Integrate the Customer Sheet
Let your customers manage their saved payment methods in your app settings.
Note
The Customer Sheet is intended for use on an app settings page. For checkout and payments, use the Mobile Payment Element, which also has built-in support for saving and displaying payment methods and supports more payment methods than the Customer Sheet.
The Customer Sheet is a prebuilt UI component that lets your customers manage their saved payment methods. You can use the Customer Sheet UI outside of a checkout flow, and the appearance and styling is customizable to match the appearance and aesthetic of your app. Customers can add and remove payment methods, which get saved to the customer object, and set their default payment method stored locally on the device. Use both the Mobile Payment Element and the Customer Sheet to provide customers a consistent end-to-end solution for saved payment methods.
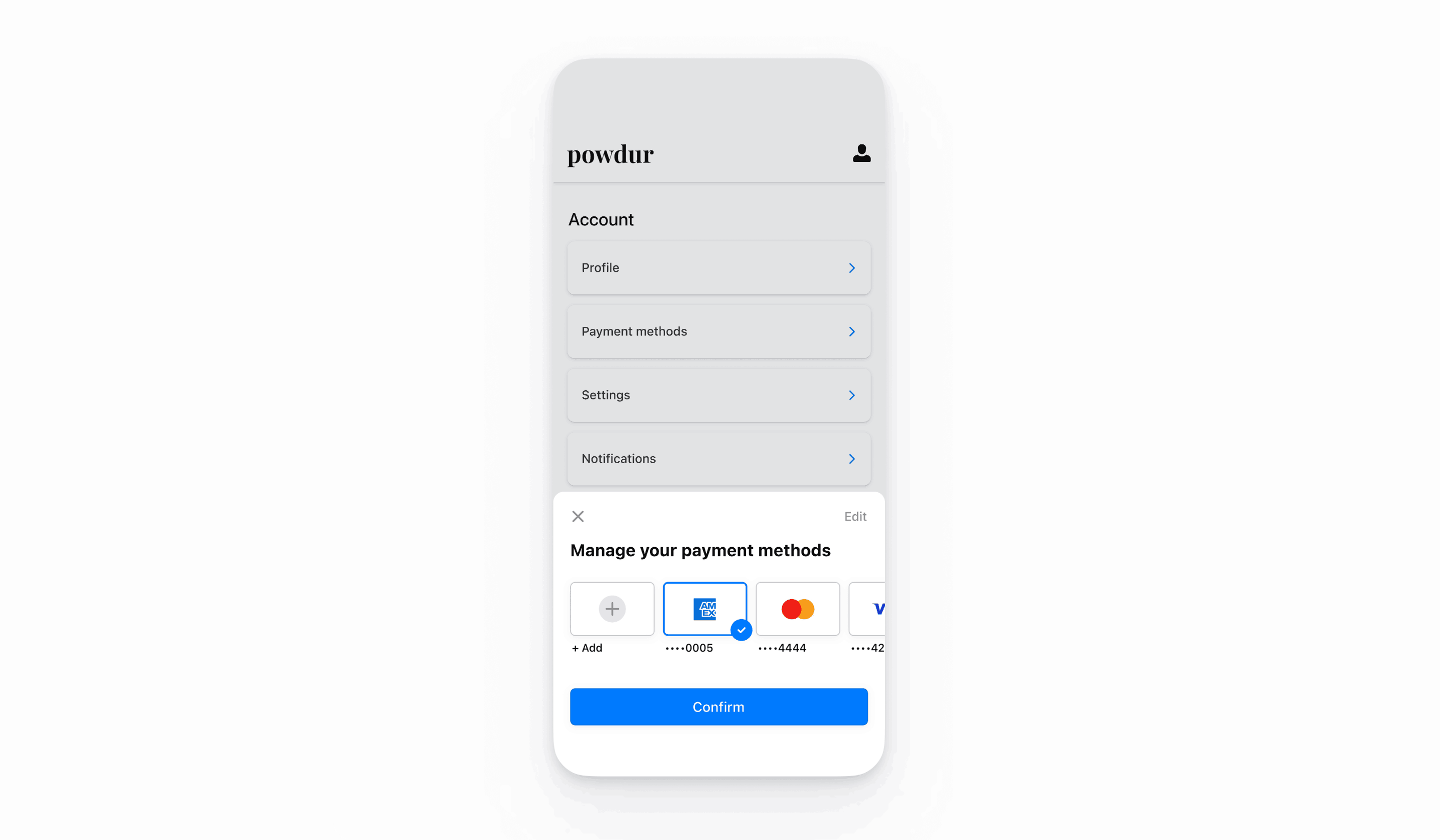
CustomerAdapter uses Customer Ephemeral Keys and serves as a bridge to help users of legacy products adopt CustomerSheet more quickly. If you’re starting a new integration, we recommend adopting CustomerSession over Customer Ephemeral Keys.
Set up Stripe
First, you need a Stripe account. Register now.
The Stripe iOS SDK is open source, fully documented, and compatible with apps supporting iOS 13 or above.
Note
For details on the latest SDK release and past versions, see the Releases page on GitHub. To receive notifications when a new release is published, watch releases for the repository.
Configure the SDK with your Stripe publishable key on app start. This enables your app to make requests to the Stripe API.
Enable payment methods
View your payment methods settings and enable the payment methods you want to support. You need at least one payment method enabled to create a SetupIntent.
By default, Stripe enables cards and other prevalent payment methods that can help you reach more customers, but we recommend turning on additional payment methods that are relevant for your business and customers. See Payment method support for product and payment method support, and our pricing page for fees.
Note
CustomerSheet only supports cards, US bank accounts, and SEPA Direct Debit.
Add Customer endpointsServer-side
Create two endpoints on your server: one for fetching a Customer’s ephemeral key, and one to create a SetupIntent for saving a new payment method to the Customer.
- Create an endpoint to return a Customer ID and an associated ephemeral key. You can view the API version used by the SDK here.
- Create an endpoint to return a SetupIntent configured with the Customer ID.
If you only plan to use the payment method for future payments when your customer is present during the checkout flow, set the usage parameter to on_session to improve authorization rates.
Create a Customer adapterClient-side
A StripeCustomerAdapter
enables a CustomerSheet
to communicate with Stripe. On the client, configure a StripeCustomerAdapter
with providers that make requests to these endpoints on your server.
import StripePaymentSheet let customerAdapter = StripeCustomerAdapter(customerEphemeralKeyProvider: { let json = await myBackend.getCustomerEphemeralKey() return CustomerEphemeralKey(customerId: json["customerId"]!, ephemeralKeySecret: json["ephemeralKeySecret"]!) }, setupIntentClientSecretProvider: { let json = await myBackend.getSetupIntentForCustomer() return json["setupIntentClientSecret"]! })
Configure the sheet
Next, configure the Customer Sheet with your StripeCustomerAdapter
and a CustomerSheet.Configuration.
var configuration = CustomerSheet.Configuration() // Configure settings for the CustomerSheet here. For example: configuration.headerTextForSelectionScreen = "Manage your payment method" let customerSheet = CustomerSheet(configuration: configuration, customer: customerAdapter)