Build a subscriptions integration
Create and manage subscriptions to accept recurring payments.
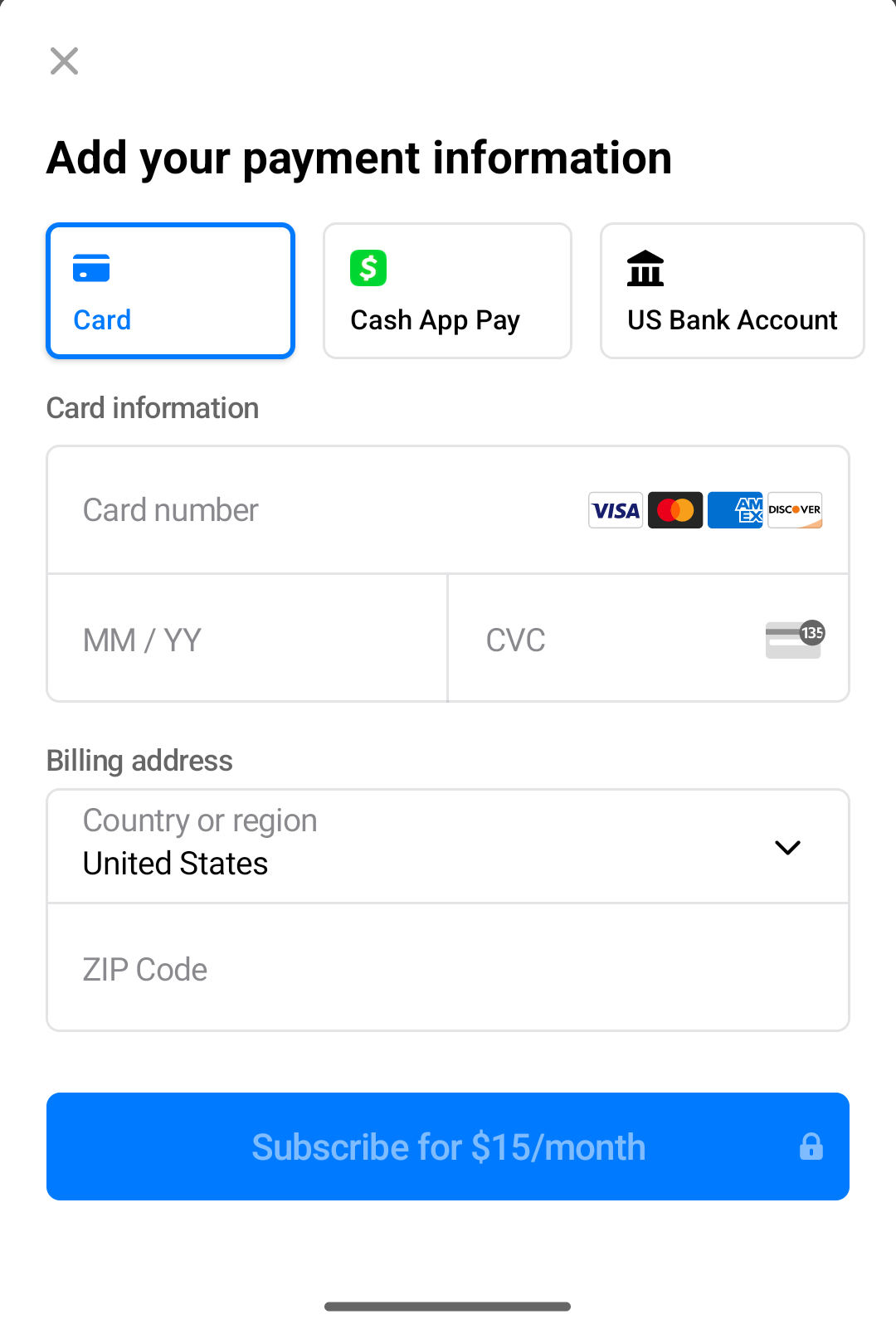
Customise with the Appearance API.
Use this guide to learn how to sell fixed-price subscriptions. You’ll use the Mobile Payment Element to create a custom payment form that you embed in your app.
Note
If you’re selling digital products or services that are consumed within your app (for example, subscriptions, in-game currencies, game levels, access to premium content, or unlocking a full version), you must use Apple’s in-app purchase APIs. This rule has some exceptions, including one-to-one personal services and apps based in specific regions. See the App Store review guidelines for more information.
Build your subscription
This guide shows you how to:
- Model your business by building a product catalogue.
- Create a registration process to add customers.
- Create subscriptions and collect payment information.
- Test and monitor the status of payments and subscriptions.
- Let customers change their plan or cancel the subscription.
- Learn how to use flexible billing mode to access enhanced billing behavior and additional features.
How to model it on Stripe
Subscriptions simplify your billing by automatically creating Invoices and PaymentIntents for you. To create and activate a subscription, you need to first create a Product to model what is being sold, and a Price which determines the interval and amount to charge. You also need a Customer to store PaymentMethods used to make each recurring payment.
API object definitions
Set up Stripe
The React Native SDK is open source and fully documented. Internally, it uses the native iOS and Android SDKs. To install Stripe’s React Native SDK, run one of the following commands in your project’s directory (depending on which package manager you use):
Next, install some other necessary dependencies:
- For iOS, navigate to the ios directory and run
pod install
to ensure that you also install the required native dependencies. - For Android, there are no more dependencies to install.
Note
We recommend following the official TypeScript guide to add TypeScript support.
Stripe initialisation
To initialise Stripe in your React Native app, either wrap your payment screen with the StripeProvider
component, or use the initStripe
initialisation method. Only the API publishable key in publishableKey
is required. The following example shows how to initialise Stripe using the StripeProvider
component.
import { useState, useEffect } from 'react'; import { StripeProvider } from '@stripe/stripe-react-native'; function App() { const [publishableKey, setPublishableKey] = useState(''); const fetchPublishableKey = async () => { const key = await fetchKey(); // fetch key from your server here setPublishableKey(key); }; useEffect(() => { fetchPublishableKey(); }, []); return ( <StripeProvider publishableKey={publishableKey} merchantIdentifier="merchant.identifier" // required for Apple Pay urlScheme="your-url-scheme" // required for 3D Secure and bank redirects > {/* Your app code here */} </StripeProvider> ); }
Note
And then install the Stripe CLI. The CLI provides webhook testing and you can run it to make API calls to Stripe. This guide shows how to use the CLI to set up a pricing model in a later section.
For additional install options, see Get started with the Stripe CLI.
Create the pricing modelStripe CLI or Dashboard
Create your products and their prices in the Dashboard or with the Stripe CLI.
This example uses a fixed-price service with two different service-level options: Basic and Premium. For each service-level option, you need to create a product and a recurring price. (If you want to add a one-off charge for something like a setup fee, create a third product with a one-off price. To keep things simple, this example doesn’t include a one-off charge.)
In this example, each product bills at monthly intervals. The price for the Basic product is 5 USD. The price for the Premium product is 15 USD.
Create the customerClient and Server
Stripe needs a customer for each subscription. In your application frontend, collect any necessary information from your users and pass it to the back end.
If you need to collect address details, the Address Element enables you to collect a shipping or billing address for your customers. For more information on the Address Element, visit the Address Element page.
import React from 'react'; import {View, TextInput, StyleSheet, Button, Platform} from 'react-native'; function RegisterView() { const [email, setEmail] = React.useState(''); const createCustomer = async () => { const apiEndpoint = Platform.OS === 'ios' ? 'http://localhost:4242' : 'http://10.0.2.2:4567'; const response = await fetch(`${apiEndpoint}/create-customer`, { method: 'POST', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify({ email: email, }), }); if (response.status === 200) { const customer = await response.json(); console.log(customer); } }; return ( <View> <TextInput style={styles.input} placeholder="Email" value={email} onChangeText={setEmail} /> <Button title="Register" onPress={async () => { await createCustomer(); }} /> </View> ); } const styles = StyleSheet.create({ input: { height: 40, margin: 12, borderWidth: 1, padding: 10, }, }); export default RegisterView;
On the server, create the Stripe customer object.
Create the subscriptionClient and Server
Note
If you want to render the Payment Element without first creating a subscription, see Collect payment details before creating an Intent.
Let your new customer choose a plan and then create the subscription – in this guide, they choose between Basic and Premium.
In your app, pass the selected price ID and the ID of the customer record to the backend.
const createSubscription = async (priceId, customerId) => { const apiEndpoint = Platform.OS === 'ios' ? 'http://localhost:4242' : 'http://10.0.2.2:4567'; const response = await fetch(`${apiEndpoint}/create-subscription`, { method: 'POST', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify({ priceId: priceId, customerId: customerId, }), }); if (response.status === 200) { const subscription = await response.json(); return subscription; } };
On the back end, create the subscription with status incomplete
using payment_
. Then return the client_
from the subscription’s first payment intent to the front end to complete payment by expanding theconfirmation_
on the latest invoice of the subscription.
To enable improved subscription behavior, set billing_
to flexible
. You must use Stripe API version 2025-06-30.basil or later.
Set save_default_payment_method to on_
to save the payment method as the default for a subscription when a payment succeeds. Saving a default payment method increases the success rate of future subscription payments.
Note
If you’re using a multi-currency Price, use the currency parameter to tell the Subscription which of the Price’s currencies to use. (If you omit the currency
parameter, then the Subscription uses the Price’s default currency.)
At this point the Subscription is inactive
and awaiting payment. Here’s an example response. The minimum fields to store are highlighted, but store whatever your application frequently accesses.
{ "id": "sub_JgRjFjhKbtD2qz", "object": "subscription", "application_fee_percent": null, "automatic_tax": { "disabled_reason": null, "enabled": false, "liability": "null" }, "billing_cycle_anchor": 1623873347,
Collect payment informationClient
Use the Mobile Payment Element to collect payment details and activate the subscription. You can customise Elements to match the look and feel of your application.
The Mobile Payment Element securely collects all necessary payment details for a wide variety of payments methods. Learn about the supported payment methods for Mobile Payment Element and Subscriptions.
Add the Payment Element to your app
Note
This step shows one way to get started, but you can use any in-app payments integration.
Initialise and present the Mobile Payment Element using the PaymentSheet class.
import React from 'react'; import {useStripe, PaymentSheetError} from '@stripe/stripe-react-native'; import {View, Button} from 'react-native'; function SubscribeView({clientSecret}) { const {initPaymentSheet, presentPaymentSheet} = useStripe(); React.useEffect(() => { const initializePaymentSheet = async () => { const {error} = await initPaymentSheet({ paymentIntentClientSecret: clientSecret, returnURL: 'stripe-example://payment-sheet', // Set `allowsDelayedPaymentMethods` to true if your business handles // delayed notification payment methods like US bank accounts. allowsDelayedPaymentMethods: true, }); if (error) { // Handle error } }; initializePaymentSheet(); }, [clientSecret, initPaymentSheet]); return ( <View> <Button title="Subscribe" onPress={async () => { const {error} = await presentPaymentSheet(); if (error) { if (error.code === PaymentSheetError.Failed) { // Handle failed } else if (error.code === PaymentSheetError.Canceled) { // Handle canceled } } else { // Payment succeeded } }} /> </View> ); } export default SubscribeView;
The Mobile Payment Element renders a sheet that allows your customer to select a payment method. The form automatically collects all necessary payments details for the payment method that they select.
Setting allowsDelayedPaymentMethods
to true allows delayed notification payment methods like US bank accounts. For these payment methods, the final payment status isn’t known when the PaymentSheet
completes, and instead succeeds or fails later. If you support these types of payment methods, inform the customer their order is confirmed and only fulfil their order (for example, ship their product) when the payment is successful.
You can customise the Payment Element to match the design of your app by using the appearance
property your PaymentSheet.
object.
Confirm payment
The Mobile Payment Element creates a PaymentMethod and confirms the incomplete Subscription’s first PaymentIntent, causing a charge to be made. If Strong Customer Authentication (SCA) is required for the payment, the Payment Element handles the authentication process before confirming the PaymentIntent.
Set up a return URL (iOS only)Client-side
When a customer exits your app (for example to authenticate in Safari or their banking app), provide a way for them to automatically return to your app. Many payment method types require a return URL. If you don’t provide one, we can’t present payment methods that require a return URL to your users, even if you’ve enabled them.
To provide a return URL:
- Register a custom URL. Universal links aren’t supported.
- Configure your custom URL.
- Set up your root component to forward the URL to the Stripe SDK as shown below.
Note
If you’re using Expo, set your scheme in the app.
file.
import { useEffect, useCallback } from 'react'; import { Linking } from 'react-native'; import { useStripe } from '@stripe/stripe-react-native'; export default function MyApp() { const { handleURLCallback } = useStripe(); const handleDeepLink = useCallback( async (url: string | null) => { if (url) { const stripeHandled = await handleURLCallback(url); if (stripeHandled) { // This was a Stripe URL - you can return or add extra handling here as you see fit } else { // This was NOT a Stripe URL – handle as you normally would } } }, [handleURLCallback] ); useEffect(() => { const getUrlAsync = async () => { const initialUrl = await Linking.getInitialURL(); handleDeepLink(initialUrl); }; getUrlAsync(); const deepLinkListener = Linking.addEventListener( 'url', (event: { url: string }) => { handleDeepLink(event.url); } ); return () => deepLinkListener.remove(); }, [handleDeepLink]); return ( <View> <AwesomeAppComponent /> </View> ); }
Additionally, set the returnURL
when you call the initPaymentSheet
method:
await initPaymentSheet({ ... returnURL: 'your-app://stripe-redirect', ... });
For more information on native URL schemes, refer to the Android and iOS docs.
Listen for webhooksServer
To complete the integration, you need to process webhooks sent by Stripe. These are events triggered whenever state inside of Stripe changes, such as subscriptions creating new invoices. In your application, set up an HTTP handler to accept a POST request containing the webhook event, and verify the signature of the event:
During development, use the Stripe CLI to observe webhooks and forward them to your application. Run the following in a new terminal while your development app is running:
stripe listen --forward-to localhost:4242/webhook
For production, set up a webhook endpoint URL in the Dashboard, or use the Webhook Endpoints API.
You’ll listen to a couple of events to complete the remaining steps in this guide. See Subscription events for more details about subscription-specific webhooks.
Provision access to your serviceClient and Server
Now that the subscription is active, give your user access to your service. To do this, listen to the customer.
, customer.
, and customer.
events. These events pass a subscription object which contains a status
field indicating whether the subscription is active, overdue, or cancelled. See the subscription lifecycle for a complete list of statuses.
In your webhook handler:
- Verify the subscription status. If it’s
active
then your user has paid for your product. - Check the product the customer subscribed to and grant access to your service. Checking the product instead of the price gives you more flexibility if you need to change the pricing or billing interval.
- Store the
product.
,id subscription.
andid subscription.
in your database along with thestatus customer.
you already saved. Check this record when determining which features to enable for the user in your application.id
The state of a subscription might change at any point during its lifetime, even if your application does not directly make any calls to Stripe. For example, a renewal might fail due to an expired credit card, which puts the subscription into an overdue state. Or, if you implement the customer portal, a user might cancel their subscription without directly visiting your application. Implementing your handler correctly keeps your application state in sync with Stripe.
Cancel the subscriptionClient and Server
It’s common to allow customers to cancel their subscriptions. This example adds a cancellation option to the account settings page.
The example collects the subscription ID on the front end, but your application can get this information from your database for your logged-in user.
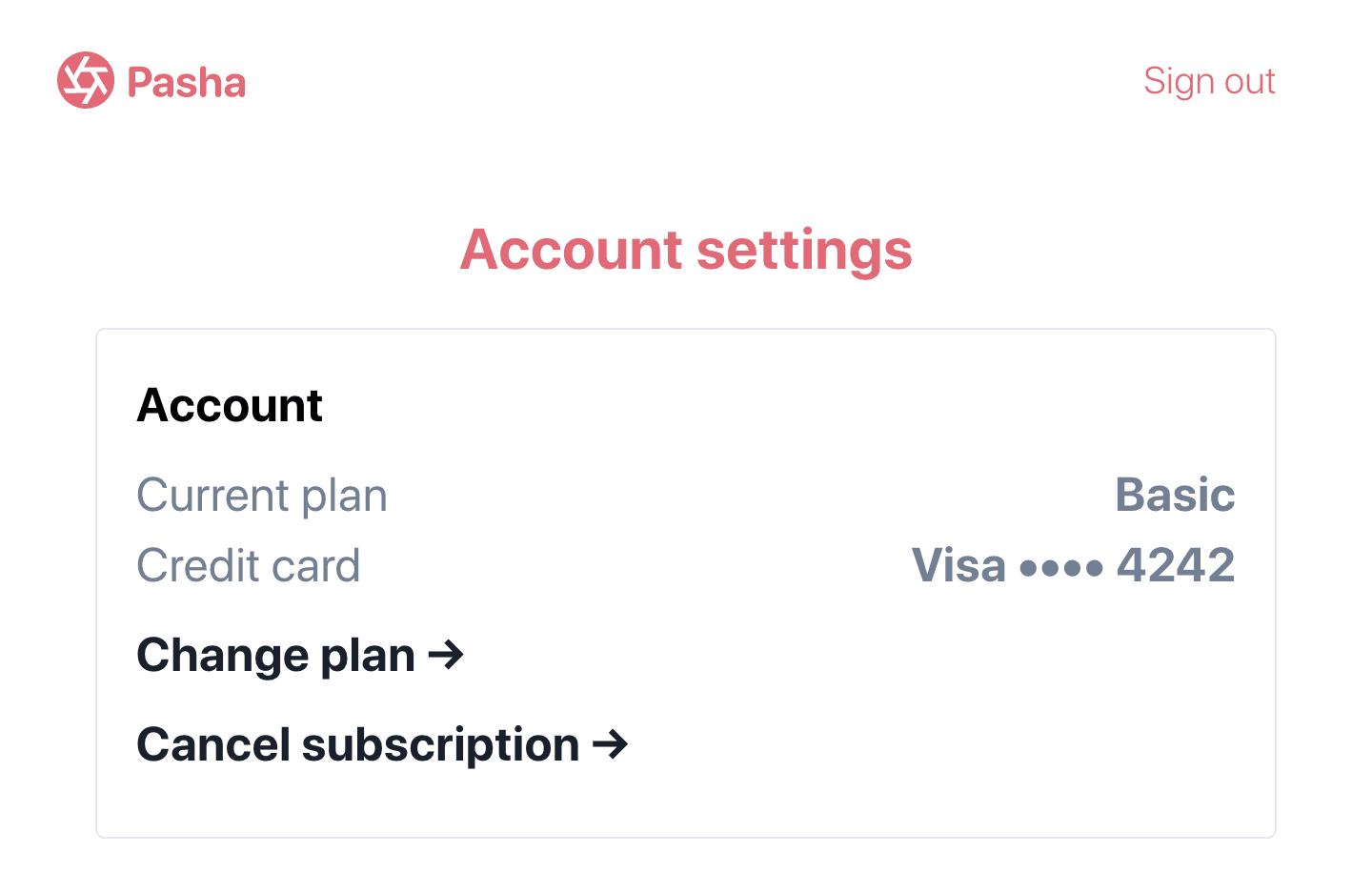
Account settings with the ability to cancel the subscription
const cancelSubscription = async subscriptionId => { const apiEndpoint = Platform.OS === 'ios' ? 'http://localhost:4242' : 'http://10.0.2.2:4567'; const response = await fetch(`${apiEndpoint}/cancel-subscription`, { method: 'POST', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify({ subscriptionId: subscriptionId, }), }); if (response.status === 200) { const subscription = await response.json(); return subscription; } };
On the back end, define the endpoint for your app to call.
Your back end receives a customer.
event.
After the subscription is cancelled, update your database to remove the Stripe subscription ID you previously stored, and limit access to your service.
When a subscription is cancelled, it can’t be reactivated. Instead, collect updated billing information from your customer, update their default payment method, and create a new subscription with their existing customer record.
Test your integration
Test payment methods
Use the following table to test different payment methods and scenarios.
Payment method | Scenario | How to test |
---|---|---|
BECS Direct Debit | Your customer successfully pays with BECS Direct Debit. | Fill out the form using the account number 900123456 and BSB 000-000 . The confirmed PaymentIntent initially transitions to processing , then transitions to the succeeded status three minutes later. |
BECS Direct Debit | Your customer’s payment fails with an account_ error code. | Fill out the form using the account number 111111113 and BSB 000-000 . |
Credit card | The card payment succeeds and doesn’t require authentication. | Fill out the credit card form using the credit card number 4242 4242 4242 4242 with any expiration, CVC, and postal code. |
Credit card | The card payment requires authentication. | Fill out the credit card form using the credit card number 4000 0025 0000 3155 with any expiration, CVC, and postal code. |
Credit card | The card is declined with a decline code like insufficient_ . | Fill out the credit card form using the credit card number 4000 0000 0000 9995 with any expiration, CVC, and postal code. |
SEPA Direct Debit | Your customer successfully pays with SEPA Direct Debit. | Fill out the form using the account number AT321904300235473204 . The confirmed PaymentIntent initially transitions to processing, then transitions to the succeeded status three minutes later. |
SEPA Direct Debit | Your customer’s PaymentIntent status transitions from processing to requires_ . | Fill out the form using the account number AT861904300235473202 . |
Monitor events
Set up webhooks to listen to subscription change events, such as upgrades and cancellations. Learn more about subscription webhooks. You can view events in the Dashboard or with the Stripe CLI.
For more details, see testing your Billing integration.
Disclose Stripe to your customers
Stripe collects information on customer interactions with Elements to provide services to you, prevent fraud, and improve its services. This includes using cookies and IP addresses to identify which Elements a customer saw during a single checkout session. You’re responsible for disclosing and obtaining all rights and consents necessary for Stripe to use data in these ways. For more information, visit our privacy center.