Customer Sheet を導入する
顧客がアプリの設定で保存済みの決済手段を管理できるようにします。
注
Customer Sheet は、アプリの設定ページでの使用を目的としています。決済フローと支払いには、Mobile Payment Element を使用します。Mobile Payment Element には、決済手段の保存と表示のサポートも組み込まれており、Customer Sheet よりも多くの決済手段に対応しています。
Customer Sheet は、顧客が保存済みの決済手段を管理するための構築済み UI コンポーネントです。Customer Sheet UI は決済フロー以外で使用することができ、外観やスタイルは自社のアプリに合わせてカスタマイズ可能です。顧客は、Customer オブジェクトに保存される決済手段を追加および削除し、デバイスのローカル上に保存されるデフォルトの決済手段を設定できます。Mobile Payment Element と Customer Sheet の両方を使用して、保存された決済手段に対して一貫性のあるエンドツーエンドのソリューションを顧客に提供します。
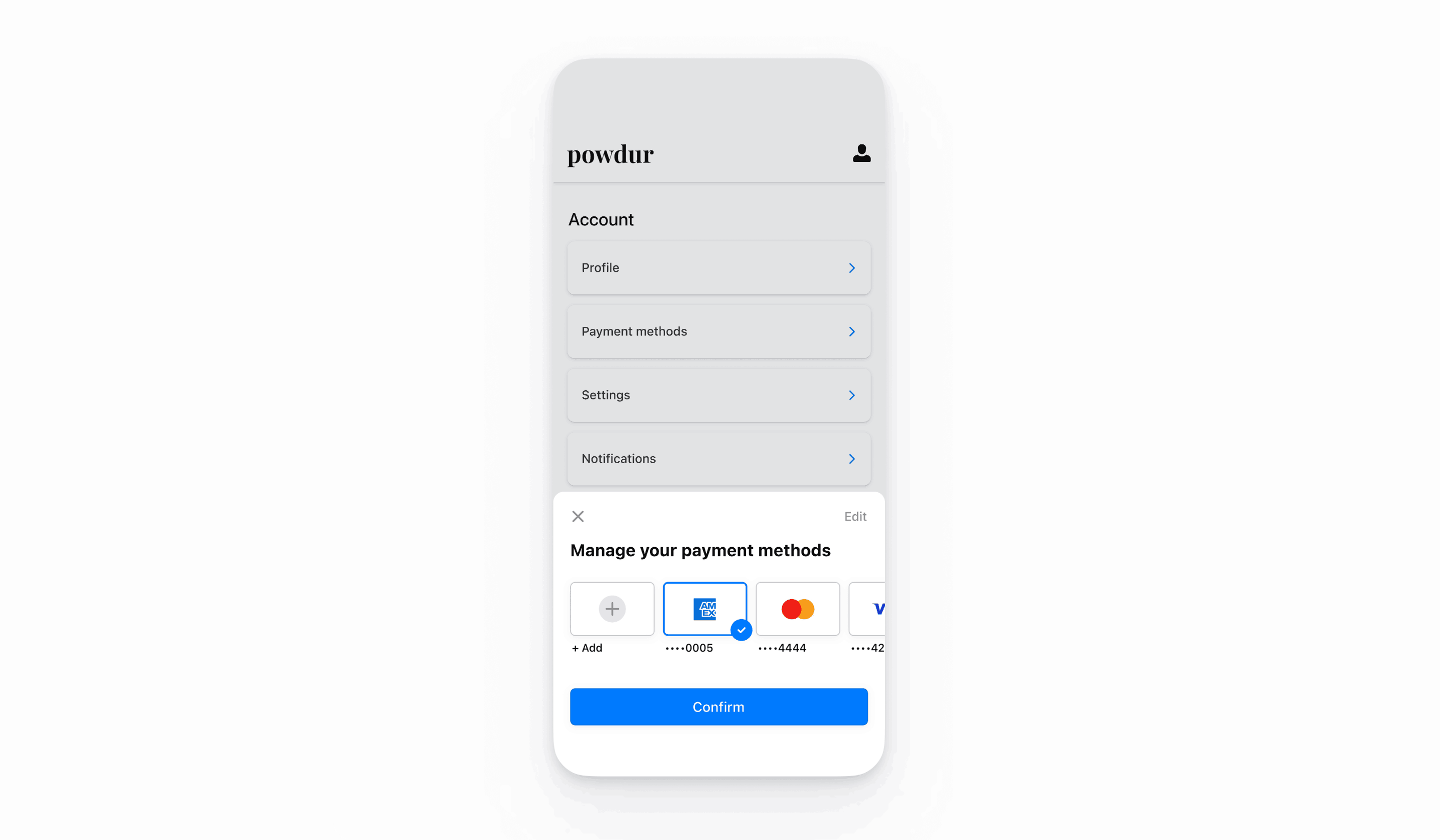
Stripe を設定する
まず、Stripe アカウントが必要です。今すぐ登録してください。
React Native SDK はオープンソースであり、詳細なドキュメントが提供されています。内部では、ネイティブの iOS および Android の SDK を使用します。Stripe の React Native SDK をインストールするには、プロジェクトのディレクトリーで (使用するパッケージマネージャーによって異なる) 次のいずれかのコマンドを実行します。
次に、その他の必要な依存関係をインストールします。
- iOS の場合は、ios ディレクトリーに移動して
pod install
を実行し、必要なネイティブ依存関係もインストールします。 - Android の場合は、依存関係をインストールする必要はありません。
注
公式の TypeScript ガイドに従って TypeScript のサポートを追加することをお勧めします。
Stripe の初期化
React Native アプリで Stripe を初期化するには、決済画面を StripeProvider
コンポーネントでラップするか、initStripe
初期化メソッドを使用します。publishableKey
の API 公開可能キーのみが必要です。次の例は、StripeProvider
コンポーネントを使用して Stripe を初期化する方法を示しています。
import { useState, useEffect } from 'react'; import { StripeProvider } from '@stripe/stripe-react-native'; function App() { const [publishableKey, setPublishableKey] = useState(''); const fetchPublishableKey = async () => { const key = await fetchKey(); // fetch key from your server here setPublishableKey(key); }; useEffect(() => { fetchPublishableKey(); }, []); return ( <StripeProvider publishableKey={publishableKey} merchantIdentifier="merchant.identifier" // required for Apple Pay urlScheme="your-url-scheme" // required for 3D Secure and bank redirects > {/* Your app code here */} </StripeProvider> ); }
支払い方法を有効にする
支払い方法の設定を表示して、サポートする支払い方法を有効にします。SetupIntent を作成するには、少なくとも 1 つは支払い方法を有効にする必要があります。
多くの顧客から決済を受け付けられるよう、Stripe では、カードやその他一般的な決済手段がデフォルトで有効になっていますが、ビジネスや顧客に適した追加の決済手段を有効にすることをお勧めします。プロダクトと決済手段のサポートについては決済手段のサポートを、手数料については料金体系ページをご覧ください。
注
現時点では、CustomerSheet はカードとアメリカの銀行口座のみに対応しています。
Customer エンドポイントを追加するサーバー側
サーバー側で、Customer の一時キーを取得するエンドポイントと、新しい決済手段を Customer に保存する SetupIntent を作成するエンドポイントの 2 つのエンドポイントを作成します。
- Customer ID を指定して設定された SetupIntent を返すエンドポイントを作成します。
今後の支払いで、顧客が決済フローでオンセッション時にのみ決済手段を使用する場合、usage パラメーターを on_session に設定して、オーソリ率を改善します。
画面を初期化する
次に、希望の設定を CustomerSheetBeta.
に指定して、Customer Sheet を設定します。
import {CustomerSheetBeta} from '@stripe/stripe-react-native'; const {error} = await CustomerSheetBeta.initialize({ setupIntentClientSecret: 'SETUP-INTENT', customerEphemeralKeySecret: 'EPHEMERAL-KEY', customerId: 'CUSTOMER-ID', headerTextForSelectionScreen: 'Manage your payment method', returnURL: 'my-return-url://', });