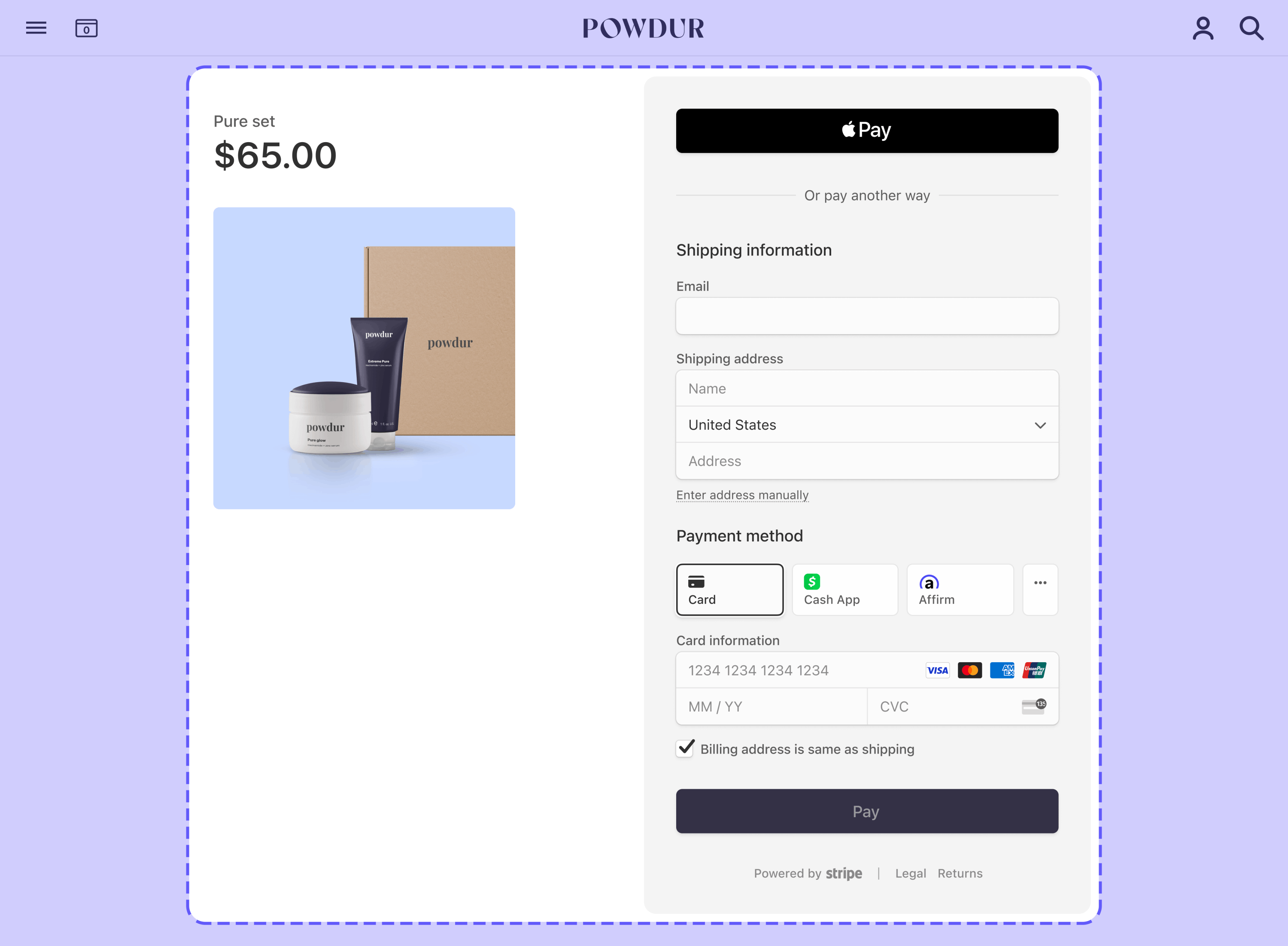
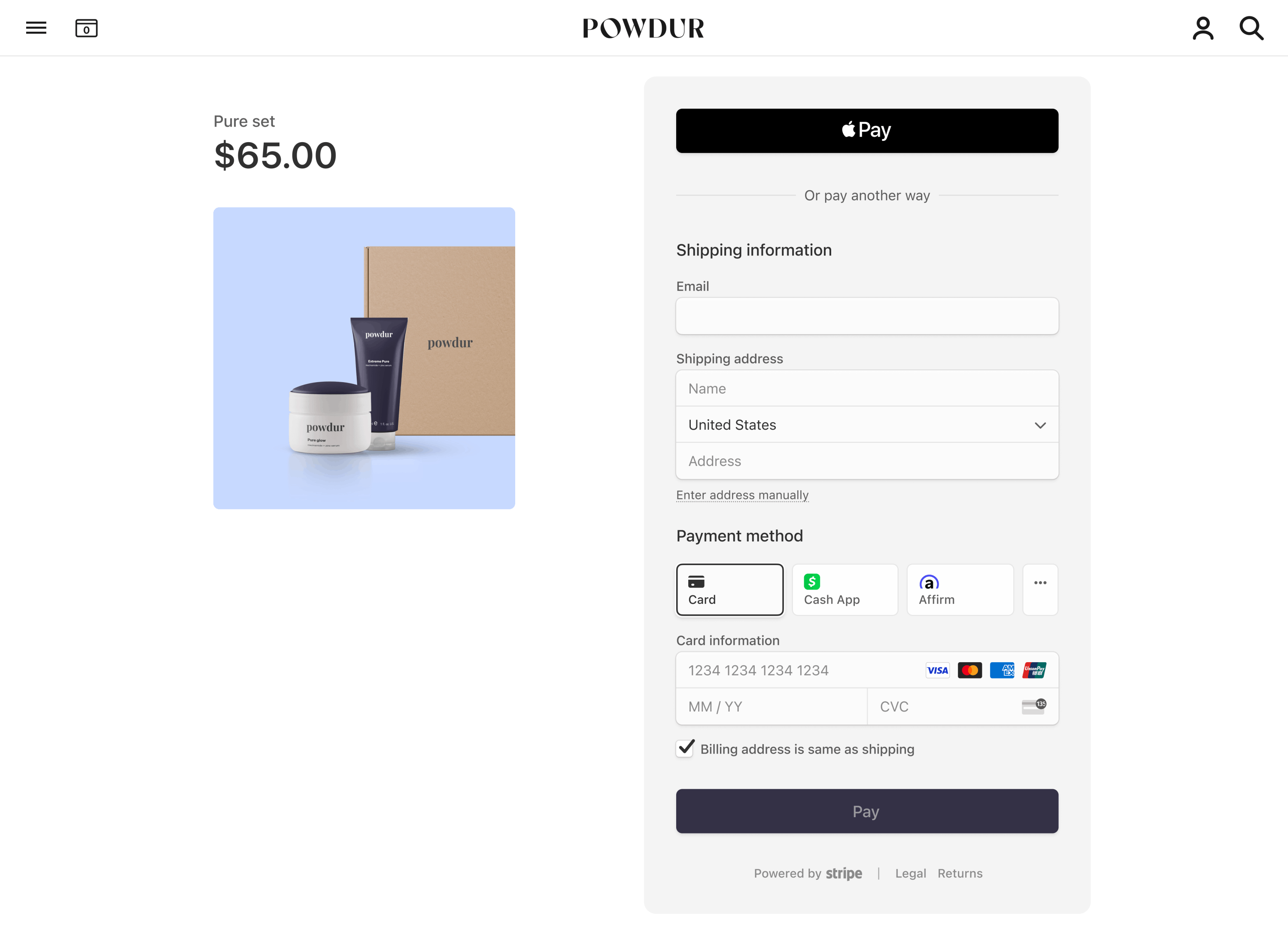
Integration effort
Integration type
Embed prebuilt payment form on your site
UI customization
Use the branding settings in the Stripe Dashboard to match Checkout to your site design.
Set up StripeServer-side
First, register for a Stripe account.
Use our official libraries to access the Stripe API from your application:
Create a Checkout SessionServer-side
From your server, create a Checkout Session and set the ui_mode to embedded
. You can configure the Checkout Session with line items to include and options such as currency.
To return customers to a custom page that you host on your website, specify that page’s URL in the return_url parameter. Include the {CHECKOUT_
template variable in the URL to retrieve the session’s status on the return page. Checkout automatically substitutes the variable with the Checkout Session ID before redirecting.
Read more about configuring the return page and other options for customizing redirect behavior.
After you create the Checkout Session, use the client_
returned in the response to mount Checkout.
Mount CheckoutClient-side
Checkout renders in an iframe that securely sends payment information to Stripe over an HTTPS connection.
Common mistake
Avoid placing Checkout within another iframe because some payment methods require redirecting to another page for payment confirmation.
Customize appearance
Customize Checkout to match the design of your site by setting the background color, button color, border radius, and fonts in your account’s branding settings.
By default, Checkout renders with no external padding or margin. We recommend using a container element such as a div to apply your desired margin (for example, 16px on all sides).
Show a return page
After your customer attempts payment, Stripe redirects them to a return page that you host on your site. When you created the Checkout Session, you specified the URL of the return page in the return_url parameter. Read more about other options for customizing redirect behavior.
When rendering your return page, retrieve the Checkout Session status using the Checkout Session ID in the URL. Handle the result according to the session status as follows:
complete
: The payment succeeded. Use the information from the Checkout Session to render a success page.open
: The payment failed or was canceled. Remount Checkout so that your customer can try again.
const session = await fetch(`/session_status?session_id=${session_id}`) if (session.status == 'open') { // Remount embedded Checkout } else if (session.status == 'complete') { // Show success page // Optionally use session.payment_status or session.customer_email // to customize the success page }
Redirect-based payment methods
During payment, some payment methods redirect the customer to an intermediate page, such as a bank authorization page. When they complete that page, Stripe redirects them to your return page.
Learn more about redirect-based payment methods and redirect behavior.
Test your integration
To test your embedded payment form integration:
- Create an embedded Checkout Session and mount Checkout on your page.
- Fill out the payment details with a method from the table below.
- Enter any future date for card expiry.
- Enter any 3-digit number for CVC.
- Enter any billing postal code.
- Click Pay. You’re redirected to your
return_
.url - Go to the Dashboard and look for the payment on the payments page. If your payment succeeded, you’ll see it in that list.
- Click your payment to see more details, like a Checkout summary with billing information and the list of purchased items. You can use this information to fulfill the order.
Learn more about testing your integration.
See Testing for additional information to test your integration.