Request a payment data import
Securely import sensitive payment data.
Stripe lets you retain your existing customer and payment data when you migrate to Stripe. We work with your team and current payment provider, as needed, to securely migrate your information in a few steps:
- Build your Stripe integration.
- Request and confirm the migration details.
- Update your integration to complete the migration.
- (Optional) Migrate subscriptions.
This process allows you to accept and charge new customers on Stripe and continue charging your existing customers with your current processor until the migration is complete. Your customers incur no downtime. After the migration process completes, you can process all payments on Stripe.
Build and test your Stripe integration before requesting data from your current processor. This gives you plenty of time to verify and test your new integration. If you have any questions about the migration process or integrating with Stripe, let us know.
Build your Stripe integration
Stripe simplifies your security requirements so that your customers don’t have to leave your site to complete a payment. This is done through a combination of client-side and server-side steps:
- From your website running in the customer’s browser, Stripe securely collects their payment details.
- Stripe responds with a representative token.
- The browser submits the token to your server, along with any other form data.
- Your server-side code uses that token in an API request (for example, when creating a charge).
This approach streamlines your website’s checkout flow, while sensitive payment information never touches your server. This allows you to operate in accordance with PCI-compliance regulations, which can save you time and provide financial benefits.
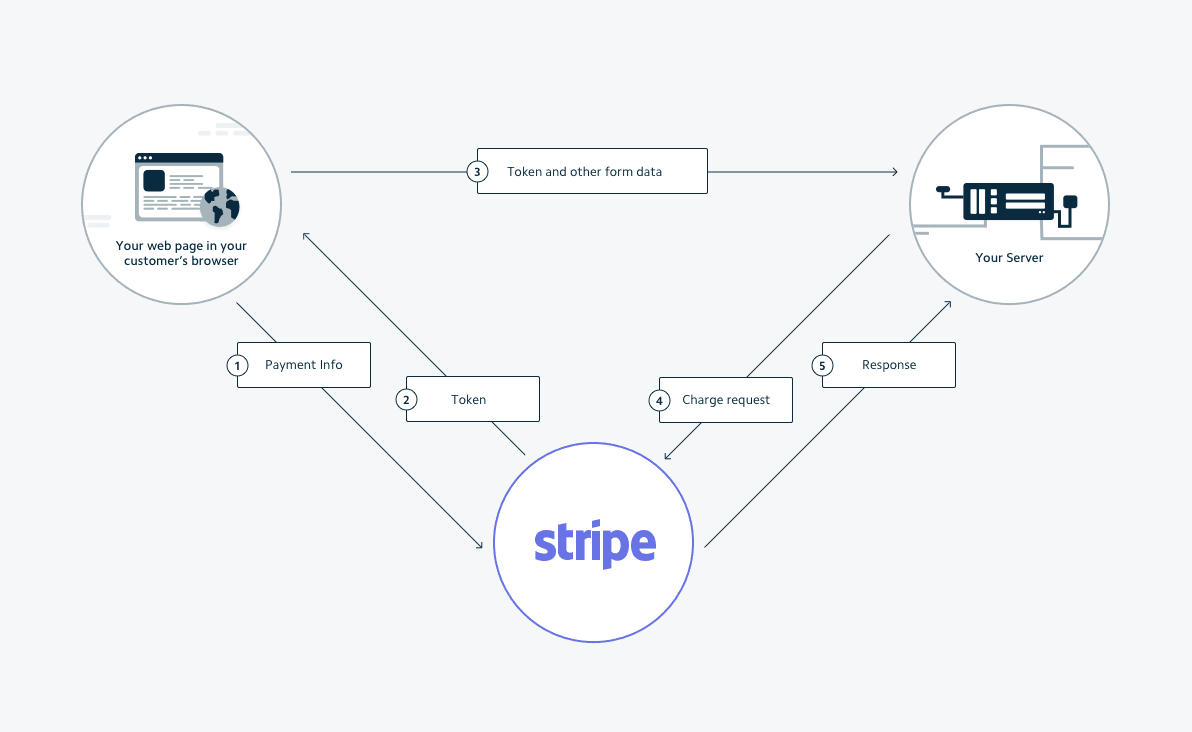
Stripe’s payment process flow
Compared to other payment processors, a Stripe integration can differ in the following ways:
- Your customer never leaves your website.
- Token creation isn’t tied to a specific product or amount.
- There’s no need to create a client-side key on-demand. You use a set, publishable API key instead.
Prepare your integration
For all new customer tokens (not imported), implement the following:
- Use Customer objects to save the card information.
- Collect and tokenise customer card information with one of our recommended payments integrations.
- Create charges for these new customers.
Using this approach, you can accept payments from your new customers on Stripe without affecting your current customers in your existing processor during the migration process.
Integration considerations
Designing your integration before you ask your payment processor to transfer data to Stripe is the most efficient way to handle imported data. Some actions you can take before requesting an import include:
- Complete your Stripe account setup.
- Remap customer records.
- Handle updates to payment information during the migration.
- Enable all optimisations, such as Adaptive Acceptance, Card Account Updater (CAU), and network tokens.
Remap customer records
If you prefer, you can configure your integration to import the payment method data from previous records into existing Stripe customer objects. Doing so prevents the migration from creating a new (possibly duplicate) customer in your Stripe account for each unique customer ID in the files we receive from your prior processor.
After migrating, you might still have to update some records to correspond with the new Stripe Customer identifier, if:
- You created the Stripe customer before migration, then we imported the payment information to update this customer record.
- We imported the payment information as a new customer record.
For example, customer jenny.rosen@example.com might have ID 42
in your database, corresponding to ID 1893
in your previous processor’s system, but is ID cus_
in your Stripe account. In this case, you must now map your ID 42
to the Stripe ID cus_
in your database. Stripe provides a post-import mapping file to help you identify required remapping.
Handle updates to payment information
If customers update their payment information with your previous processor in the window between transferring the data and completing the import, those changes are lost.
Update your site’s process for handling updates to saved payments to prevent errors or billing issues for your customers. This includes preparations to perform a self-migration for any customer without a stored Stripe customer ID:
- Create a new Customer object in Stripe for your customer.
- Attach the payment method to the Customer object.
- If necessary, migrate subscriptions.
After migration completes, Stripe automatically handles card-triggered updates, such as expiry date changes.
Request and confirm the migration details
- After you complete your integration and are ready to process payments on Stripe, request your payment data from your previous processor. Many processors require the account owner to request a data transfer.
- Log in to your Stripe account to submit the migration request form to request your import migration.
- Engage with Stripe through the authenticated email thread we create upon receipt of your migration request.
Warning
Never send sensitive credit card details or customer information directly to Stripe. If you have this data, let us know in your migration request form so we can help you securely transfer your data.
Stripe can import your customer billing address information and payment details. For details on migrating specific payment types, see:
Data migrations doesn’t migrate subscriptions, but you can recreate them separately or import them using the Billing Migration Toolkit.
Your previous processor might take a few days or several weeks to transfer the final data to Stripe. Allow for this transition time in your migration plan.
After your previous processor transfers your data, Stripe reviews the data and identifies any problems with the import. We work with you and your previous processor to correct any issues. We then share a summary of the import for your final review and approval.
After your approval, Stripe imports the data into your account. We create a Customer for each unique customer in the transferred data file, and create and attach the customer’s cards as Card or Payment Method objects. If the transferred data specifies the customer’s default card, we set that as the customer’s default payment method for charges and subscription payments.
If your Stripe account has accumulated significant customer records by the time you migrate, consider mapping import date into existing Stripe customer objects instead of creating new Customer objects.
Stripe typically imports data within 10 business days of receiving the correct data from your previous processor, along with any supplementary data files you want to share with our team.
Update your integration
After completing the import, Stripe sends you a choice of a CSV or JSON file that shows the mapped relationship between your current processor’s IDs and the imported Stripe object IDs. Parse this mapping file and update your database accordingly. Make sure your integration handled any card updates that took place during the transition.
Post import mapping file
After you update your integration with this mapping file, you can begin charging all of your customers on Stripe.
{ "1893": { "cards": { "2600": { "id": "card_2222222222", "fingerprint": "x9yW1WE4nLvl6zjg", "last4": "4242", "exp_month": 1, "exp_year": 2020, "brand": "Visa" }, "3520": { "id": "card_3333333333", "fingerprint": "nZnMWbJBurX3VHIN", "last4": "0341", "exp_month": 6, "exp_year": 2021, "brand": "Mastercard" } }, "id": "cus_abc123def456" } }
The example JSON mapping above shows:
- Imported customer ID 1893 as a new Stripe Customer with ID
cus_
.abc123def456 - Imported customer card ID 2600 as a new Stripe Card with ID
card_
.2222222222 - Imported customer card ID 3520 as a new Stripe Card with ID
card_
.3333333333
Stripe can import card data as PaymentMethods instead of Card objects if you specify it in your migration request. The following examples show the mapping files for different types of payment information imports.
Post import payment declines
After migrating, monitor your payments performance to make sure the acceptance rate for imported payment data matches your expectations.
Payment acceptance (or issuer authorisation rate) is the percentage of transactions that issuers successfully authorise out of all transactions submitted for payment. This metric excludes blocked transactions (for example due to Radar rules) because those are never submitted for authorisation.
In both your general approach and post migration, align your payment authorisation optimisation goals with your business objectives. For example, a digital goods business with low unit cost might set their risk level to block fewer payments. Consider the potential effects:
- Increased conversion rates due to less friction.
- Increased exposure to fraud due to riskier payments getting through.
- Lower raw issuer authorisation rates due to fraud model blocks by the issuer.
Make sure you provide accurate data (such as cardholder name, billing address, and email). Reflecting the cardholder’s intent maximizes successful authorisation potential.
Identify cards on file
Payment data migrations involve cards on file (cards saved for a future merchant-initiated or off session payment for the same customer). Make sure you store imported payment data and label payments using those cards on file with the correct off_
parameter. If you improperly identify cards on file:
- Issuers who can’t confirm a cardholder’s consent to future or recurring payments might decline them.
- The payment data might be ineligible for certain Stripe optimisation products such as Card account updater (CAU) and Network tokens (NT).
Monitor decline reasons for optimisation opportunities
Following your migration, your issuer decline reasons can help you identify whether migrated payment data is transacting as expected. Spikes in certain types of declines might benefit from the following optimisation products:
- Card account updater: Stripe’s partnerships with card networks allows us to automatically obtain updates for expired or replaced cards in both real-time and the background.
- Automatic retries (Dunning): Use caution because retrying numerous cards (such as after a migration) can appear suspicious to issuers. If you use Stripe’s Smart retries for your billing payments, the machine learning model analyses decline code, payment method updates, and bank risk threshold activity to retry recurring revenue payments more strategically.
- Network tokens: Replace a specific payment account number (PAN) with a secure token from the card network to make sure PAN updates (like renewal or replacement) automatically reflect in the token.
- Adaptive acceptance: Stripe uses machine learning to assess the effect of minor adjustments (such as formatting) to an authorisation request in real-time, then refines the payment retry before returning the original decline to the customer.
- Customer outreach: Asking your customer to log in and re-enter or re-verify their payment details often re-establishes your business’s trustworthiness with the customer and the payment providers. Consider notifying customers through channels other than email, such as text messages or in-app notifications.
The following table shows which optimisation products offer improvement for a variety of decline reasons.
Decline codes might include | Migration effect | Do | Don’t |
---|---|---|---|
| Updates to card data during the natural migration lag can cause saved card data to be out of date. |
| Retry |
| Changes to your statement descriptor or other identification markers might trigger issuer risk models or confuse your customer. |
| Card account updater |
| Some migrated payment data might be missing initial card validation details, such as the network token or original transaction ID. |
| Network tokens |
| Customers might report lost or stolen cards during a migration lag. Look out for a special CONTAC event in conjunction with these declines. |
|
|
1 Retrying lost or stolen payment data can appear suspicious to card issuers.
Migration PGP key
If you’re unfamiliar with PGP, see GPG and start by importing a public key. After you familiarise yourself with the basics of PGP, use the following PGP key to encrypt sensitive data (such as credit card information) for PCI-compliant migration.
PGP migration key
This creates FILENAME.gpg with the following information:
- Key ID:
9C78B7620C1E99AD
- Key type:
RSA
- Key size:
4096 bits
- Fingerprint:
AEBF 7C48 38C4 4D2F DC99 A3F9 9C78 B762 0C1E 99AD
- User ID:
Stripe Import Key (PCI) <support-migrations@stripe.
com>
After you import our key, you can encrypt files to send by running this command in your command line prompt:
gpg --encrypt --recipient 9C78B7620C1E99AD FILENAME
For more details on providing encrypted data to Stripe, see Upload supplementary data.