Collect card payments
Prepare your application and back end to collect card payments using Stripe Terminal.
For BBPOS WisePOS E and Stripe Reader S700, we recommend server-side integration because it uses the Stripe API instead of a Terminal SDK to collect payments.
Collecting payments with Stripe Terminal requires writing a payment flow in your application. Use the Stripe Terminal SDK to create and update a PaymentIntent, an object representing a single payment session.
While the core concepts are similar to SDK-based integrations, you follow slightly different steps with the server-driven integration:
- Create a PaymentIntent. You can define whether to automatically or manually capture your payments.
- Process the payment. Authorization on the customer’s card takes place when the reader processes the payment.
- (Optional) Capture the PaymentIntent.
Note
This integration shape does not support offline card payments.
Create a PaymentIntent
The first step in collecting payments is to start the payment flow. When a customer begins checking out, your backend must create a PaymentIntent object that represents a new payment session on Stripe. With the server-driven integration, you create the PaymentIntent server-side.
In a sandbox, you can use test amounts to simulate different error scenarios. In live mode, the amount of the PaymentIntent displays on the reader for payment.
For Terminal payments, the payment_
parameter must include card_
.
To accept Interac payments in Canada, you must also include interac_
in payment_
. Learn about regional considerations for Canada.
To accept non-card payment methods in supported countries, you must also specify your preferred types in payment_
. Learn about additional payment methods.
You can control the payment flow as follows:
- To fully control the payment flow for
card_
payments, set thepresent capture_
tomethod manual
. This allows you to add a reconciliation step before finalizing the payment. - To authorize and capture payments in one step, set the
capture_
tomethod automatic
.
Process the payment
You can process a payment immediately with the card presented by a customer, or instead inspect card details before proceeding to process the payment. For most use cases, we recommend processing immediately, as it is a simpler integration with less API calls and webhook events. However, if you would like to insert your own business logic before the card is authorized, you can use the two-step collect-and-confirm flow.
If you’re using a simulated reader, use the present_payment_method endpoint to simulate a cardholder tapping or inserting their card on the reader. Use test cards to simulate different success or failure scenarios.
Capture the payment
If you defined capture_
as manual
during PaymentIntent creation in Step 1, the SDK returns an authorized but not captured PaymentIntent to your application. Learn more about the difference between authorization and capture. When your application receives a confirmed PaymentIntent, make sure it notifies your backend to capture the PaymentIntent. To do so, create an endpoint on your backend that accepts a PaymentIntent ID and sends a request to the Stripe API to capture it.
A successful capture call results in a PaymentIntent with a status of succeeded
.
Warning
You must manually capture PaymentIntents
within two days or the authorization expires and funds are released to the customer.
Verify the reader state 
To make sure the reader completed an action, your application must verify the reader state before initiating a new reader action or continuing to capture the payment. In most cases, this verification allows you to confirm a successful (approved) payment and show any relevant UX to your operator for them to complete the transaction. In other cases, you might need to handle errors, including declined payments.
Use one of the following to check the reader status:
Listen to webhooks Recommended
For maximum resiliency, we recommend your application listens to webhooks from Stripe to receive real-time notifications of the reader status. Stripe sends three webhooks to notify your application of a reader’s action status:
Status | Description |
---|---|
terminal. | Sent when a reader action succeeds, such as when a payment is authorized successfully. |
terminal. | Sent when a reader action fails, such as when a card is declined due to insufficient funds. |
terminal. Preview | Sent when a reader action is updated, such as when a payment method is collected (only triggered for the collect_ action). |
To listen for these webhooks, create a webhook endpoint. We recommend having a dedicated webhook endpoint for only these events because they’re high priority and in the critical payment path.
Poll the Stripe API 
In case of webhook delivery issues, you can poll the Stripe API by adding a check status
button to your point of sale interface that the operator can invoke, if needed.
Use the PaymentIntent 
You can retrieve the PaymentIntent that you passed to the reader for processing. When you create a PaymentIntent it has an initial status of requires_
. After you successfully collect the payment method, the status updates to requires_
. After the payment processes successfully, the status updates to requires_
.
Use the reader object 
You can use the Reader object, which contains an action attribute that shows the latest action received by the reader and its status. Your application can retrieve a Reader to check if the status of the reader action has changed.
The Reader object is also returned as the response to the process payment step. The action
type when processing a payment is process_
.
The action.
updates to succeeded
for a successful payment. This means you can proceed with completing the transaction. Other values for action.
include failed
or in_
.
Handle errors 
The following errors are the most common types your application needs to handle:
- Avoiding double charges
- Payment failures
- Payment timeout
- Payment cancellation
- Reader busy
- Reader timeout
- Reader offline
- Missing webhooks
- Delayed webhooks
Avoiding double charges 
The PaymentIntent object enables money movement at Stripe—use a single PaymentIntent to represent a transaction.
Re-use the same PaymentIntent after a card is declined (for example, if it has insufficient funds), so your customer can try again with a different card.
If you edit the PaymentIntent, you must call process_payment_intent to update the payment information on the reader.
A PaymentIntent must be in the requires_
state before Stripe can process it. An authorized, captured, or canceled PaymentIntent can’t be processed by a reader and results in an intent_
error:
{ "error": { "code": "intent_invalid_state", "doc_url": "https://docs.stripe.com/error-codes#intent-invalid-state", "message": "Payment intent must be in the requires_payment_method state to be processed by a reader.", "type": "invalid_request_error" } }
Payment failures 
The most common payment failure is a failed payment authorization (for example, a payment that’s declined by the customer’s bank due to insufficient funds).
When a payment authorization fails, Stripe sends the terminal.
webhook. Check the action.failure_code and action.failure_message attributes to know why a payment is declined:
{ "id": "tmr_xxx", "object": "terminal.reader", "action": { "failure_code": "card_declined", "failure_message": "Your card has insufficient funds.", "process_payment_intent": { "payment_intent": "pi_xxx" }, "status": "failed", "type": "process_payment_intent" }, ... }
In the case of a declined card, prompt the customer for an alternative form of payment. Use the same PaymentIntent in another request to the process_payment_intent endpoint. If you create a new PaymentIntent, you must cancel the failed PaymentIntent to prevent double charges.
For card read errors (for example, an error reading the chip), the reader automatically prompts the customer to retry without any notification to your application. If multiple retries fail, you can prompt for another payment method by making another process_payment_intent request.
Payment timeout 
A reader with unreliable internet connectivity can fail to process a payment because of a networking request timeout when authorizing the card. The reader shows a processing screen for several seconds, followed by a failure screen, and you receive a terminal.
webhook with a failure_
of connection_
:
{ "id": "tmr_xxx", "object": "terminal.reader", "action": { "failure_code": "connection_error", "failure_message": "Could not connect to Stripe.", "process_payment_intent": { "payment_intent": "pi_xxx" }, "status": "failed", "type": "process_payment_intent" }, ... }
The payment confirmation request might have been processed by Stripe’s backend systems, but the reader might have disconnected before receiving the response from Stripe. When receiving a webhook with this failure code, fetch the PaymentIntent status
to verify if the payment is successfully authorized.
Make sure your network meets our network requirements to minimize timeouts.
Payment cancellation 
Programmatic cancellation
You might need to cancel an in-flight payment. For example, if a customer adds items to their purchase after your integration has already initiated payment collection on the reader. Use the cancel_action endpoint to reset the reader:
Note
You can’t cancel a reader action in the middle of a payment authorization. If a customer has already presented their card to pay on the reader, you must wait for processing to complete. An authorization normally takes a few seconds to complete. Calling cancel_action during an authorization results in a terminal_
error.
Customer-initiated cancellation
Users can set the value of enable_
on these endpoints:
When set to true, smart reader users see a cancel button.
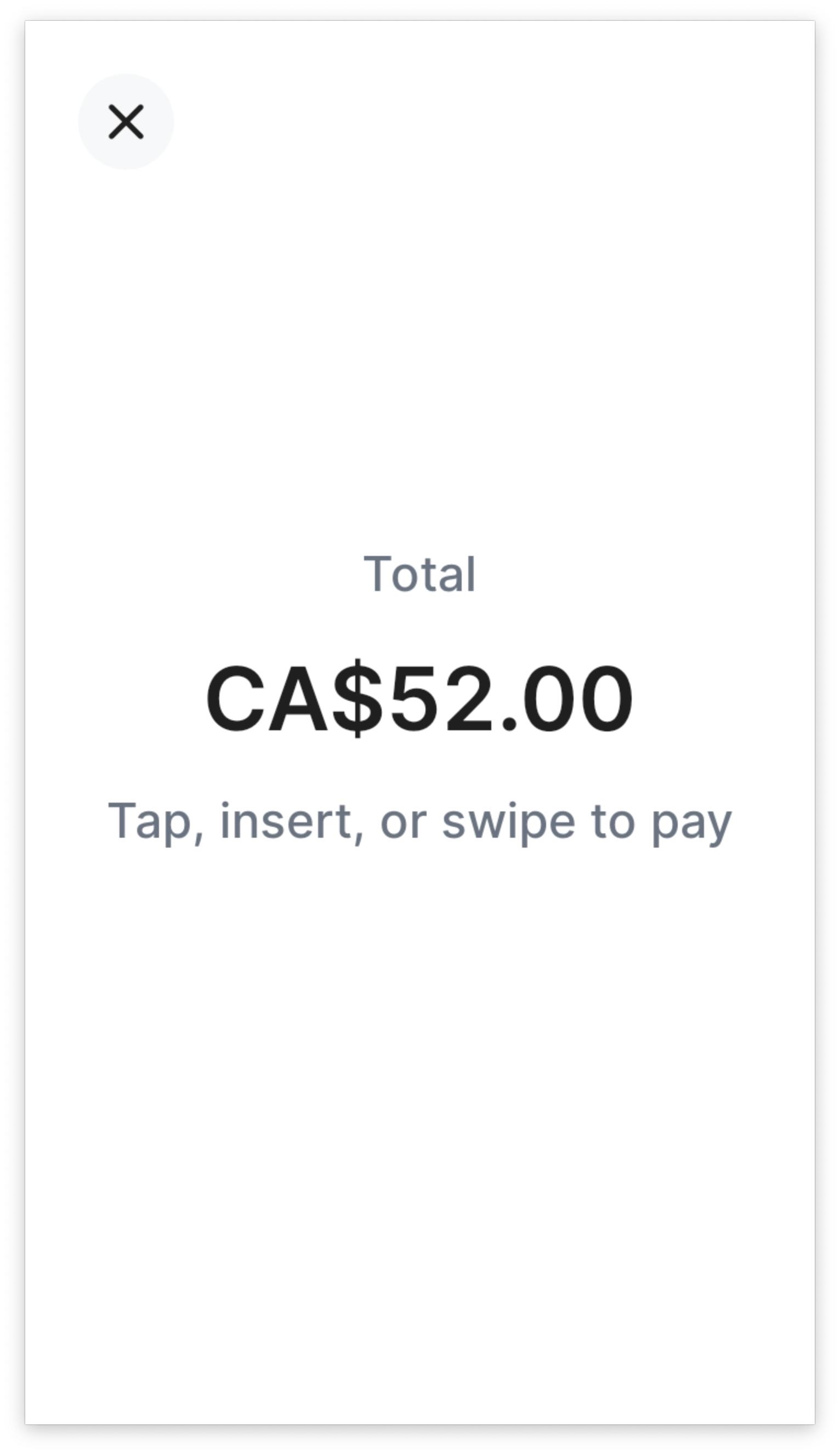
Payment collection with cancellation enabled
Tapping the cancel button cancels the active transaction. Stripe sends a terminal.
webhook with a failure_code of customer_
.
{ "action": { "failure_code": "customer_canceled", "failure_message": "This action could not be completed due to an error on the card reader.", "process_payment_intent": { "payment_intent": "pi_xxx", "process_config": { "enable_customer_cancellation": true } }, "status": "failed", "type": "process_payment_intent" } }
Reader busy 
A reader can process only one payment at a time. While it’s processing a payment, attempting a new payment fails with a terminal_
error:
{ "error": { "code": "terminal_reader_busy", "doc_url": "https://docs.stripe.com/error-codes#terminal-reader-timeout", "message": "Reader is currently busy processing another request. Please reference the integration guide at https://stripe.com/docs/terminal/payments/collect-card-payment?terminal-sdk-platform=server-driven#handle-errors for details on how to handle this error.", "type": "invalid_request_error" } }
Payments that have not begun processing can be replaced with a new payment.
A reader also rejects an API request if it’s busy performing updates, changing settings or if a card is inserted from the previous transaction.
Reader timeout 
On rare occasions, a reader might fail to respond to an API request on time because of temporary networking issues. If this happens, you receive a terminal_
error code:
{ "error": { "code": "terminal_reader_timeout", "doc_url": "https://docs.stripe.com/error-codes#terminal-reader-timeout", "message": "There was a timeout when sending this command to the reader. Please reference the integration guide at https://stripe.com/docs/terminal/payments/collect-card-payment?terminal-sdk-platform=server-driven#handle-errors for details on how to handle this error.", "type": "invalid_request_error" } }
In this case, we recommend you retry the API request. Make sure your network meets our network requirements to minimize timeouts.
On rare occasions, a terminal_
error code is a false negative. In this scenario, you receive a terminal_
error from the API as described above, but the reader has actually received the command successfully. False negatives happen when Stripe sends a message to the reader, but doesn’t receive an acknowledgement back from the reader due to temporary networking failures.
Reader offline 
A location losing its internet connection might result in interrupted communication between the reader and Stripe. In this case, a reader is unresponsive to events initiated from your point of sale application and backend infrastructure.
A reader that consistently fails to respond to API requests is most likely powered off (for example, the power cord is disconnected or it’s out of battery) or not correctly connected to the internet.
A reader is considered offline if Stripe hasn’t received any signal from that reader in the past 2 minutes. Attempting to call API methods on a reader that’s offline results in a terminal_
error code:
{ "error": { "code": "terminal_reader_offline", "doc_url": "https://docs.stripe.com/error-codes#terminal-reader-offline", "message": "Reader is currently offline, please ensure the reader is powered on and connected to the internet before retrying your request. Reference the integration guide at https://stripe.com/docs/terminal/payments/collect-card-payment?terminal-sdk-platform=server-driven#handle-errors for details on how to handle this error.", "type": "invalid_request_error" } }
Refer to our network requirements to make sure a reader is correctly connected to the internet.
Missing webhooks 
When a reader disconnects in the middle of a payment, it can’t update its action status in the API. In this scenario, the reader shows an error screen after a card is presented. However, the Reader object in the API doesn’t update to reflect the failure on the device, and you also don’t get reader action webhooks. A reader might be left with an action status of in_
when this happens, and a cashier has to intervene by calling the cancel_action endpoint to reset the reader state.
Delayed webhooks 
On rare occasions, if Stripe is having an outage, reader action webhooks might be late. You can query the status of the Reader or the PaymentIntent objects to know what their latest state is.
Webhook events 
Webhook | Description |
---|---|
terminal. | Sent when an asynchronous action succeeds. Sent for actions that need card presentment, such as process_ , confirm_ , process_ , and refund_ . |
terminal. | Sent when an asynchronous action fails. Sent for actions that need card presentment such as process_ , process_ , refund_ . No webhook is sent for the set_ and cancel_ actions. Your integration must handle these errors. |
terminal. | Sent when an asynchronous action is updated. Sent for actions such as collect_ . |