Accept a stablecoin paymentPublic preview
Start accepting stablecoins by integrating the Crypto payment method.
You can accept stablecoin payments with Checkout, Elements, or can be directly integrated through the Payment Intents API. If you’re a Connect platform, see Connect support.
When integrated, the option to pay with crypto appears in your checkout page, redirecting customers to a page hosted by crypto.link.com for payment completion. There, your customers can connect their wallet, and save and reuse their account using Link. You’re immediately notified if the payment succeeds or fails. Before you get started, see our demo.
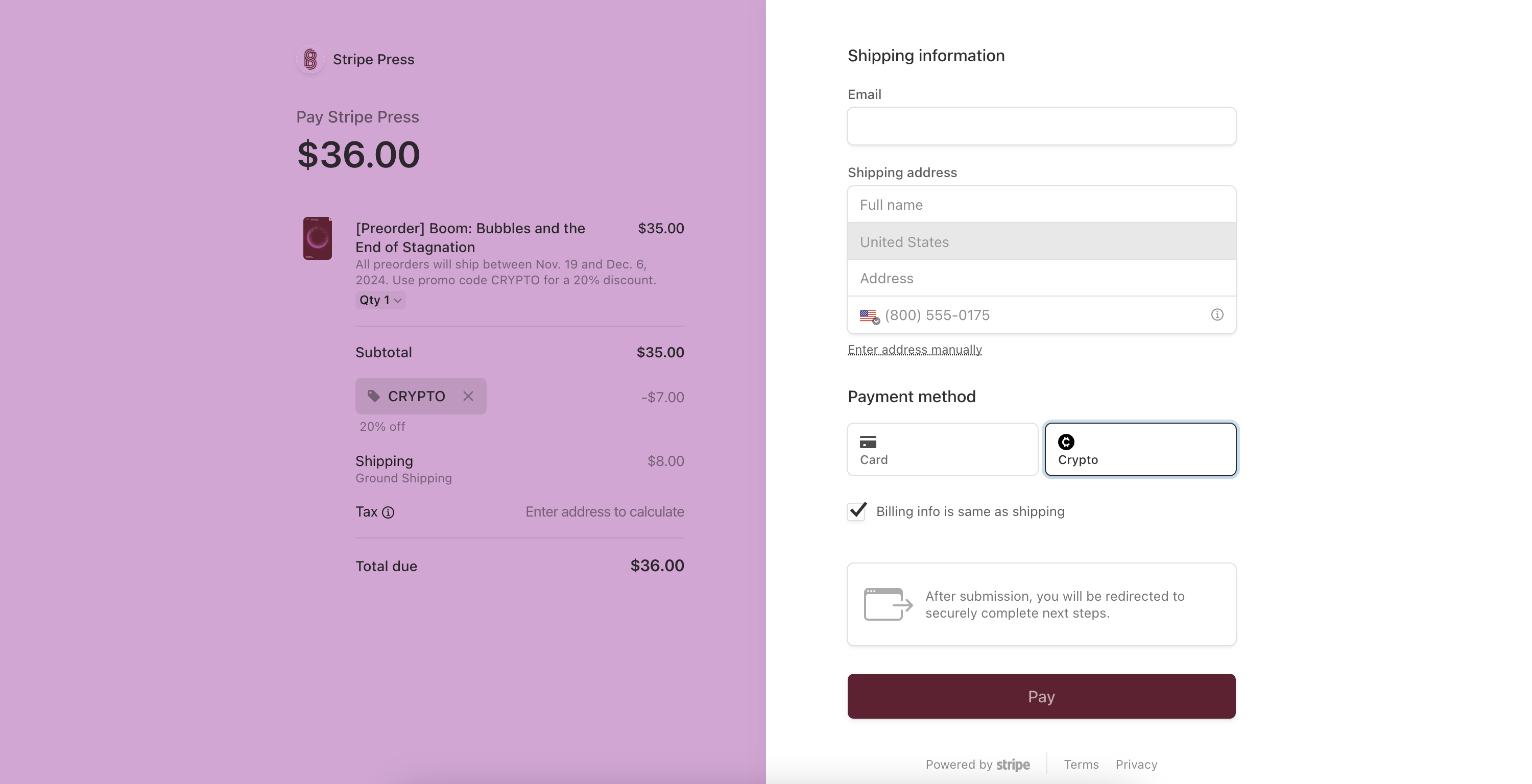
Let your users pay with crypto
Integrate Pay with Crypto directly through the Payment Intents API. Before you create a PaymentIntent, turn on Crypto in your payment methods settings.
Set up StripeServer-side
First, create a Stripe account or sign in.
Use our official libraries to access the Stripe API from your application:
Create a PaymentIntent and retrieve the client secretServer-side
The PaymentIntent object represents your intent to collect payment from your customer and tracks the lifecycle of the payment process. Create a PaymentIntent on your server and specify the amount to collect and a supported currency. If you have an existing Payment Intents integration, add crypto
to the list of payment_method_types.
curl https://api.stripe.com/v1/payment_intents \ -u
: \ -d "payment_method_types[]"=crypto \ -d amount=1099 \ -d currency=usdsk_test_BQokikJOvBiI2HlWgH4olfQ2
Retrieve the client secret
The PaymentIntent includes a client secret that the client side uses to securely complete the payment process. You can use different approaches to pass the client secret to the client side.
Redirect to the stablecoin payments page
Use Stripe.js to submit the payment to Stripe when a customer chooses Crypto as a payment method. Stripe.js is the foundational JavaScript library for building payment flows. It automatically handles complexities like the redirect described below, and lets you extend your integration to other payment methods. Include the Stripe.js script on your checkout page by adding it to the <head>
of your HTML file.
<head> <title>Checkout</title> <script src="https://js.stripe.com/v3/"></script> </head>
Create an instance of Stripe.js with the following JavaScript on your checkout page:
// Set your publishable key. Remember to change this to your live publishable key in production! // See your keys here: https://dashboard.stripe.com/apikeys const stripe = Stripe(
);'pk_test_TYooMQauvdEDq54NiTphI7jx'
Use the PaymentIntent client secret and call stripe.
to handle the Pay with Crypto redirect. Add a return_
to determine where Stripe redirects the customer after they complete the payment:
const form = document.getElementById('payment-form'); form.addEventListener('submit', async function(event) { event.preventDefault(); // Set the clientSecret of the PaymentIntent const { error } = await stripe.confirmPayment({ clientSecret: clientSecret, confirmParams: { payment_method_data: { type: 'crypto', }, // Return URL where the customer should be redirected after the authorization return_url: `${window.location.href}`, }, }); if (error) { // Inform the customer that there was an error. const errorElement = document.getElementById('error-message'); errorElement.textContent = result.error.message; } });
The return_
corresponds to a page on your website that displays the result of the payment. You can determine what to display by verifying the status of the PaymentIntent. To verify the status, the Stripe redirect to the return_
includes the following URL query parameters. You can also append your own query parameters to the return_
. They persist throughout the redirect process.
payment_ | The unique identifier for the PaymentIntent . |
payment_ | The client secret of the PaymentIntent object. |
Test your integration 
Test your Pay with Crypto integration with your test API keys by viewing the redirect page. You can test the successful payment case by authenticating the payment on the redirect page. The PaymentIntent transitions from requires_
to succeeded
.
In sandbox, pay with testnet crypto assets on the payment page you’re redirected to.
Configure your wallet to the test network you intend to pay over. For example, if you want to pay with USDC on Ethereum, make sure your wallet is set to Ethereum’s Sepolia test network.
Fund your wallet with test assets
You can use testnet “faucets” to top up your wallet. Here are a few: