Listen for address input
Use the Address Element to collect local and international addresses for your customers.
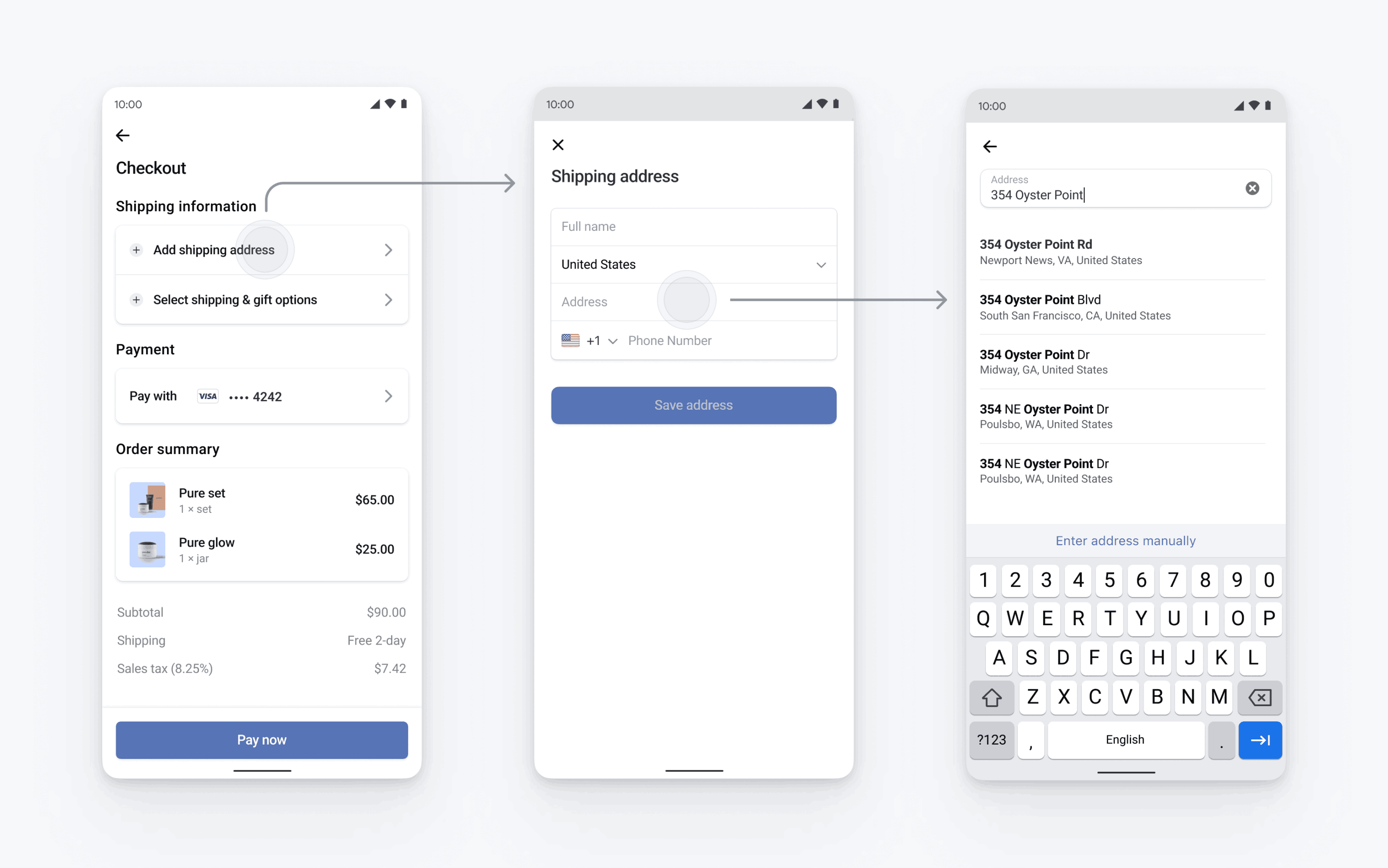
Set up Stripe
First, you need a Stripe account. Register now.
The Stripe Android SDK is open source and fully documented.
To install the SDK, add stripe-android
to the dependencies
block of your app/build.gradle
file:
apply plugin: 'com.android.application' android { ... } dependencies { // ... // Stripe Android SDK implementation 'com.stripe:stripe-android:20.43.0' }
Note
For details on the latest SDK release and past versions, see the Releases page on GitHub. To receive notifications when a new release is published, watch releases for the repository.
Configure the SDK with your Stripe publishable key so that it can make requests to the Stripe API, such as in your Application
subclass:
import com.stripe.android.PaymentConfiguration class MyApp : Application() { override fun onCreate() { super.onCreate() PaymentConfiguration.init( applicationContext,
) } }"pk_test_TYooMQauvdEDq54NiTphI7jx"
OptionalSet up address autocomplete suggestions
The address element uses the Google Places SDK to fetch address autocomplete suggestions. To enable autocomplete suggestions, you need to include the Google Places SDK dependency to your app’s build.gradle
.
dependencies { implementation 'com.google.android.libraries.places:places:2.6.0' }
Address autocomplete suggestions requires a Google Places API key. Follow the Google Places SDK setup guide to generate your API key.
Configure the Address Element
You can configure the Address Element with details such as displaying default values, setting allowed countries, customizing the appearance, and so on. Refer to AddressLauncher.Configuration
for the complete list of configuration options.
val addressConfiguration = AddressLauncher.Configuration( additionalFields: AddressLauncher.AdditionalFieldsConfiguration( phone: AdditionalFieldsConfiguration.FieldConfiguration.Required ), allowedCountries: setOf("US", "CA", "GB"), title: "Shipping Address", googlePlacesApiKey = "(optional) YOUR KEY HERE" )
Retrieve address details
Retrieve the address details by creating an instance of AddressLauncher
in the onCreate
lifecycle method of your Activity
or Fragment
and creating a callback method that implements the AddressLauncherResultCallback
interface.
private lateinit var addressLauncher: AddressLauncher private var shippingDetails: AddressDetails? = null override fun onCreate(savedInstanceState: Bundle?) { addressLauncher = AddressLauncher(this, ::onAddressLauncherResult) } private fun onAddressLauncherResult(result: AddressLauncherResult) { // TODO: Handle result and update your UI when (result) { is AddressLauncherResult.Succeeded -> { shippingDetails = result.address } is AddressLauncherResult.Canceled -> { // TODO: Handle cancel } } }
The AddressLauncherResult
can be Succeeded
or Canceled
. See more implementation details.
Note
Stripe requires that you instantiate the AddressLauncher
during the onCreate
lifecycle event and not after. Otherwise, the callback can’t be registered properly, and your app will crash.
Present the Address Element
Present the address element using the address launcher and configuration from the previous steps.
addressLauncher.present( publishableKey = publishableKey, configuration = addressConfiguration )